容器简介
c++常用的容器为vector,其是顺序容器。它将单一类型元素聚集起来成为容器,然后根据位置来存储和访问这些元素,这就是顺序容器。顺序容器的元素排列次序与元素值无关,而是由元素添加到容器里的次序决定。容器类共享公共的接口,这使标准库更容易学习,只要学会其中一种类型就能运用另一种类型。
容器的使用
头文件
#include <vector>
#include <list>
#include <deque>
容器的创建
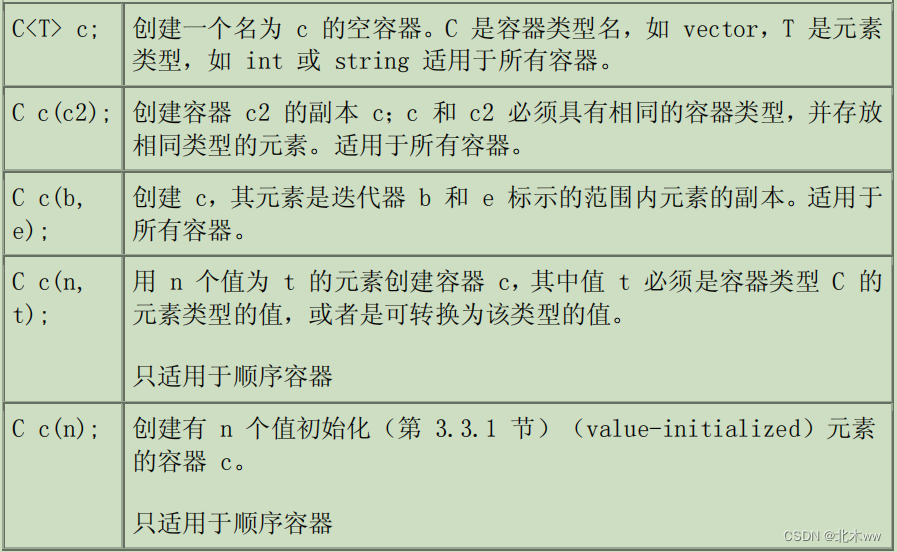
容器副本的创建
当不使用默认构造函数,而是用其他构造函数初始化顺序容器时,必须指出该容器有多少个元素,并提供这些元素的初值。同时指定元素个数和初值的一个方法是将新创建的容器初始化为一个同类型的已存在容器的副本:
vector<int> ivec;
vector<int> ivec2(ivec); // ok: ivec is vector<int>
list<int> ilist(ivec); // error: ivec is not list<int>
vector<double> dvec(ivec); // error: ivec holds int not double
容器的初始化
创建容器时, 我们可以去直接指定容器的大小和容器中任意一个元素来初始化。容器的大小可以是常量,也可以是非常量的表达式。
const list<int>::size_type list_size = 64;
list<string> slist(list_size, "eh?"); // 64 strings, each is eh?
这段代码表示 slist 含有 64 个元素,每个元素都被初始化为“eh?”字符串。
容器大小
容器内元素类型的约束
元素类型
容器的容器
定义 vector 类型的容器 lines,其元素为 string 类型的 vector 对象:
vector< vector<string> > lines;
注意:两个大于号之间有空格,必须这样使用。
迭代器
迭代器的使用
常用迭代器运算
除上诉外,vector容器还支持以下运算
计算vector容器的中心位置:
vector<int>::iterator iter = vec.begin() + vec.size()/2;
容器定义的类型别名
容器的begin和end操作
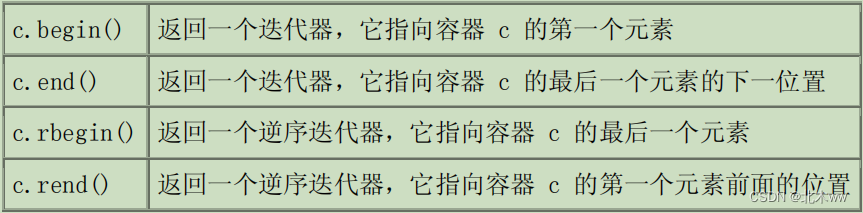
元素的插入
string text_word;
while (cin >> text_word)
container.push_back(text_word);
上面的代码,循环读取string类型的常量,通过使用push_back函数添加到容器container的末尾。
其他添加元素的操作:
实例:
将一段元素添加到容器中:
test.insert(test.end(),10,"Anna");
上面的代码吧10个string类型的常量Anna,添加到test容器的末尾。
将数组的全部或一部分添加到容器中:
string arr[4] = { "1","2","3","4"};
//将数组全部添加进去
test.insert(test.end(),arr,arr+4);
//吧数组的第3个和第4个元素添加进去
test.insert(test.end(),arr+2,arr+4);
元素的删除
指定或部分删除
while (!ilist.empty()) {
process(ilist.front()); // do something with the current top of ilist
ilist.pop_front(); // done; remove first element
}
这个循环非常简单:使用 front 操作获取要处理的元素,然后调用pop_front 函数从容器 list 中删除该元素。pop_front 和 pop_back 函数的返回值并不是删除的元素值, 而是 void。要获取删除的元素值,则必须在删除元素之前调用notfront 或 back 函数。
删除一个或一段元素更通用的方法是 erase 操作。该操作有两个版本:删除由一个迭代器指向的单个元素,或删除由一对迭代器标记的一段元素。erase的这两种形式都返回一个迭代器,它指向被删除元素或元素段后面的元素。也就是说,如果元素 j 恰好紧跟在元素 i 后面,则将元素 i 从容器中删除后,删除操作返回指向 j 的迭代器。
全部删除
slist.clear(); // delete all the elements within the container
slist.erase(slist.begin(), slist.end()); // equivalent
不要储存end操作返回的迭代器
vector<int>::iterator first = v.begin(),
last = v.end();
while (first != last) {
first = v.insert(first, 42);
++first; // advance first just past the element we added
}
while (first != v.end()) {
// do some processing
first = v.insert(first, 42); // insert new value
++first; // advance first just past the element we added
}
赋值和swap
c1 = c2; // replace contents of c1 with a copy of elements in c2
c1.erase(c1.begin(), c1.end()); // delete all elements in c1
c1.insert(c1.begin(), c2.begin(), c2.end()); // insert c2
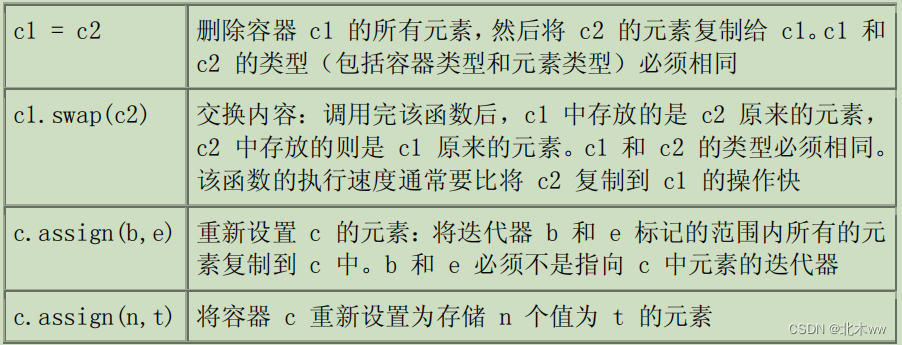
vector<string> svec1(10); // vector with 10 elements
vector<string> svec2(24); // vector with 24 elements
svec1.swap(svec2);