- 纯JavaScript代码实现京东轮播图效果
- 功能包括,点击左右按钮跳转下一张图片,点击小圆圈跳转图片,鼠标移动轮播图上面停止轮播,无缝循环播放,
- 代码注释写在里面咯
CSS样式
* {
margin: 0;
padding: 0;
}
.box {
width: 415px;
height: 241px;
background-color: salmon;
margin: 50px auto;
position: relative;
z-index: 99;
overflow: hidden;
}
.imgList {
position: absolute;
list-style: none;
width: 600%;
left: 0;
top: 0;
}
.imgList li {
float: left;
}
.imgList li img {
width: 415px;
height: 241px;
cursor: pointer;
}
.circle {
position: absolute;
width: 100px;
height: 10px;
z-index: 999;
display: flex;
justify-content: space-around;
align-items: center;
left: 158px;
top: 221px;
}
.circle li {
list-style: none;
width: 10px;
height: 10px;
border-radius: 50%;
cursor: pointer;
background-clip: content-box;
border: 1px solid rgba(255, 255, 255, 0.5);
padding: 1px;
}
.current {
background-color: #fff;
}
.leftBtn {
position: absolute;
width: 30px;
height: 60px;
color: black;
background-color: rgba(255, 255, 255, 0.5);
left: 0;
top: 90px;
z-index: 66;
text-decoration: none;
text-align: center;
line-height: 60px;
display: none;
}
.rightBtn {
position: absolute;
width: 30px;
height: 60px;
color: black;
background-color: rgba(255, 255, 255, 0.5);
right: 0;
top: 90px;
z-index: 66;
text-decoration: none;
text-align: center;
line-height: 60px;
display: none;
}
页面
<div class="box">
<a href="javaScript:;" class="leftBtn">左</a>
<a href="javaScript:;" class="rightBtn">右</a>
<ul class="imgList">
<li><img src="images/suning1.jpg" alt=""></li>
<li><img src="images/suning2.jpg" alt=""></li>
<li><img src="images/suning3.jpg" alt=""></li>
<li><img src="images/suning4.jpg" alt=""></li>
</ul>
<ol class="circle">
</ol>
</div>
JavaScript代码
var leftBtn = document.querySelector('.leftBtn');
var rightBtn = document.querySelector('.rightBtn');
var box = document.querySelector('.box');
var boxWidth = box.offsetWidth;
box.addEventListener('mouseenter', function () {
leftBtn.style.display = 'block';
rightBtn.style.display = 'block';
clearInterval(timer);
timer = null;
})
box.addEventListener('mouseleave', function () {
leftBtn.style.display = 'none';
rightBtn.style.display = 'none';
timer = setInterval(function () {
rightBtn.click();
}, 2000);
})
var ul = box.querySelector('ul');
var ol = box.querySelector('.circle')
for (var i = 0; i < ul.children.length; i++) {
var li = document.createElement('li');
li.setAttribute('index', i);
ol.appendChild(li);
li.addEventListener('click', function () {
for (var i = 0; i < ol.children.length; i++) {
ol.children[i].className = '';
}
this.className = 'current';
var index = this.getAttribute('index');
num = index;
circle = index;
animate(ul, -index * boxWidth)
})
}
ol.children[0].className = 'current';
var first = ul.children[0].cloneNode(true);
ul.appendChild(first);
var num = 0;
var circle = 0;
rightBtn.addEventListener('click', function () {
if (num == ul.children.length - 1) {
ul.style.left = 0;
num = 0;
}
num++;
animate(ul, -num * boxWidth);
circle++;
if (circle == ol.children.length) {
circle = 0;
}
circleChange();
})
leftBtn.addEventListener('click', function () {
if (num == 0) {
num = ul.children.length - 1;
ul.style.left = -num * boxWidth + 'px';
}
num--;
animate(ul, -num * boxWidth);
circle--;
circle = circle < 0 ? ol.children.length - 1 : circle;
circleChange();
});
function circleChange() {
for (var i = 0; i < ol.children.length; i++) {
ol.children[i].className = '';
}
ol.children[circle].className = 'current';
}
var timer = setInterval(function () {
rightBtn.click();
}, 2000);
function animate(obj, target, callback) {
clearInterval(obj.timer);
obj.timer = setInterval(function () {
var step = (target - obj.offsetLeft) / 10;
step = step > 0 ? Math.ceil(step) : Math.floor(step);
if (obj.offsetLeft == target) {
clearInterval(obj.timer);
if (callback) {
callback();
}
}
obj.style.left = obj.offsetLeft + step + 'px';
}, 15)
}
效果
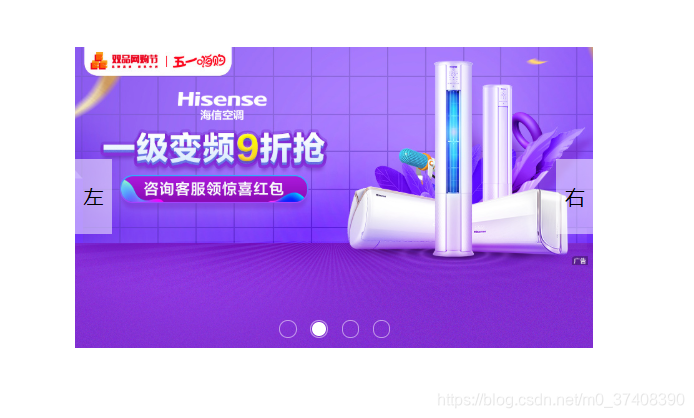