js原型
//定义一个类
class Student {
constructor(name, score) {
this.name = name;
this.score = score;
}
introduce() {
//introduce方法是类上的方法
console.log("xxx");
}
}
let stu = new Student("柴柴", 11); //创建一个实例
stu.introduce()
console.log("stu", stu);
找一个对象的属性或方法时,如果在当前的对象上找不到,就会去它原型上找,如果有这样一个方法就会去调用
stu实例是由Student这个类创建成的
stu.Prototype指向一个对象,这个对象上面有introduce方法
类Student上面也有一个Prototype属性,这个属性也指向一个对象,这个对象上面有introduce方法
stu.Prototype===Student.Prototype指向同一个对象
js原型链
// 子类可以继承父类的属性和方法,extends、super
class Person {
constructor(name) {
this.name = name;
}
drink() {
console.log("person的方法drink");
}
}
class Student extends Person {
constructor(name, score) {
super(name);
this.score = score;
}
introduce() {
console.log(`我是${this.name},考了${this.score}分`);
}
}
let stu = new Student("柴柴", 19);
stu.drink();
console.log(stu);``
当stu上没有drink方法,会往stu的原型去寻找,此时没有的话再往它父类的原型去找,直到找到了或者是没有就返回,这就是原型链
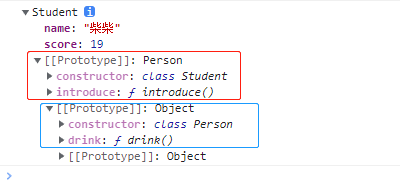
判断某个属性是否在对象上
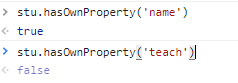