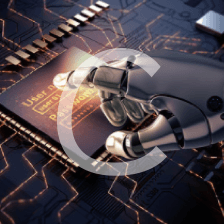
C++学习笔记
宏阳李老师
从事青少年编程教育多年、中国电子学会会员、CSP-GESP—NOI指导教师、蓝桥杯竞赛指导老师、电子协会等考优秀指导教师
展开
-
<3> C++链表的基本实现 2021-05-27
#include <iostream> #include <string> #include <sstream> using namespace std; //链表的实现 和虚拟头节点 template <typename T> class LinkedList { private: class Node { public: T e; Node* next; public: Node():e(NULL),next(NULL){} ..原创 2021-05-27 16:42:52 · 161 阅读 · 0 评论 -
<5> C++链表和递归 2021-05-28
203. 移除链表元素 给你一个链表的头节点 head 和一个整数 val ,请你删除链表中所有满足 Node.val == val 的节点,并返回 新的头节点 。 示例 1: 输入:head = [1,2,6,3,4,5,6], val = 6 输出:[1,2,3,4,5] 示例 2: 输入:head = [], val = 1 输出:[] 示例 3: 输入:head = [7,7,7,7], val = 7 输出:[] 提示: 列表中的节点在范围 [0, 104] 内 1 <= Node.原创 2021-05-28 15:18:34 · 376 阅读 · 0 评论 -
< >C++快速排序法和Select K问题 2021-06-08
#include <iostream> #include <cstdlib> #include <ctime> using namespace std; void swap(int &a,int &b){ int t=a;a=b;b=t; } void print(int arr[],int l){ for(int i=0;i<=l;i++) cout<<arr[i]<<" "; cout<<endl; }.原创 2021-06-08 14:08:24 · 310 阅读 · 0 评论 -
<6> C++基本的二分搜索树 2021-05-25
//基本的二分搜索树 #include <iostream> #include <cstdio> #include <stack> #include <queue> #include <string> #include <sstream> #include <ctime> #include <cstdlib> using namespace std; template<typename T> cl.原创 2021-05-25 18:04:35 · 119 阅读 · 0 评论 -
< >C++归并排序法和逆序对 2021-06-07
//归并排序 #include <iostream> #include <cstdlib> #include <ctime> #include <algorithm> #define N 10000000 using namespace std; int arr[N] = {7, 1, 4, 2, 8, 3, 6, 5}; int tmp[N]; //拷贝arr数组到tmp数组里 void Arr_cpy(int arr[],int length,i.原创 2021-06-07 11:29:39 · 226 阅读 · 0 评论 -
<4> C++纯虚类--测试数据运行速度 2021-05-27
//使用纯虚类实现接口 //使用两种不同底层的数据结构来实现栈的操作 //并测试其数据运行速度。 #include <iostream> #include <vector> #include <list> #include <stdlib.h> #include <ctime> using namespace std; //声明一个纯虚类实现接口 规范了实现类的方法 template <typename T> class My.原创 2021-05-27 20:30:49 · 173 阅读 · 0 评论 -
<2> C++栈和队列的基本实现 2021-05-26
20. 有效的括号 给定一个只包括 '(',')','{','}','[',']' 的字符串 s ,判断字符串是否有效。 有效字符串需满足: 左括号必须用相同类型的右括号闭合。 左括号必须以正确的顺序闭合。 示例 1: 输入:s = "()" 输出:true 示例 2: 输入:s = "()[]{}" 输出:true 示例 3: 输入:s = "(]" 输出:false 示例 4: 输入:s = "([)]" 输出:false 示例 5: 输入:s = "{[]}" 输出:true 示例 6:.原创 2021-05-26 19:55:52 · 177 阅读 · 0 评论 -
<1> C++二次封装动态数组 2021-05-24
//C++二次封装动态数组 #include <iostream> #include <cstdio> #include <cstdlib> #include <string> #include <sstream> using namespace std; template<typename T> class Array { private: T* data; int capacity; int size; public: /.原创 2021-05-24 17:01:13 · 224 阅读 · 0 评论 -
C++数据结构--01数组二次封装成动态数组 2021-04-23
//C++数据结构--01数组二次封装成动态数组 #include <iostream> #include <cstdio> #include <cstdlib> #include <string> #include <sstream> using namespace std; template<typename T> class Array { private: T* data; int capacity; int size;.原创 2021-04-23 13:19:27 · 220 阅读 · 0 评论 -
C++学习笔记_21 优先级队列实现-堆积树-堆排序 2021-05-24
//C++学习笔记_21 优先级队列实现-堆积树-堆排序 #include<vector> #include<iostream> #include<string> #include<deque> #include<queue> using namespace std; class MyPriQue_V0 { private: vector<int> elems; //使用 vector 来存储优先级队列中的数据 pu...原创 2021-05-24 15:55:12 · 271 阅读 · 0 评论 -
C++学习笔记_20 适配器容器-priority_queue 2021-05-19
priority_queue 优先级队列 插入容器内的数据,按降序排列方式被取出 -最先取出的数据,是队列中,值最大的那个 // C++学习笔记_20 适配器容器- priority_queue // #include<iostream> #include<string> #include<queue> using namespace std; class AAA { private: int x; int y; public: AAA().原创 2021-05-19 16:25:12 · 214 阅读 · 0 评论 -
C++学习笔记_19 适配器容器-stack queue 2021-05-19
stack 容器(栈) 只支持在栈顶 存取 元素 后进先出 queue容器(队列) 从容器尾部插入元素,从容器头部取元素 先进先出 // C++学习笔记_19 适配器容器-stack queue #include <iostream> #include<string> #include<vector> #include<list> #include<stack> //STL 中栈的头文件 #include<queue>...原创 2021-05-19 15:21:52 · 229 阅读 · 0 评论 -
C++学习笔记_18 线性容器(vector_list_deque)总结 2021-05-18
vector list deque //C++学习笔记_18 线性容器(vector_list_deque)总结 // #include<iostream> #include<string> #include<vector> #include<deque> #include<list> using namespace std; void TestAll() { vector<int> iVec...原创 2021-05-18 15:16:51 · 276 阅读 · 0 评论 -
C++学习笔记_17 线性容器-Deque容器 2021-05-18
双端队列 deque容器特点 综合了 vector 和 list 的优点 支持下标访问, 也支持两端添加和删除元素 //C++学习笔记_17 线性容器-Deque容器 #include<iostream> #include<string> #include<deque> using namespace std; template <typename T> void PrintDeq(const deque<T> &tDeq) {原创 2021-05-18 14:52:01 · 270 阅读 · 0 评论 -
C++学习笔记_16 线性容器-List容器 2021-05-13
链表(双向) list 不支持对元素的下标访问,在任何位置添加和删除元素,都非常方便。 // C++学习笔记_16 线性容器-List容器 #include<iostream> #include<string> #include<list> #include<vector> using namespace std; template <typename T> void PrintList(const list<T> &a.原创 2021-05-14 13:14:43 · 214 阅读 · 0 评论 -
C++学习笔记_15 线性容器-vector容器 2021-05-12
// C++学习笔记_15 线性容器-vector容器 #include<vector> #include<iostream> #include<string> #include<cstdlib> using namespace std; template <typename T> void PrintVecInfo(const vector<T> &tVec) { cout << "Empty ..原创 2021-05-12 15:13:34 · 433 阅读 · 0 评论 -
C++学习笔记_14 迭代器、与容器无关的算法函数 2021-05-12
// C++学习笔记_14 迭代器、与容器无关的算法函数 #include "stdafx.h" #include<iostream> #include<string> #include"List.h" //这是一个单向链表类 #include"DbList.h" //这是一个双链表类 using namespace std; void TestString() { string ss = "Hello world!"; string::iterator i.原创 2021-05-12 13:03:21 · 253 阅读 · 0 评论 -
C++学习笔记_13 双向链表和链表模板 2021-05-06
// C++学习笔记_13 双向链表和链表模板 #include<cstdio> #include<iostream> using namespace std; class AAA { private: int x; int y; public: AAA() :x(0), y(0) {} AAA(int a, int b) :x(a), y(b){} friend ostream& operator << (ost.原创 2021-05-06 20:25:32 · 174 阅读 · 0 评论 -
C++学习笔记_12 单向链表和单向链表模板 2021-04-29
// C++学习笔记_12 单向链表和单向链表模板 #include<cstdio> #include<iostream> #include<string> using namespace std; //C 语言链表两个问题: //1: 定义链表的时候,我们需要指定链表中存放的数据类型 // 不同数据类型的链表,需要分别实现 //2: LIST *pNode; 语义容易引起误解 // 它既可以代表一个节点,也可以代表一个链表 //--》1:通过模板类来实现。.原创 2021-04-29 11:28:16 · 384 阅读 · 0 评论 -
C++学习笔记_11 模板编程 2021-04-29
// C++学习笔记_11 模板编程 #include<cstdio> #include<iostream> #include<string> using namespace std; /* //交换两个整数变量的值 void swap(int &a, int &b) { int tmp = a;a = b; b = tmp; } //我们想交换两个字符串的值? void swap(string& s1, string& s2) .原创 2021-04-29 10:34:22 · 322 阅读 · 0 评论 -
C++学习笔记_10 异常处理 2021-04-27
// C++学习笔记_10 异常处理 #include <iostream> #include<string> #include<exception> using namespace std; int mydiv(int x, int y) { //if (y == 0) return -1; //return 什么都不合适 if (y == 0) throw "除数为0"; //抛出异常 if (x < y) throw 0;.原创 2021-04-27 14:39:16 · 202 阅读 · 0 评论 -
C++学习笔记_09 IO流 2021-04-26
//C++学习笔记_09 IO流 #include<cstdio> #include<cstring> #include<iomanip> #include<iostream> #include<fstream> #include<sstream> using namespace std; void Test_iostream() { //输出字符串到控制台, 怎么输出的? //把 "Hello world!" 这.原创 2021-04-26 17:06:14 · 218 阅读 · 0 评论 -
C++学习笔记_08 运算符重载 2021-04-22
//C++学习笔记_08 运算符重载 #include<cstdio> #include<iostream> using namespace std; //有理数类 class CRationalNum { private: int bad; //分母为0,这是一个无效的数 bad=1 表示数字无效 int num; //分子 int den; //分母 public: CRationalNum() :num(0), den(1),bad..原创 2021-04-22 14:56:12 · 209 阅读 · 0 评论 -
C++学习笔记_07 const、指针、引用 2021-04-19
//C++学习笔记_07 const、指针、引用_01 #include<iostream> #include<cstring> using namespace std; //编译的步骤:编译预处理 --》 编译 --》 汇编 --》链接 //const 修饰变量,变量值不允许修改 //C++ 派:都用 const 来定义宏变量,替代C语言内的 define const int M = 8; //优点 1 : 有数据类型,代码更加严谨 //优.原创 2021-04-19 17:38:33 · 183 阅读 · 0 评论 -
C++学习笔记_06 深拷贝和浅拷贝 2021-04-19
//C++学习笔记_06 深拷贝和浅拷贝 #include<cstdio> #include<cstring> #include<string> #include<iostream> using namespace std; void TestString() { char szName[12]; char *pName; //szName 的值不能改变,它的值,是栈内存的地址 //szName = "Jack"; //.原创 2021-04-19 15:53:02 · 193 阅读 · 0 评论 -
C++学习笔记_05 string 2021-04-16
//C++学习笔记_05 string #include<cstring> #include<string> #include<iostream> using namespace std; void TestChar() { //我们如果要定义一个字符串 char str[]="abcd"; //等价于 {'a','b','c','d','\0'}; char cArr[]={'a','b','c','d'}; char *pStr.原创 2021-04-16 17:21:35 · 157 阅读 · 0 评论 -
C++学习笔记_04抽象类、多态 2021-04-15
//C++学习笔记_04抽象类、多态 (多重继承的歧义性问题 和 virtual虚继承) #include<cstring> #include<cstdio> #include<iostream> using namespace std; class CBase { public: //我们的类,一定至少要有一个构造函数 //如果不定义构造函数,系统会自动生成一个空的构造函数(构造函数内不做任何事情) //同样的,如果不定义析构函数,系统会.原创 2021-04-15 20:00:54 · 219 阅读 · 0 评论 -
C++学习笔记_03类的继承 2021-04-15
//C++学习笔记_03类的继承 友元类 #include<cstring> #include<iostream> #define C_MAX 10 using namespace std; //如果 课程这个类,只有学生才有,不能说单独定义一个课程对象 class CCourse { friend class CStudent; private: char cname[C_MAX][16]; //课程名称(多个课程,最多 C_MAX 门课程) ...原创 2021-04-15 16:18:46 · 235 阅读 · 0 评论 -
C++学习笔记_02类和对象 2021-04-15
//C++学习笔记_02类和对象 #include<iostream> #include<cstdio> #include<cstring> using namespace std; //类和结构体很类似。都用于定义一个数据结构 //类中定义变量(函数)都默认是private //结构体中 默认是public class CAnimal { friend void TestFriend(); //授权 TestFriend 这个函数,访.原创 2021-04-15 09:16:53 · 434 阅读 · 0 评论 -
C++学习笔记_01基础 2021-04-15
//C++学习笔记_01基础 #include <iostream> #include <stdio.h> #include <cstring> #include <time.h> using namespace std;//命名空间::默认使用这个命名空间中的函数 void Print();//函数声明 void TestBitOper()//位运算 { int a=123; int b=456; int c=a&.原创 2021-04-15 09:14:31 · 236 阅读 · 1 评论