在google地图上定位一个地点,然后点击弹出一个popView显示一下详细信息
这个popView 随便整的,没有美化练着玩的
这个popView 随便整的,没有美化练着玩的
Android专业开发群1:150086842
Android专业开发群2:219277004
标签: <无>
代码片段(5)
[图片] 未命名3.jpg
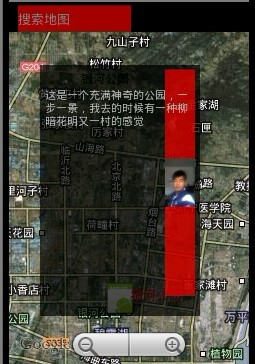
[图片] 未命名4.jpg
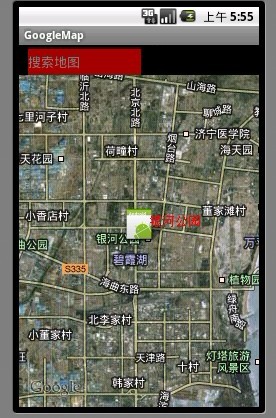
[代码] 新建一个图层
01 | package com.android.angking.yibai; |
02 |
03 | import java.util.ArrayList; |
04 |
05 | import android.content.Context; |
06 | import android.graphics.Canvas; |
07 | import android.graphics.Color; |
08 | import android.graphics.Paint; |
09 | import android.graphics.Point; |
10 | import android.graphics.drawable.Drawable; |
11 |
12 | import com.google.android.maps.ItemizedOverlay; |
13 | import com.google.android.maps.MapView; |
14 | import com.google.android.maps.OverlayItem; |
15 | import com.google.android.maps.Projection; |
16 |
17 | public class CustomItemizedOverlay extends ItemizedOverlay<OverlayItem>{ |
18 | private ArrayList<OverlayItem> mOverlays = new ArrayList<OverlayItem>(); |
19 | private Context context; |
20 | public CustomItemizedOverlay(Context context,Drawable defaultMarker){ |
21 | super (boundCenterBottom(defaultMarker)); |
22 | this .context = context; |
23 | } |
24 | public void draw(Canvas canvas,MapView mapView, boolean shadow){ |
25 | super .draw(canvas, mapView, shadow); |
26 | //Projection 接口用于屏幕像素点坐标体系和地球概况纬度点坐标体系之间的变换 |
27 | Projection projection = mapView.getProjection(); |
28 | //遍历所有的OverlayItem |
29 | for ( int index = this .size()- 1 ;index>= 0 ;index--){ |
30 | //获得给定索引的item |
31 | OverlayItem overlayItem = getItem(index); |
32 | //把经纬度变换相对于MapView左上角的屏幕像素坐标 |
33 | Point point = projection.toPixels(overlayItem.getPoint(), null ); |
34 | |
35 | Paint paintText = new Paint(); |
36 | paintText.setColor(Color.RED); |
37 | paintText.setTextSize( 13 ); |
38 | //绘制文本 |
39 | canvas.drawText(overlayItem.getTitle(), point.x+ 10 , point.y- 15 , paintText); |
40 | } |
41 | } |
42 | protected boolean onTap( int index){ |
43 | setFocus(mOverlays.get(index)); |
44 | return super .onTap(index); |
45 | |
46 | } |
47 | public void removeAll(){ |
48 | if (mOverlays.size()>= 0 ){ |
49 | mOverlays.removeAll(mOverlays); |
50 | } |
51 | } |
52 | public void addOverlay(OverlayItem overlay){ |
53 | mOverlays.add(overlay); |
54 | populate(); |
55 | } |
56 | // public CustomItemizedOverlay(Drawable defaultMarker) { |
57 | // super(boundCenterBottom(defaultMarker)); |
58 | // // TODO Auto-generated constructor stub |
59 | // } |
60 |
61 | @Override |
62 | protected OverlayItem createItem( int i) { |
63 | // TODO Auto-generated method stub |
64 | return mOverlays.get(i); |
65 | } |
66 |
67 | @Override |
68 | public int size() { |
69 | // TODO Auto-generated method stub |
70 | return mOverlays.size(); |
71 | } |
72 | |
73 |
74 | } |
[代码] 自定义的OverlayItem
01 | package com.android.yibai.antking; |
02 |
03 | import android.graphics.Bitmap; |
04 |
05 | import com.google.android.maps.GeoPoint; |
06 | import com.google.android.maps.OverlayItem; |
07 |
08 | public class MyOverlayItem extends OverlayItem{ |
09 | private Bitmap bitmap; |
10 | public MyOverlayItem(GeoPoint point, String title, String snippet,Bitmap bitmap) { |
11 | super (point, title, snippet); |
12 | this .bitmap = bitmap; |
13 | // TODO Auto-generated constructor stub |
14 | } |
15 | public Bitmap getBitmap(){ |
16 | return bitmap; |
17 | } |
18 |
19 | } |
[代码] 主类
001 | package com.android.yibai.antking; |
002 |
003 | import java.util.List; |
004 |
005 | import android.graphics.Bitmap; |
006 | import android.graphics.BitmapFactory; |
007 | import android.graphics.drawable.Drawable; |
008 | import android.os.Bundle; |
009 | import android.view.View; |
010 | import android.widget.ImageView; |
011 | import android.widget.TextView; |
012 |
013 | import com.google.android.maps.GeoPoint; |
014 | import com.google.android.maps.ItemizedOverlay; |
015 | import com.google.android.maps.MapActivity; |
016 | import com.google.android.maps.MapView; |
017 | import com.google.android.maps.Overlay; |
018 | import com.google.android.maps.OverlayItem; |
019 |
020 | public class MapMain extends MapActivity{ |
021 | /** |
022 | * 地图 |
023 | */ |
024 | protected MapView mapView; |
025 | /** |
026 | * 弹出的气泡View |
027 | */ |
028 | private View popView; |
029 | |
030 | private int [] image={R.drawable.icon}; |
031 | |
032 | public void onCreate(Bundle savedInstanceState) { |
033 | super .onCreate(savedInstanceState); |
034 | |
035 | //初始化气泡,并设置为不可见 |
036 | popView = View.inflate( this , R.layout.popview, null ); |
037 | setContentView(R.layout.main); |
038 | |
039 | //获得map |
040 | mapView = (MapView) this .findViewById(R.id.mapview); |
041 | mapView.addView(popView, new MapView.LayoutParams( |
042 | MapView.LayoutParams.WRAP_CONTENT, |
043 | MapView.LayoutParams.WRAP_CONTENT, null |
044 | ,MapView.LayoutParams.BOTTOM_CENTER)); |
045 | //这里没有给GeoPoint ,在onFoucusChangeListener中设置 |
046 | popView.setVisibility(View.GONE); |
047 | /** |
048 | * 创建图标资料(用于显示在overlayItem所表示表记的地位 |
049 | */ |
050 | Drawable drawable = this .getResources().getDrawable(R.drawable.icon); |
051 | |
052 | //为mark定以地位和鸿沟 |
053 | drawable.setBounds( 0 , 0 ,drawable.getIntrinsicWidth(), |
054 | drawable.getIntrinsicHeight()); |
055 | CustomItemizedOverlay overlay = new CustomItemizedOverlay( this ,drawable); |
056 | //设置显示/隐藏气泡的位置 |
057 | overlay.setOnFocusChangeListener(onFocusChangeListener); |
058 | |
059 | /** |
060 | * 创建并添加一个标志 |
061 | */ |
062 | GeoPoint point = new GeoPoint( 35422006 , 119524095 ); |
063 | //创建标识 |
064 | Bitmap bitmap = BitmapFactory.decodeResource(MapMain. this .getResources(), R.drawable.psu); |
065 | MyOverlayItem overlayItem = new MyOverlayItem(point |
066 | , "银河公园" , "这是一个充满神奇的公园,一步一景,我去的时候有一种柳暗花明又一村的感觉" ,bitmap); |
067 | overlay.addOverlay(overlayItem); |
068 | /** |
069 | * 创建第二个标识 |
070 | */ |
071 | Bitmap bitmap1 = BitmapFactory.decodeResource( this .getResources(), R.drawable.icon); |
072 | GeoPoint point1 = new GeoPoint(( int )( 22.53108 *1E6),( int )( 113.99151 *1E6)); |
073 | MyOverlayItem overlayItem1 = new MyOverlayItem(point1, "秀丽中华" , "中国最好的旅游胜地之一" ,bitmap1); |
074 | overlay.addOverlay(overlayItem1); |
075 | |
076 | //向地图里添加自定义的ItemizedOverlay |
077 | List<Overlay> mapOverlays =mapView.getOverlays(); |
078 | mapOverlays.add(overlay); |
079 | //设置地图为卫星地图 |
080 | mapView.setSatellite( true ); |
081 | //设置地图可以缩放 |
082 | mapView.setBuiltInZoomControls( true ); |
083 | /** |
084 | * 取得地图管理对象,用于把握地图 |
085 | * |
086 | */ |
087 | //设置地图的中间 |
088 | mapView.getController().setCenter(point); |
089 | //设置地图默认的缩放级别 |
090 | mapView.getController().setZoom( 13 ); |
091 | |
092 | } |
093 | @Override |
094 | protected boolean isRouteDisplayed() { |
095 | // TODO Auto-generated method stub |
096 | return false ; |
097 | } |
098 | private final ItemizedOverlay.OnFocusChangeListener onFocusChangeListener = new ItemizedOverlay.OnFocusChangeListener() { |
099 |
100 | @Override |
101 | public void onFocusChanged(ItemizedOverlay overlay, OverlayItem newFocus) { |
102 | // TODO Auto-generated method stub |
103 | //创建气泡窗口 |
104 | if (popView!= null ){ |
105 | popView.setVisibility(View.GONE); |
106 | } |
107 | if (newFocus != null ){ |
108 | MapView.LayoutParams geoLp =(MapView.LayoutParams) popView |
109 | .getLayoutParams(); |
110 | geoLp.point = newFocus.getPoint(); //这行用于popView的定位 |
111 | TextView title = (TextView)popView.findViewById(R.id.map_bubbleTitle); |
112 | title.setText(newFocus.getTitle()); |
113 | |
114 | TextView desc = (TextView)popView.findViewById(R.id.map_bubbleText); |
115 | ImageView image = (ImageView)popView.findViewById(R.id.map_bubbleImage); |
116 | image.setImageBitmap(((MyOverlayItem) newFocus).getBitmap()); |
117 | if (newFocus.getSnippet()== null |
118 | ||newFocus.getSnippet().length()== 0 ){ |
119 | desc.setVisibility(View.GONE); |
120 | } else { |
121 | desc.setVisibility(View.VISIBLE); |
122 | desc.setText(newFocus.getSnippet()); |
123 | } |
124 | mapView.updateViewLayout(popView,geoLp); |
125 | popView.setVisibility(View.VISIBLE); |
126 | } |
127 | } |
128 | |
129 | |
130 | }; |
131 |
132 | } |