1.选择排序
选择排序分为已排序区和未排序区,选择排序会从未排序数组中寻找最小的数组,将它放在已排序的末尾。
选择排序示意图
代码实现
package DataStruct2.Sort;
//选择排序不稳定,最坏时间复杂度为O(n^2) 最好时间复杂度为O(n^2)
public class selectionSort {
public static void main(String[] args) {
int []a=new int[]{6,4,5,2};
selectionSort(a,4);
for(int b :a){
System.out.print( b+" ");
}
}
// a为数组,n为数组大小
//选择排序
public static void selectSort(int []a,int maxsize){
if (maxsize<=1) return;
for (int i = 0; i <maxsize-1 ; i++) {
int mini=i;
for (int j=i; j<=maxsize-1; j++) {
if (a[j]<a[mini]){
mini=j;
}
}
int temp=a[mini];
a[mini]=a[i];
a[i]=temp;
}
}
}
2.插入排序
冒泡排序分为已排序分区和未排序分区,从未排序分区里获取第一个元素,然后比较已排序分区里的元素然后插入到已排序分区中
代码示意图
代码实现
package DataStruct2.Sort;
//插入排序最好时间复杂度为O(n) 最坏时间复杂度为O(n^2) 稳定
public class insertSort {
public static void main(String[] args) {
int []a=new int[]{3,4,2,1};
insertSort(a,4);
for(int b :a){
System.out.print( b+" ");
}}
public static void insertSort(int a[],int n){
if (n<=1) return;
for (int i = 1; i < n; i++) {
int value=a[i];
int j=i-1;
for (; j >=0 ; j--) {
if (a[j]>value){
a[j+1]=a[j];
}else{
break;
}
}
a[j+1]=value;
}
}
}
3.冒泡排序
冒泡排序是数组从前往后选取最大的元素,放在数组的最后一个位置。
冒泡排序示意图
代码实现
package DataStruct2.Sort;
//冒泡排序最好时间复杂度为O(n) 最坏时间复杂度为O(n^2) 稳定
public class bubbleSort {
public static void main(String[] args) {
int[] a = new int[]{3, 4, 2, 1};
bubbleSort(a, 4);
for (int b : a) {
System.out.print(b + " ");
}
}
public static void bubbleSort(int a[], int n) {
if (n <= 1) return;
boolean flag = false;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (a[j] > a[j + 1]) {
int temp = a[j + 1];
a[j + 1] = a[j];
a[j] = temp;
flag = true;
}
}
if (flag != true) {
break;
}
}
}
}
总结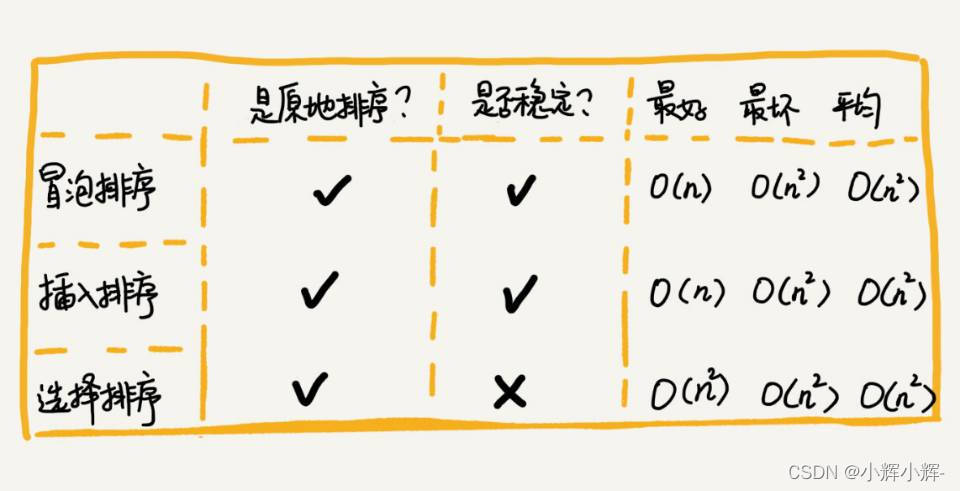
每一个排序都不是简单的排序,都需要思考最好情况和最差情况,然后在不断地完善,是排序能够达到最优解,提高了自己对排序算法的了解。