本文编写了一个简单的TCP通信winform控制台例程:
服务器写法:
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
namespace TCPserver
{
class Program
{
static void Main(string[] args)
{
Socket tcpServer = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
/*固定写法*/
IPAddress ipAddress = new IPAddress(new byte[] { 192, 168, 42, 27 }); //win+R ---cmd --ipconfig--看你的IPv4的IP,因为是字节用逗号分割
IPEndPoint iPEndPoint = new IPEndPoint(ipAddress, 7788); //ip + 端口号 //服务器IP +端口
tcpServer.Bind(iPEndPoint); //绑定
tcpServer.Listen(100); //监听
Console.WriteLine("服务器提示:开始等待客户端连接:");
Socket client = tcpServer.Accept(); //已连接上的客户端对象。 如果没连上 程序会卡在Accept()这里一直等
Console.WriteLine("服务器提示:一个客户端连接过来...");
//接收部分
byte[] data = new byte[1024];
int recive_length = client.Receive(data); //,函数自带返回值,真实收到多少数据长度 会返回给recive_length ,数据存在data里
String recive_mesaagr = Encoding.UTF8.GetString(data);
Console.WriteLine("收到客户端发送消息为:" + recive_mesaagr); //把收到的byte 变成 string
//发送部分
string strSendMessage = "服务器发送的消息:欢迎你!";
client.Send(Encoding.UTF8.GetBytes(strSendMessage)); //规定双方通信用 byte 收到byte再自己解包变成string
client.Close();
tcpServer.Close();
}
}
}
客户端写法:
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
namespace TCPclient
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
Socket tcpClient = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
/*固定写法*/
IPAddress ipAddress = new IPAddress(new byte[] { 192, 168, 42, 27 }); //win+R ---cmd --ipconfig--看你的IPv4的IP,因为是字节用逗号分割
IPEndPoint iPEndPoint = new IPEndPoint(ipAddress, 7788); //ip + 端口号 //客户端IP +端口
Console.WriteLine("客户端提示----开始连接服务器");
tcpClient.Connect(iPEndPoint); //连接
Console.WriteLine("客户端提示----成功连接上服务器..");
//发送部分
string strSendMessage = "客户端发送的消息:你好!来自客服端.....";
tcpClient.Send(Encoding.UTF8.GetBytes(strSendMessage)); //规定双方通信用 byte 收到byte再自己解包变成string
//接收部分
byte[] data = new byte[1024];
int recive_length = tcpClient.Receive(data); //,函数自带返回值,真实收到多少数据长度 会返回给recive_length ,数据存在data里
Console.WriteLine("收到服务器发送消息为:"+ Encoding.UTF8.GetString(data)); //把收到的byte 变成 string
tcpClient.Close(); //用完要关闭
}
}
}
运行结果:
服务器:
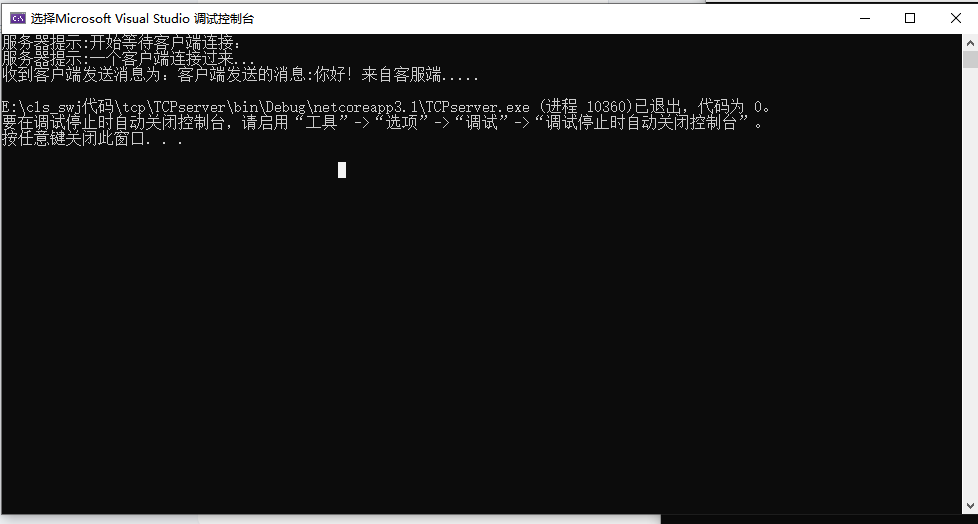
客户端:
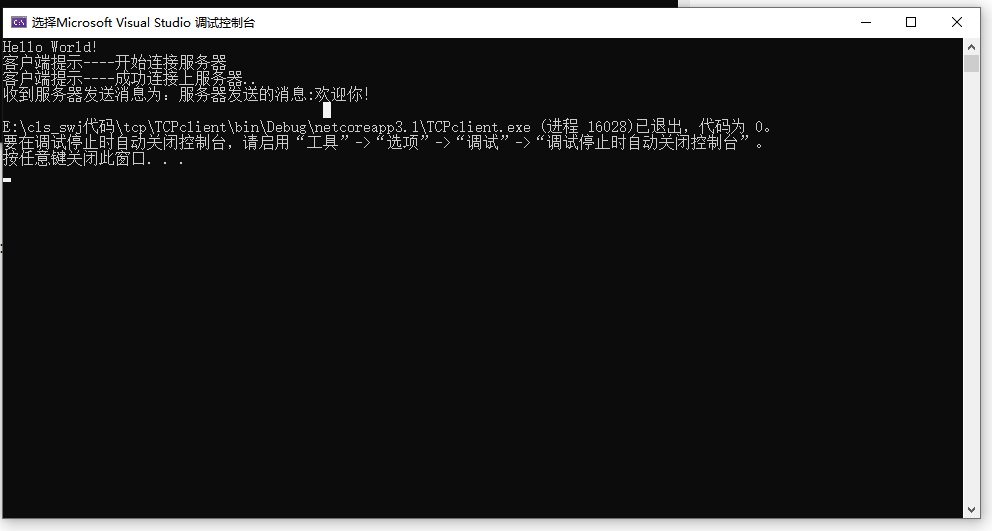
但是这只是 服务器连接一个客户端的写法,要是想用服务器连接多个客户端,还需用到线程,一个线程负责接收,一个线程负责发送....