文件上传
代码
提交页面
<%@ taglib uri="/struts-tags" prefix="s"%>
.....
<s:form action="Pic_upload" enctype="multipart/form-data" method="post">
<s:file name="pb.pic" />
<s:submit value="ok"/>
</s:form>
实体类
package com.bean;
import java.io.File;
public class PicBean {
private File pic;
private String picContentType;
private String picFileName;
public PicBean() {
super();
}
public File getPic() {
return pic;
}
public void setPic(File pic) {
this.pic = pic;
}
public String getPicContentType() {
return picContentType;
}
public void setPicContentType(String picContentType) {
this.picContentType = picContentType;
}
public String getPicFileName() {
return picFileName;
}
public void setPicFileName(String picFileName) {
this.picFileName = picFileName;
}
}
Action类
package com.action;
import java.io.File;
import java.io.IOException;
import javax.servlet.http.HttpSession;
import org.apache.commons.io.FileUtils;
import org.apache.struts2.ServletActionContext;
import com.bean.PicBean;
public class PicAction {
private PicBean pb;
private String path;
public PicBean getPb() {
return pb;
}
public void setPb(PicBean pb) {
this.pb = pb;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public String upload() {
System.out.println("文件名称" + pb.getPicFileName());
System.out.println("文件类型" + pb.getPicContentType());
String saveFilePathString = ServletActionContext.getServletContext().getRealPath("/") + "/image/";
File saveFile = new File(saveFilePathString);
if (!saveFile.exists()) {
saveFile.mkdir();
}
String savePicString = saveFilePathString + pb.getPicFileName();
File savePic = new File(savePicString);
try {
FileUtils.copyFile(pb.getPic(), savePic);
} catch (IOException e) {
e.printStackTrace();
}
HttpSession session = ServletActionContext.getRequest().getSession();
session.setAttribute("image",
ServletActionContext.getRequest().getContextPath() + "/image/" + pb.getPicFileName());
path = "show.jsp";
return "ok";
}
}
struts.xml设置
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<constant name="struts.custom.i18n.resources" value="mess"/>
<constant name="struts.ui.theme" value="simple"></constant>
<package name="upload" extends="struts-default" namespace="/">
<action name="Pic_upload" class="com.action.PicAction" method="upload">
<interceptor-ref name="fileUpload">
<param name="allowedTypes">image/png,image/jpg,image/jpeg,image/gif</param>
<param name="maximumSize">2000000</param>
</interceptor-ref>
<interceptor-ref name="defaultStack"/>
<result name="ok">show.jsp</result>
</action>
</package>
</struts>
展示页面
展示:
<img src="${image}"><br>
实际操作
提交
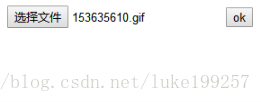
展示
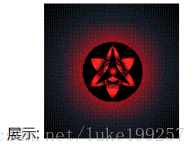