package socketDemo;
import java.util.concurrent.CountDownLatch;
public class CoachRacerDemo {
private CountDownLatch countDownLatch = new CountDownLatch(3);
public void racer() {
String name = Thread.currentThread().getName();
System.out.println(name+"正在准备。。。");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(name+"准备完毕");
countDownLatch.countDown();
}
public void coach() {
String name = Thread.currentThread().getName();
System.out.println(name+"等待运动员准备。。。");
try {
countDownLatch.await();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("所有运动员已经就绪,"+name+"开始训练");
}
public static void main(String[] args) {
final CoachRacerDemo coachRacerDemo = new CoachRacerDemo();
Thread thread1 = new Thread(new Runnable() {
public void run() {
coachRacerDemo.racer();
}
},"运动员1");
Thread thread2 = new Thread(new Runnable() {
public void run() {
coachRacerDemo.racer();
}
},"运动员2");
Thread thread3 = new Thread(new Runnable() {
public void run() {
coachRacerDemo.racer();
}
},"运动员3");
Thread thread4 = new Thread(new Runnable() {
public void run() {
coachRacerDemo.coach();
}
},"教练");
thread1.start();
thread2.start();
thread3.start();
thread4.start();
}
}
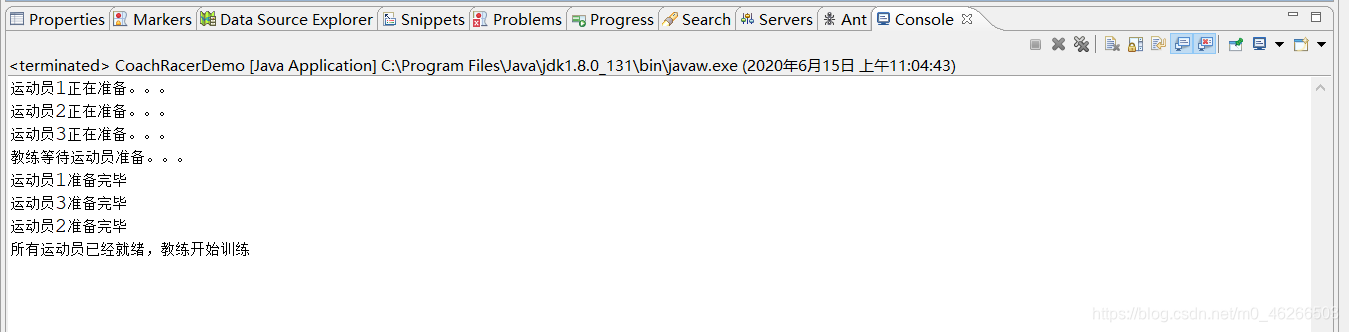