通过 Naive-UI 的数据表格组件为例,实现表格动态显示、隐藏列字段:
<template>
<n-button @click="filterModal = true">
<template #icon>
<n-icon>
<ClearAllOutlined />
</n-icon>
</template>
筛选
</n-button>
<n-modal title="表格字段筛选" v-model:show="filterModal" preset="card" style="width: 600px;">
<n-data-table v-model:checked-row-keys="zdCheckedRowKeys" :single-line="false" :columns="zdColumns"
:data="zdData" :row-key="zdRowKey" :max-height="500" @update:checked-row-keys="filterCol" />
</n-modal>
<n-data-table v-model:checked-row-keys="checkedRowKeys" :single-line="false" :columns="columns"
:data="data" :row-key="rowKey" :loading="loading" :scroll-x="scrollX" :max-height="500" />
</template>
<script lang="ts" setup>
import { ref, onMounted } from "vue";
import { ClearAllOutlined } from '@vicons/material'
const loading = ref(false)
const scrollX = ref(0)
const checkedRowKeys = ref<Array<any>>([])
const rowKey = (row: any) => row
const data= ref<Array<any>>([])
const recordColumns: any = [
{ type: 'selection', title: '复选框', key: 'checkbox', width: 60 },
{ title: '苹果', key: 'apple', width: 100 },
{ title: '芒果', key: 'mango', width: 100 },
{ title: '火龙果', key: 'pitaya', width: 100 },
{ title: '西瓜', key: 'watermelon', width: 100 },
{ title: '禁用列', key: 'disabled', width: 100 },
]
let columns: any = recordColumns.slice()
const filterModal = ref(false)
const zdCheckedRowKeys = ref<Array<any>>([])
const zdRowKey = (row: any) => row.title
const zdData = ref<Array<any>>([])
const zdColumns: any = [
{
type: 'selection',
width: 60,
disabled(row: any) {
return row.title == '复选框' || row.title == '禁用列'
}
},
{
title: '字段名',
key: 'title'
}
]
onMounted(() => {
recordColumns.forEach((item: any) => {
zdData.value.push({ title: item.title })
zdCheckedRowKeys.value.push(item.title);
})
getTableWidth(columns, 0)
})
function filterCol(value:any){
loading.value = true;
columns = recordColumns.filter((item: any) => value.includes(item.title) )
scrollX.value = getTableWidth(columns, 0)
loading.value = false;
}
function getTableWidth(arr: any, preWidth: any){
var addWidth = preWidth;
arr.forEach((item: any) => {
if(item.children){
addWidth += getTableWidth(item.children, 0);
}else{
addWidth += item.width;
}
})
return addWidth;
}
</script>
实现结果:
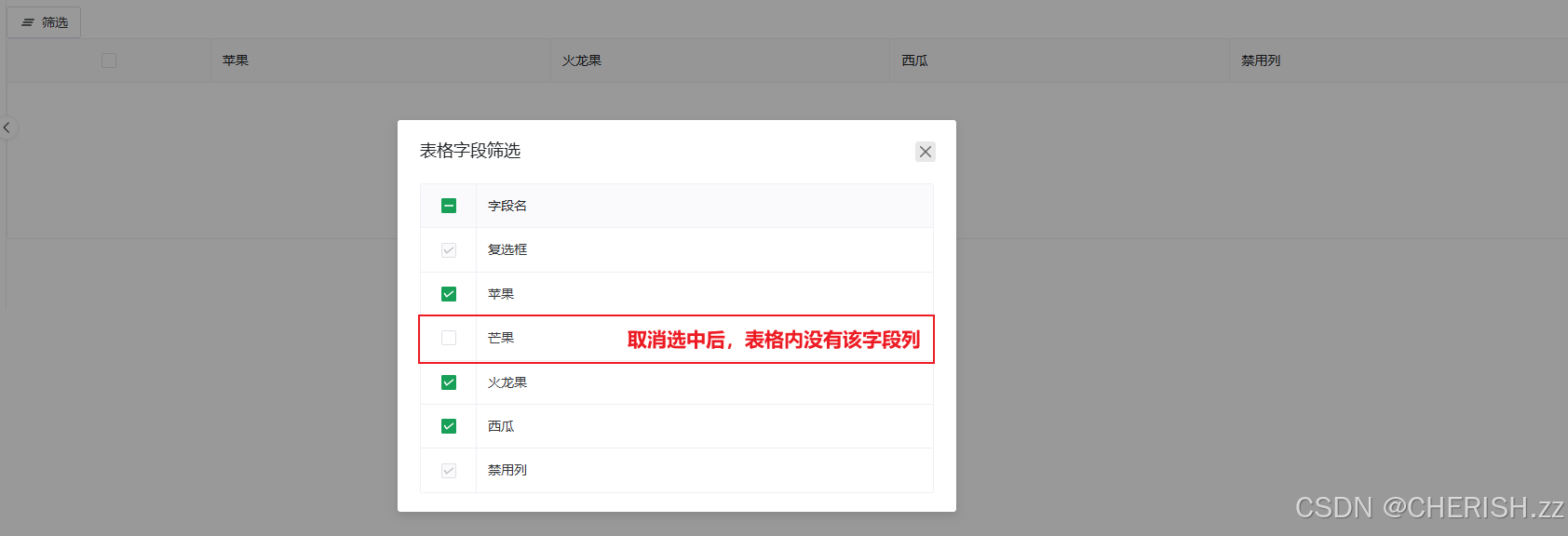