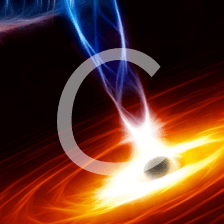
java开发
老汉在此
个人博客链接:https://blogofvictor.link/
展开
-
LintCode 15. 全排列
给定一个数字列表,返回其所有可能的排列。public class Solution { /* * @param nums: A list of integers. * @return: A list of permutations. */ public List<List<Integer>> permute(int[] nums...原创 2018-04-14 11:07:38 · 306 阅读 · 0 评论 -
二分查找
public class BinarySearch { public static void main(String[] args) { BinarySearch binarySearch = new BinarySearch(); int[] nums = {4,5,9,9,12,13,14,15,15,18}; System.out.println(binarySearch.bi...原创 2018-04-13 19:29:34 · 122 阅读 · 0 评论 -
使用CountDownLatch实现多线程同时启动
import java.util.concurrent.CountDownLatch;/** * 使用CountDownLatch实现多线程同时启动 * @author snlai */public class CountDownLatchTest { public static void main(String[] args) { System.out.pr...原创 2018-04-13 19:28:04 · 710 阅读 · 0 评论 -
LintCode 88. 最近公共祖先
给定一棵二叉树,找到两个节点的最近公共父节点(LCA)。最近公共祖先是两个节点的公共的祖先节点且具有最大深度。public class Solution { /* * @param root: The root of the binary search tree. * @param A: A TreeNode in a Binary. * @param B: ...原创 2018-04-22 17:43:33 · 318 阅读 · 0 评论 -
LintCode 87. 删除二叉查找树的节点
给定一棵具有不同节点值的二叉查找树,删除树中与给定值相同的节点。如果树中没有相同值的节点,就不做任何处理。你应该保证处理之后的树仍是二叉查找树。public class Solution { /* * @param root: The root of the binary search tree. * @param value: Remove the node with...原创 2018-04-22 17:42:07 · 241 阅读 · 0 评论 -
LintCode 84. 落单的数 III
给出2*n + 2个的数字,除其中两个数字之外其他每个数字均出现两次,找到这两个数字。public class Solution { /* * @param A: An integer array * @return: An integer array */ public List<Integer> singleNumberIII(int[...原创 2018-04-22 17:41:00 · 204 阅读 · 0 评论 -
LintCode 83. 落单的数 II
给出3*n + 1 个的数字,除其中一个数字之外其他每个数字均出现三次,找到这个数字。public class Solution { /** * @param A: An integer array * @return: An integer */ public int singleNumberII(int[] A) { // write your ...原创 2018-04-18 09:09:00 · 193 阅读 · 0 评论 -
LintCode 82. 落单的数
给出2*n + 1 个的数字,除其中一个数字之外其他每个数字均出现两次,找到这个数字。public class Solution { /** * @param A: An integer array * @return: An integer */ public int singleNumber(int[] A) { // write your co...原创 2018-04-18 09:07:20 · 237 阅读 · 0 评论 -
LintCode 75. 寻找峰值
你给出一个整数数组(size为n),其具有以下特点:相邻位置的数字是不同的A[0] < A[1] 并且 A[n - 2] > A[n - 1]假定P是峰值的位置则满足A[P] > A[P-1]且A[P] > A[P+1],返回数组中任意一个峰值的位置。public class Solution { /* * @param A: An integers arra...原创 2018-04-18 09:03:54 · 221 阅读 · 0 评论 -
LintCode 72. 中序遍历和后序遍历树构造二叉树
根据中序遍历和后序遍历树构造二叉树/** * Definition of TreeNode: * public class TreeNode { * public int val; * public TreeNode left, right; * public TreeNode(int val) { * this.val = val; * ...原创 2018-04-17 09:04:03 · 204 阅读 · 0 评论 -
LintCode 71. 二叉树的锯齿形层次遍历
给出一棵二叉树,返回其节点值的锯齿形层次遍历(先从左往右,下一层再从右往左,层与层之间交替进行) /** * Definition of TreeNode: * public class TreeNode { * public int val; * public TreeNode left, right; * public TreeNode(int val) {...原创 2018-04-17 09:02:43 · 184 阅读 · 0 评论 -
LintCode 70. 二叉树的层次遍历 II
给出一棵二叉树,返回其节点值从底向上的层次序遍历(按从叶节点所在层到根节点所在的层遍历,然后逐层从左往右遍历)/** * Definition of TreeNode: * public class TreeNode { * public int val; * public TreeNode left, right; * public TreeNode(int v...原创 2018-04-17 09:01:14 · 185 阅读 · 0 评论 -
LintCode 69. 二叉树的层次遍历
给出一棵二叉树,返回其节点值的层次遍历(逐层从左往右访问)/** * Definition of TreeNode: * public class TreeNode { * public int val; * public TreeNode left, right; * public TreeNode(int val) { * this.val ...原创 2018-04-17 08:59:41 · 192 阅读 · 0 评论 -
LintCode 66. 二叉树的前序遍历
给出一棵二叉树,返回其节点值的前序遍历。/** * Definition of TreeNode: * public class TreeNode { * public int val; * public TreeNode left, right; * public TreeNode(int val) { * this.val = val; *...原创 2018-04-17 08:57:07 · 283 阅读 · 0 评论 -
CountDownLatch使用Demo
import java.util.concurrent.CountDownLatch;public class CountDownLatchTest1 { public static void main(String[] args) { final CountDownLatch latch = new CountDownLatch(2); new Thread(){ publ...原创 2018-04-13 19:32:14 · 696 阅读 · 0 评论 -
二叉树深度和广度优先搜索
import java.util.LinkedList;public class DepthFirstSearchAndBreadthFirstSearch { public static class TreeNode { public int val; public TreeNode left, right; public TreeNode(int...原创 2018-04-13 19:34:06 · 173 阅读 · 0 评论 -
线程安全的双重检查单例模式
/** * 双重检查实现单例模式本身是线程不安全的,主要原因在于instance = new DoubleCheckSingleton()这句代码非原子操作,而编译器又存在指令重排序的问题, * 可能存在线程1先分配内存,然后将instance指向该内存,这时instance并不为空,但并未初始化,若此时线程2调用getInstance方法,则会直接返回instance,然而 * insta...原创 2018-04-13 19:37:56 · 932 阅读 · 0 评论 -
LintCode 14. 二分查找
给定一个排序的整数数组(升序)和一个要查找的整数target,用O(logn)的时间查找到target第一次出现的下标(从0开始),如果target不存在于数组中,返回-1。public class Solution { /** * @param nums: The integer array. * @param target: Target to find. ...原创 2018-04-14 11:05:52 · 124 阅读 · 0 评论 -
LintCode 13. 字符串查找
对于一个给定的 source 字符串和一个 target 字符串,你应该在 source 字符串中找出 target 字符串出现的第一个位置(从0开始)。如果不存在,则返回 -1。public class Solution { /* * @param source: source string to be scanned. * @param target: target...原创 2018-04-14 11:04:18 · 203 阅读 · 0 评论 -
LintCode 12. 带最小值操作的栈
实现一个带有取最小值min方法的栈,min方法将返回当前栈中的最小值。你实现的栈将支持push,pop 和 min 操作,所有操作要求都在O(1)时间内完成。import java.util.LinkedList;public class MinStack { public LinkedList dataStack; public LinkedList minStack; ...原创 2018-04-14 11:01:46 · 218 阅读 · 0 评论 -
LintCode 8. 旋转字符串
给定一个字符串和一个偏移量,根据偏移量旋转字符串(从左向右旋转)public class Solution { /** * @param str: An array of char * @param offset: An integer * @return: nothing */ public void rotateString(char[]...原创 2018-04-14 10:59:40 · 154 阅读 · 0 评论 -
LintCode 7. Serialize and Deserialize Binary Tree
设计一个算法,并编写代码来序列化和反序列化二叉树。将树写入一个文件被称为“序列化”,读取文件后重建同样的二叉树被称为“反序列化”。如何反序列化或序列化二叉树是没有限制的,你只需要确保可以将二叉树序列化为一个字符串,并且可以将字符串反序列化为原来的树结构。import java.util.LinkedList;/** * Definition of TreeNode: * public cl...原创 2018-04-14 10:57:55 · 178 阅读 · 0 评论 -
LintCode 6. 合并排序数组 II
合并两个排序的整数数组A和B变成一个新的数组。public class Solution { /** * @param A: sorted integer array A * @param B: sorted integer array B * @return: A new sorted integer array */ public int...原创 2018-04-14 10:50:26 · 212 阅读 · 0 评论 -
LintCode 5. 第k大元素
在数组中找到第k大的元素class Solution { /* * @param k : description of k * @param nums : array of nums * @return: description of return */ public int kthLargestElement(int k, int[] nu...原创 2018-04-14 10:47:34 · 205 阅读 · 0 评论 -
LintCode 2. 尾部的零
设计一个算法,计算出n阶乘中尾部零的个数public class Solution { /* * @param n: An integer * @return: An integer, denote the number of trailing zeros in n! */ public long trailingZeros(long n) { ...原创 2018-04-14 09:59:54 · 110 阅读 · 0 评论 -
LintCode 1. A + B 问题
给出两个整数a和b, 求他们的和, 但不能使用 +等数学运算符。/** * 不使用加法符号实现加法运算,通过位操作递归实现 * @author snlai * @create 2018-02-14 */public class Add { public static void main(String[] args) { int a = 3452434; ...原创 2018-04-14 09:53:02 · 208 阅读 · 0 评论 -
LintCode 90. k数和 II
Your title here...Given n unique integers, number k (1<=k<=n) and target.Find all possible k integers where their sum is target.public class Solution { /* * @param A: an integer array ...原创 2018-04-23 20:35:32 · 371 阅读 · 0 评论 -
LintCode 89. k数和
给定n个不同的正整数,整数k(k < = n)以及一个目标数字。 在这n个数里面找出K个数,使得这K个数的和等于目标数字,求问有多少种方案?public class Solution { /** * @param A: An integer array * @param k: A positive integer (k <= length(A)) ...原创 2018-04-23 20:34:15 · 485 阅读 · 0 评论 -
静态内部类加载实现单例模式
/** * 静态类内部加载 * 既可实现懒汉模式,又线程安全 * @author snlai */public class SingletonTest { private static class SingletonHolder { private static SingletonTest ourInstance = new SingletonTest(); }...原创 2018-04-13 19:40:47 · 232 阅读 · 0 评论 -
快速排序
public class QuickSort { public static void main(String[] args) { QuickSort quickSort = new QuickSort(); int[] array = {1,6,4,8,5,3,0,8,7}; quickSort.quickSort(array, 0, array.len...原创 2018-04-13 19:39:46 · 121 阅读 · 0 评论 -
LintCode 63. 搜索旋转排序数组 II
跟进“搜索旋转排序数组”,假如有重复元素又将如何?是否会影响运行时间复杂度?如何影响?为何会影响?写出一个函数判断给定的目标值是否出现在数组中。public class Solution { /** * @param A: an integer ratated sorted array and duplicates are allowed * @param target: A...原创 2018-04-17 08:55:29 · 144 阅读 · 0 评论 -
LintCode 62. 搜索旋转排序数组
假设有一个排序的按未知的旋转轴旋转的数组(比如,0 1 2 4 5 6 7 可能成为4 5 6 7 0 1 2)。给定一个目标值进行搜索,如果在数组中找到目标值返回数组中的索引位置,否则返回-1。你可以假设数组中不存在重复的元素。public class Solution { /** * @param A: an integer rotated sorted array * ...原创 2018-04-17 08:54:03 · 193 阅读 · 0 评论 -
LintCode 61. 搜索区间
给定一个包含 n 个整数的排序数组,找出给定目标值 target 的起始和结束位置。如果目标值不在数组中,则返回[-1, -1]public class Solution { /** * @param A: an integer sorted array * @param target: an integer to be inserted * @return: a l...原创 2018-04-17 08:52:07 · 201 阅读 · 0 评论 -
LintCode 32. 最小子串覆盖
给定一个字符串source和一个目标字符串target,在字符串source中找到包括所有目标字符串字母的子串。public class Solution { /** * @param source : A string * @param target: A string * @return: A string denote the minimum windo...原创 2018-04-15 13:15:49 · 841 阅读 · 0 评论 -
LintCode 31. 数组划分
给出一个整数数组 nums 和一个整数 k。划分数组(即移动数组 nums 中的元素),使得:所有小于k的元素移到左边所有大于等于k的元素移到右边返回数组划分的位置,即数组中第一个位置 i,满足 nums[i] 大于等于 k。public class Solution { /** * @param nums: The integer array you should partit...原创 2018-04-15 13:14:30 · 166 阅读 · 0 评论 -
LintCode 30. 插入区间
给出一个无重叠的按照区间起始端点排序的区间列表。在列表中插入一个新的区间,你要确保列表中的区间仍然有序且不重叠(如果有必要的话,可以合并区间)。/** * Definition of Interval: * public classs Interval { * int start, end; * Interval(int start, int end) { * ...原创 2018-04-15 13:12:34 · 147 阅读 · 0 评论 -
LintCode 29. 交叉字符串
给出三个字符串:s1、s2、s3,判断s3是否由s1和s2交叉构成。public class Solution { /** * @param s1: A string * @param s2: A string * @param s3: A string * @return: Determine whether s3 is formed by int...原创 2018-04-15 13:11:06 · 234 阅读 · 0 评论 -
LintCode 28. 搜索二维矩阵
写出一个高效的算法来搜索 m × n矩阵中的值。这个矩阵具有以下特性:每行中的整数从左到右是排序的。每行的第一个数大于上一行的最后一个整数public class Solution { /** * @param matrix: matrix, a list of lists of integers * @param target: An integer * @...原创 2018-04-15 13:09:17 · 125 阅读 · 0 评论 -
LintCode 24. LFU缓存
LFU是一个著名的缓存算法实现LFU中的set 和 getpublic class LFUCache { private Map<Integer, Node> cache; private int capacity = 0; private int used = 0; /* * @param capacity: An integer ...原创 2018-04-15 13:07:45 · 545 阅读 · 0 评论 -
LintCode 20. 骰子求和
扔 n 个骰子,向上面的数字之和为 S。给定 Given n,请列出所有可能的 S 值及其相应的概率。public class Solution { /** * @param n an integer * @return a list of Map.Entry<sum, probability> */ public List<Map....原创 2018-04-15 13:06:05 · 215 阅读 · 0 评论