java学习笔记day08
思维导图
工具类及其说明书的制作 + 代码块 + 继承
一、工具类
工具类的说明书生成(IDEA)
如何使用帮助文档API⭐
使用帮助文档的例子
二、代码块⭐
实例
运行结果
三、继承⭐⭐⭐
1.概述
2.继承的好处与弊端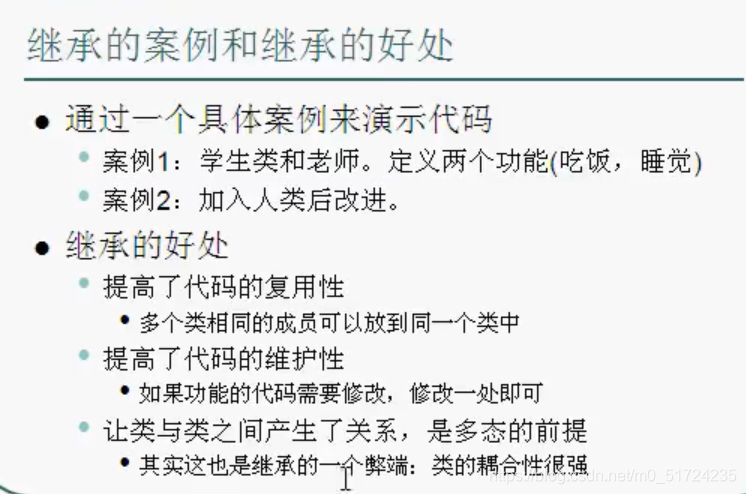
3.继承的特点
4.继承的注意事项
5.继承中成员变量的关系
6.super关键字
super与this
代码实例
7.继承中构造方法的关系⭐【P218】
8.重要的3道面试题⭐⭐
1.【P219】
class Father {
public int num = 10;
public Father() {
System.out.println("Father");
}
}
class Son extends Father{
public int num = 20;
public Son() {
System.out.println("Son");
}
public void show() {
int num = 30;
System.out.println(num);
System.out.println(this.num);
System.out.println(super.num);
}
}
public class stuy {
public static void main(String[] args) {
Son s = new Son();
s.show();
}
}
结果
2. 【P220】
class Father {
static {
System.out.println("Father的静态代码块");
}
{
System.out.println("Father的构造代码块");
}
public Father() {
System.out.println("Father的构造方法");
}
}
class Son extends Father{
static {
System.out.println("Son的静态代码块");
}
{
System.out.println("Son的构造代码块");
}
public Son() {
System.out.println("Son的构造方法");
}
}
public class stuy {
public static void main(String[] args) {
Son s = new Son();
}
}
结果
3.【P221】
class X {
Y b = new Y();
X() {
System.out.print("X");
}
}
class Y {
Y() {
System.out.print("Y");
}
}
public class stuy extends X{
Y y = new Y();
stuy() {
//super();
System.out.println("Z");
}
public static void main(String[] args) {
new stuy();
}
}
结果
9.方法重写⭐⭐
案例
案例源代码
class Phone {
public void call(String name) {
System.out.println("给"+name+"打电话");
}
}
class NewPhone extends Phone {
public void call(String name) {
super.call(name);
//System.out.println("给"+name+"打电话");
System.out.println("可以听天气预报了");
}
}
public class stuy {
public static void main(String[] args) {
NewPhone np = new NewPhone();
np.call("JOJO");
}
}
方法重写注意事项
方法重写的两个案例⭐ 【P227、P228】
1.学生案例
class Person {
private String name;
private int age;
public Person() {
}
public Person(String name,int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
class Student extends Person {
public Student() {
}
public Student(String name,int age) {
//this.name = name;错误写法
//this.age = age;错误写法
super(name,age); //易错点!!!!
}
}
public class stuy {
public static void main(String[] args) {
//方式1
Student s1 = new Student();
s1.setName("JOJO");
s1.setAge(17);
System.out.println(s1.getName()+"---"+s1.getAge());
//方式2
Student s2 = new Student("JOJO",17);
System.out.println(s2.getName()+"---"+s2.getAge());
}
}
2.猫狗案例
/*
猫狗案例讲解
先找到具体的事物,然后发现具体的事物有共性,才提取出一个父类。
猫:
成员变量:姓名,年龄,颜色
构造方法:无参,带参
成员方法:
getXxx()/setXxx()
eat()
palyGame()
狗:
成员变量:姓名,年龄,颜色
构造方法:无参,带参
成员方法:
getXxx()/setXxx()
eat()
lookDoor()
共性:
成员变量:姓名,年龄,颜色
构造方法:无参,带参
成员方法:
getXxx()/setXxx()
eat()
把共性定义到一个类中,这个类的名字叫:动物。
动物类:
成员变量:姓名,年龄,颜色
构造方法:无参,带参
成员方法:
getXxx()/setXxx()
eat()
猫:
构造方法:无参,带参
成员方法:palyGame()
狗:
构造方法:无参,带参
成员方法:lookDoor()
*/
//定义动物类
class Animal {
//姓名
private String name;
//年龄
private int age;
//颜色
private String color;
public Animal() {}
public Animal(String name,int age,String color) {
this.name = name;
this.age = age;
this.color = color;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public void eat() {
System.out.println("不要睡了,该吃饭了");
}
}
//定义猫类
class Cat extends Animal {
public Cat() {}
public Cat(String name,int age,String color) {
super(name,age,color);
}
public void playGame() {
System.out.println("猫玩英雄联盟");
}
}
//定义狗类
class Dog extends Animal {
public Dog() {}
public Dog(String name,int age,String color) {
super(name,age,color);
}
public void lookDoor() {
System.out.println("狗看家");
}
}
//测试类
class stuy {
public static void main(String[] args) {
//测试猫
//方式1
Cat c1 = new Cat();
c1.setName("Tom");
c1.setAge(3);
c1.setColor("白色");
System.out.println("猫的名字是:"+c1.getName()+";年龄是:"+c1.getAge()+";颜色是:"+c1.getColor());
c1.eat();
c1.playGame();
System.out.println("---------------");
//方式2
Cat c2 = new Cat("杰瑞",5,"土豪金");
System.out.println("猫的名字是:"+c2.getName()+";年龄是:"+c2.getAge()+";颜色是:"+c2.getColor());
c2.eat();
c2.playGame();
//作业:测试狗
}
}
特别补充!!!🤡
子类对父类private的继承,应该这样理解:
子类继承了父类的private属性或方法,只是没有办法直接调用而已!