第一题:分数
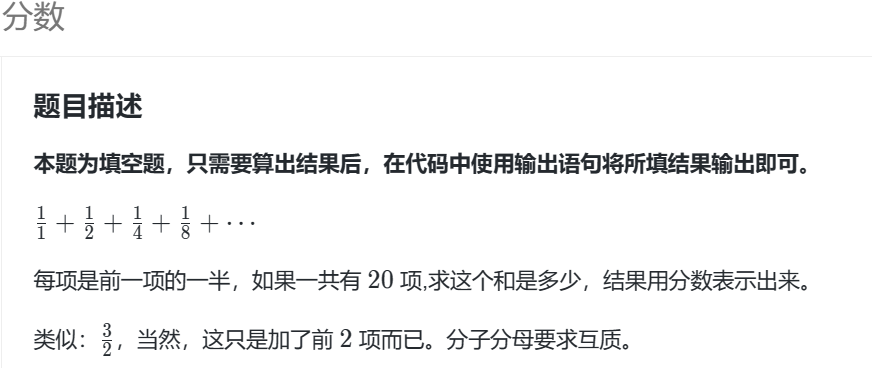
解法: 观察 1/1 1/2 1/4 这三个即可。
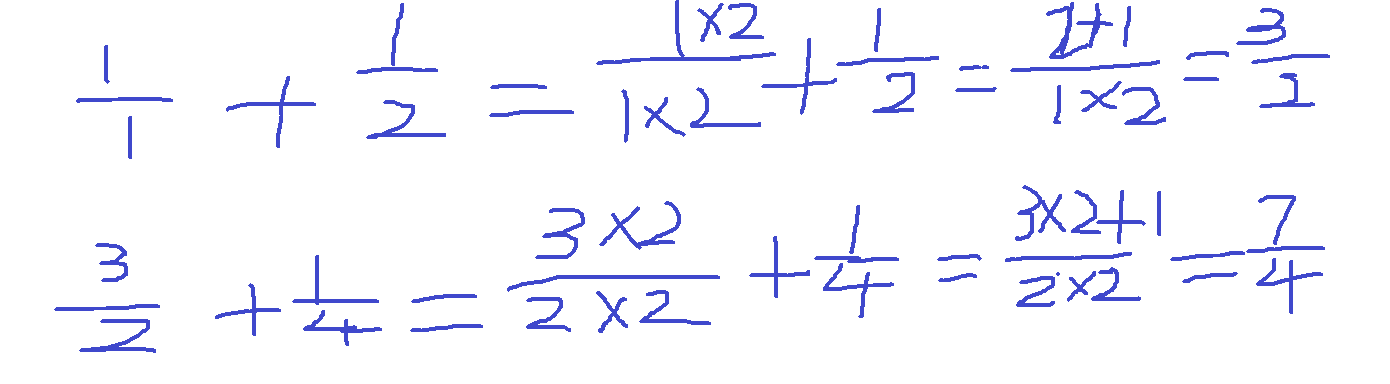
可以发现 一个公式,只要将前一项和的 分子*2+1 / 前一项和的 分母*2 即可,最后将分子分母除去它们的最大公约数。
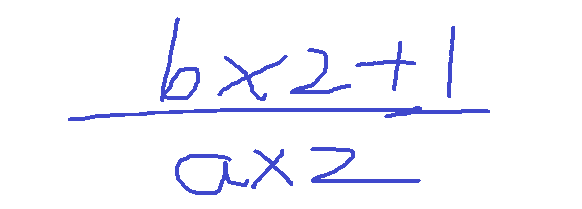
public class Main {
static int gcd(int a, int b) {
return b == 0?a:gcd(b, a%b);
}
public static void main(String[] args) {
int a = 1, b = 1;
for(int i = 2; i <= 20; i++) {
b = b*2 + 1;
a = a * 2;
}
int t = gcd(a, b);
a = a/t;
b = b/t;
System.out.println(b + "/" + a);
// System.out.println("1048575/524288");
}
}
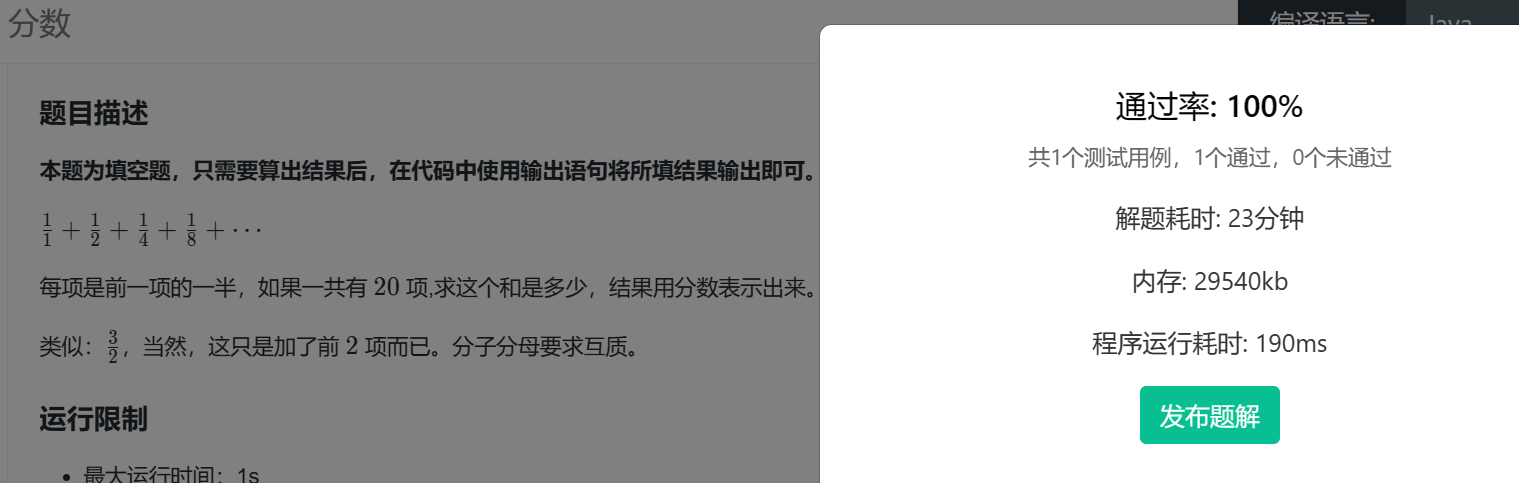
第二题:回文日期
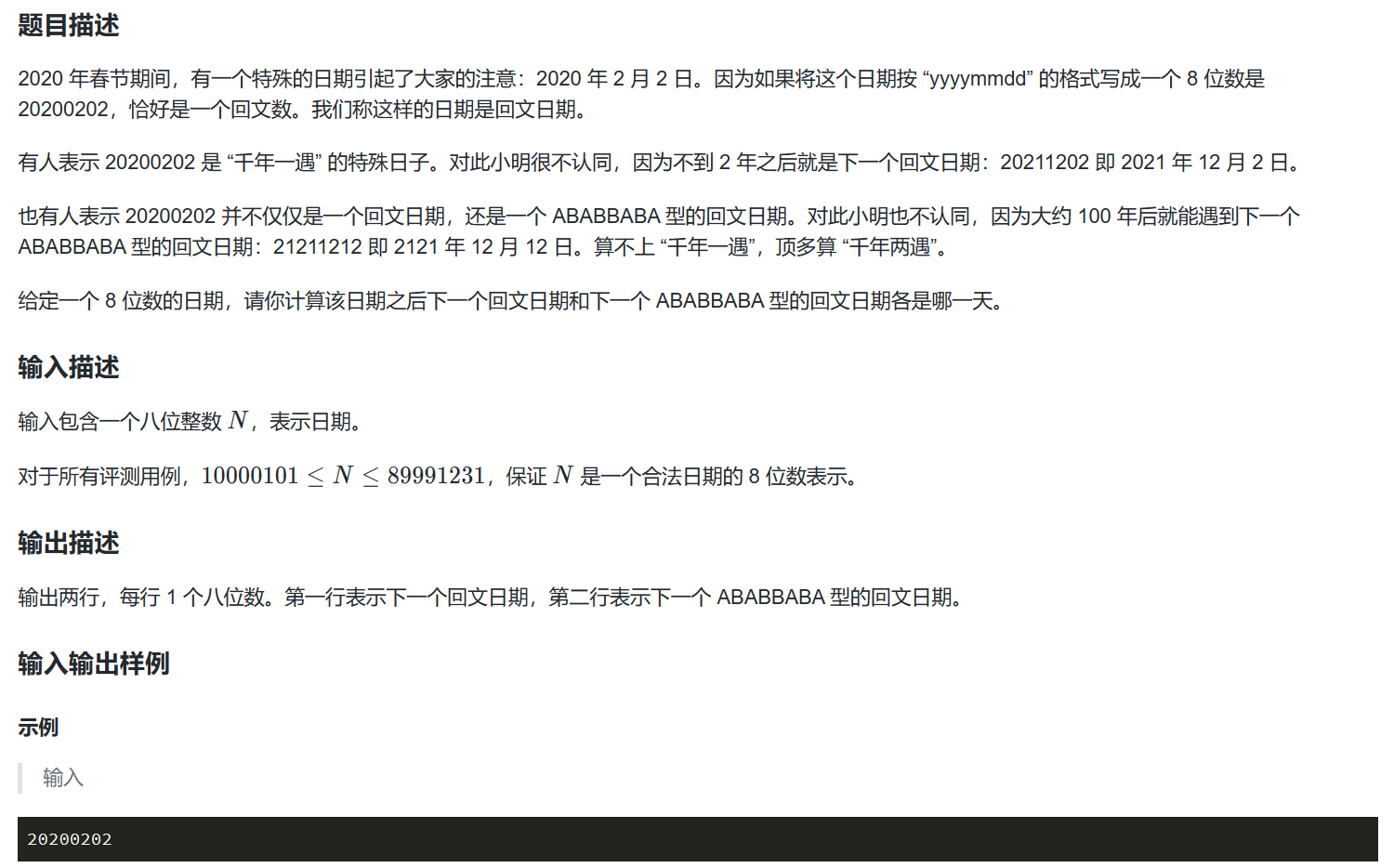

解法:1、 8位数中,取出前4位为year年份。
2、将year分解 为 回文的 month月份 和 day天数。
3、分别判断 month 和 day 是否合法。
4、如果合法则该8位数为回文,且如果month == day则该8位数为 ABABBABA型
5、如果不合法,则year++;
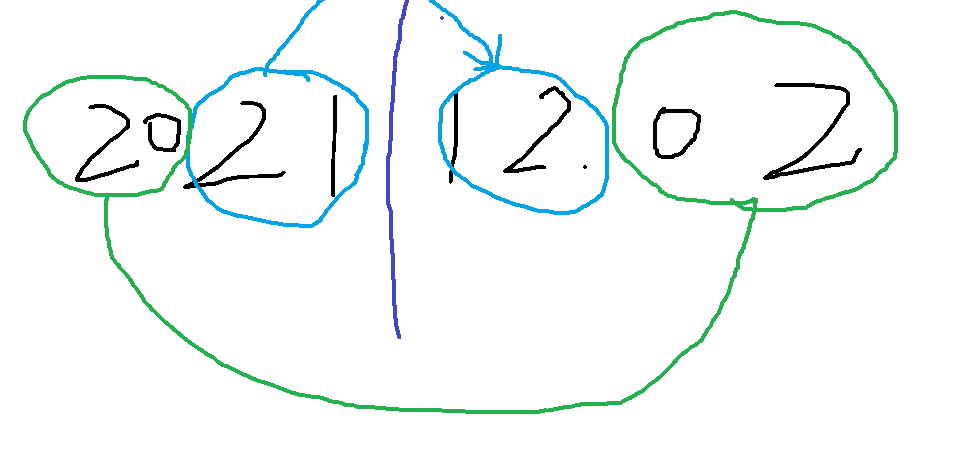
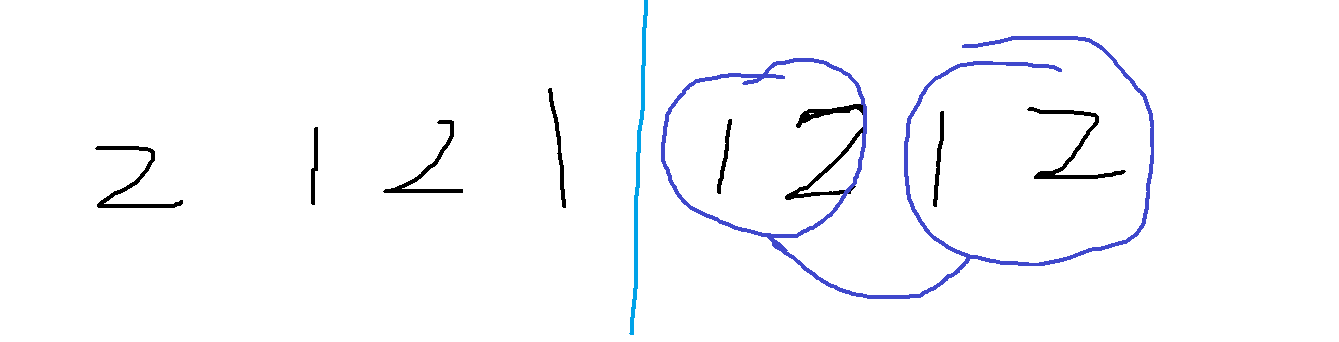
import java.util.Scanner;
public class 回文日期优雅代码 {
static int[] day = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
long n = s.nextLong();
boolean flag = false;
int y = (int) (n / 10000); //year
while(true){
int m = (y % 10) * 10 + (y%100/10); //month
int d = (y / 100 % 10) * 10 + (y / 100 / 10); //day
if(m >= 1 && m <= 12 && d >= 1 && d <= day[m]){ // 是否合法
if(!flag){ // 只打印第一次出现的回文
System.out.printf("%04d%02d%02d", y, m, d);
System.out.println();
flag = true;
}
if(m == d){ // month == day
System.out.printf("%04d%02d%02d", y, m, d);
break;
}
}
y++;
}
}
}
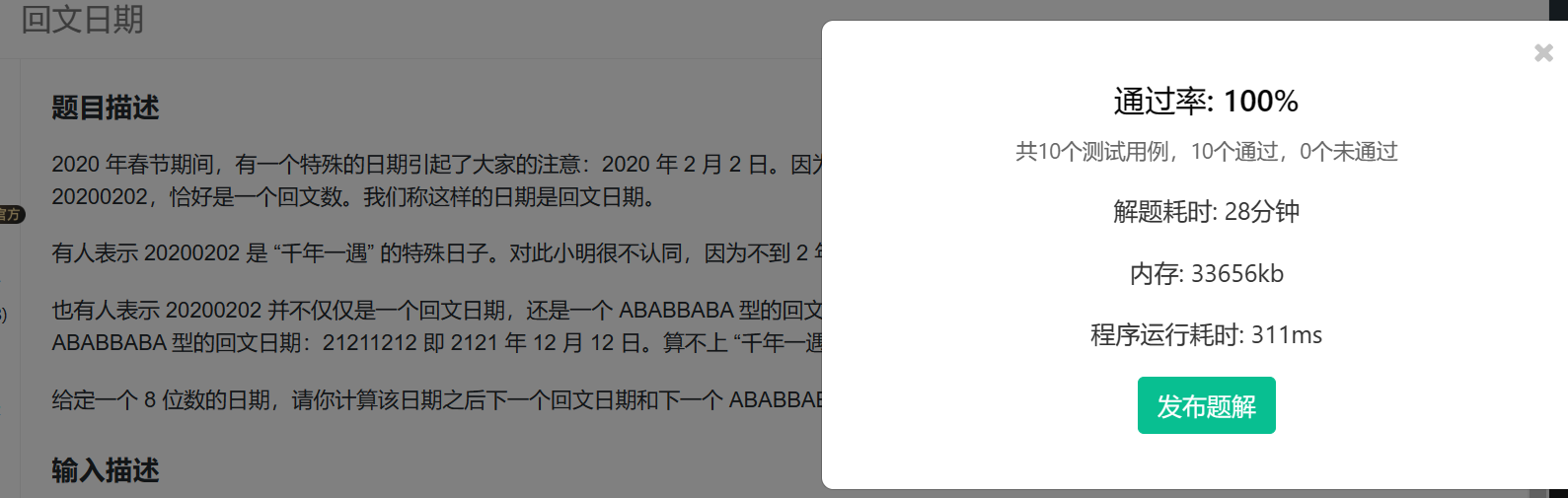
第三题:迷宫
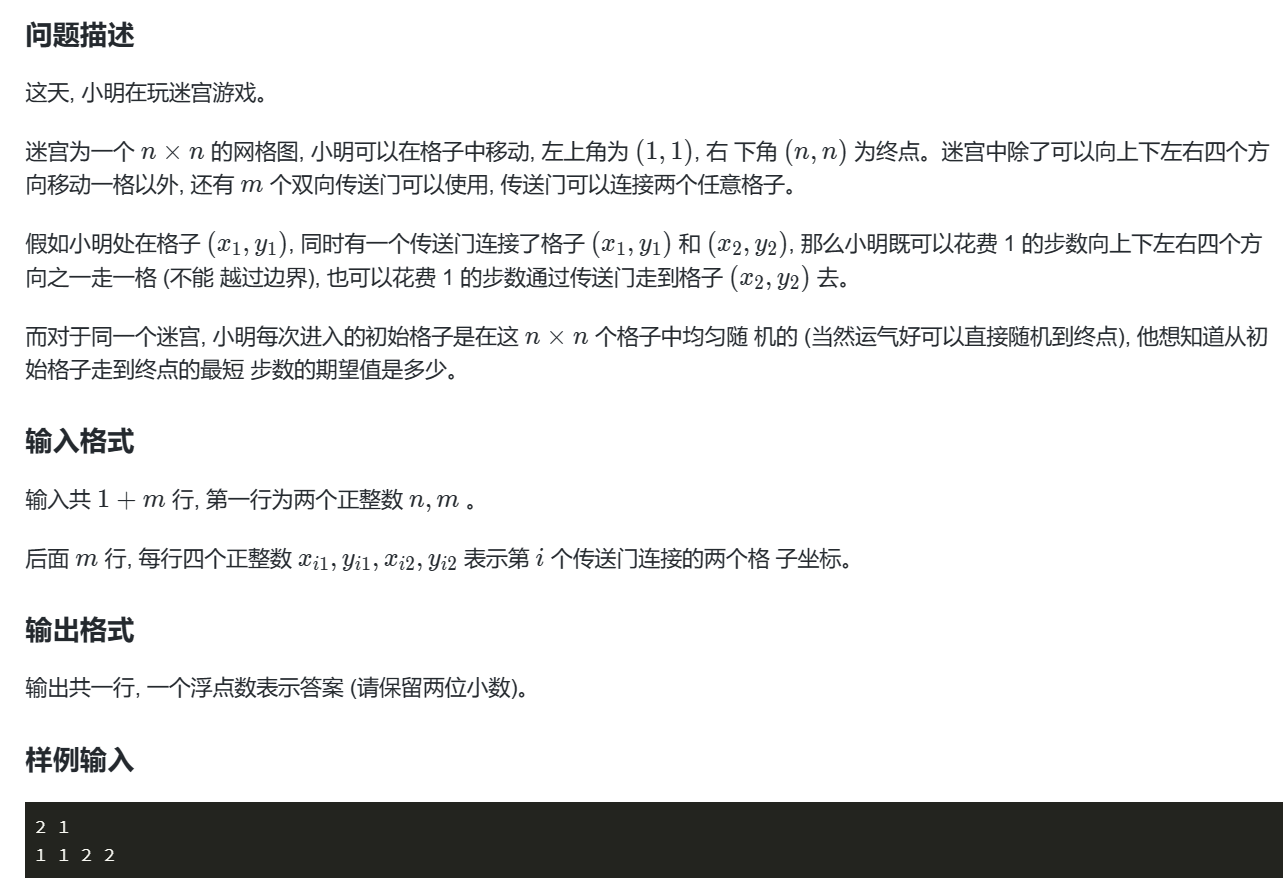
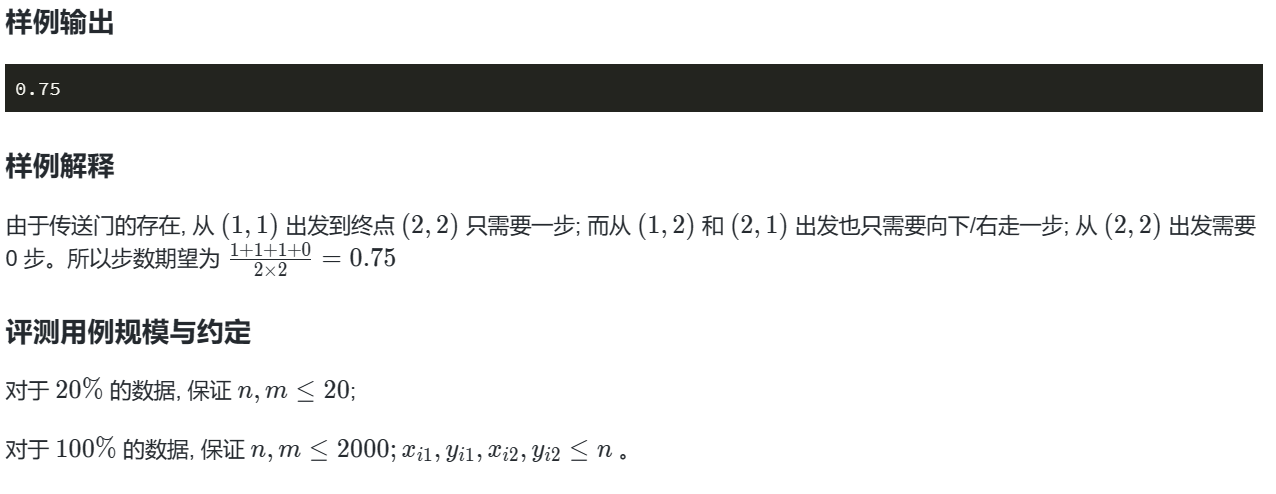
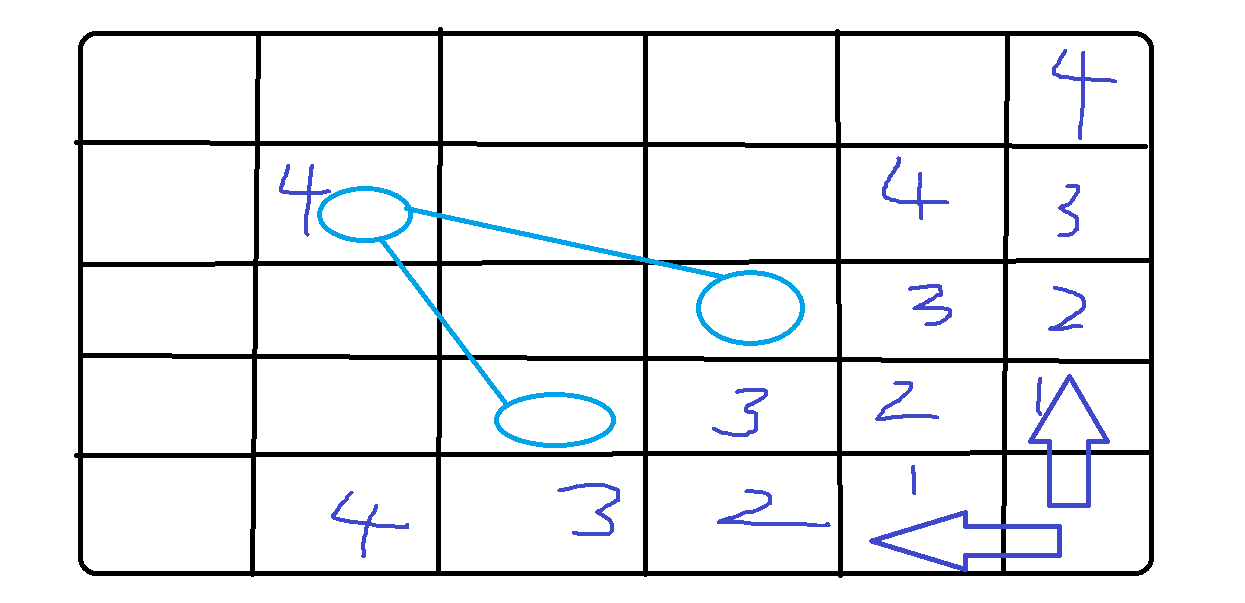
解法:
反向bfs。 将有传送门的两点记录下, 在反向bfs的过程中检查该点是否带有传送门。
且将遍历到的每一个步数加入答案中
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.Map;
import java.util.Queue;
import java.util.Scanner;
public class Main {
static int N = (int)2e3+10;
static boolean[][] st = new boolean[N][N];
static Queue<int[]> q = new LinkedList<>();
static Map<Integer, ArrayList<int[]>> mp = new HashMap<>();
static int[] dx = {0, 1, 0, -1};
static int[] dy = {1, 0, -1, 0};
static int n;
static void add(int x1, int y1, int x2, int y2) {
int key = x1 * n + y1;
if(!mp.containsKey(key)) mp.put(key, new ArrayList<>());
mp.get(key).add(new int[]{x2, y2});
}
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
n = s.nextInt();
int m = s.nextInt();
for(int i = 1; i <= m; i++) {
int x1 = s.nextInt();
int y1 = s.nextInt();
int x2 = s.nextInt();
int y2 = s.nextInt();
add(x1, y1, x2, y2);
add(x2, y2, x1, y1);
}
st[n][n] = true;
q.add(new int[] {n, n});
int res = 0;
int cnt = 0;
while(!q.isEmpty()) {
int size = q.size();
while(size-- > 0) {
res += cnt;
int[] t = q.poll();
int x = t[0], y = t[1];
int key = x * n + y;
if(mp.containsKey(key)) {
ArrayList<int[]> tmpArr = mp.get(key);
for(int[] tAr: tmpArr) {
if(!st[tAr[0]][tAr[1]]) {
q.add(new int[] {tAr[0], tAr[1]});
st[tAr[0]][tAr[1]] = true;
}
}
}
for(int i = 0; i < 4; i++) {
int tX = x - dx[i], tY = y - dy[i];
if(tX >= 1 && tX <= n && tY >= 1 && tY <= n && !st[tX][tY]) {
q.add(new int[] {tX, tY});
st[tX][tY] = true;
}
}
}
cnt++;
}
System.out.printf("%.2f", (res*1.0)/(n*n));
}
}
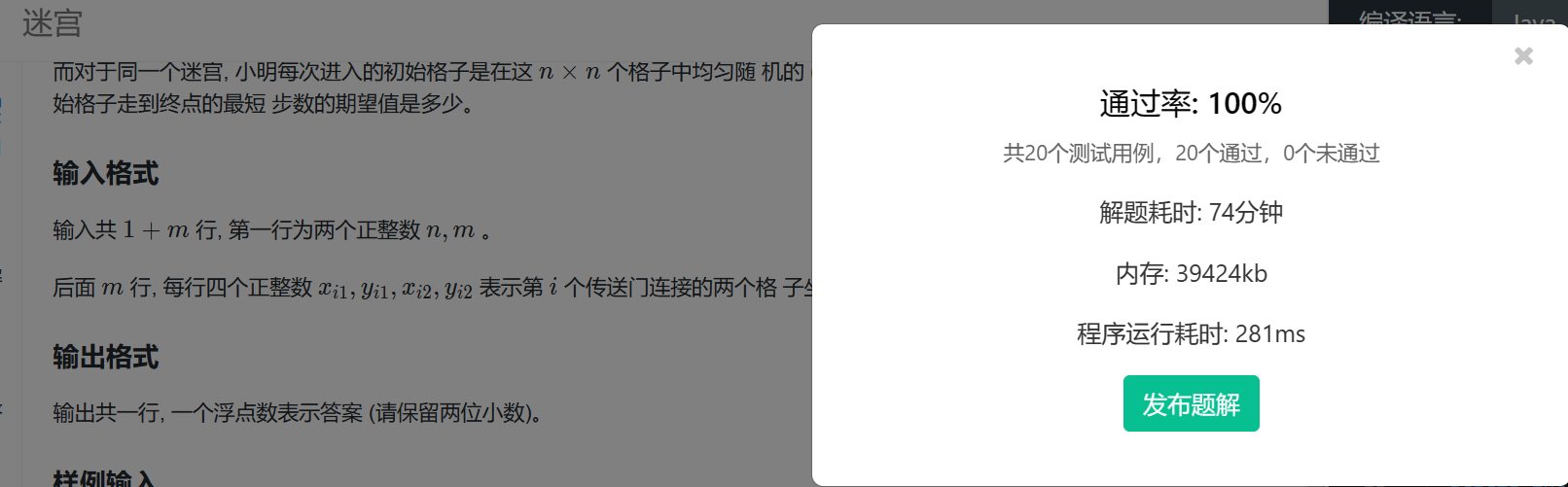
第四题:斐波那契
待我哪天功力大成,放在评论区里。如果我忘记了,有缘人@我
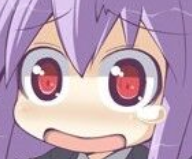