题目:剑指 Offer 22. 链表中倒数第k个节点
题目描述:输入一个链表,输出该链表中倒数第k个节点。为了符合大多数人的习惯,本题从1开始计数,即链表的尾节点是倒数第1个节点。例如,一个链表有6个节点,从头节点开始,它们的值依次是1、2、3、4、5、6。这个链表的倒数第3个节点是值为4的节点。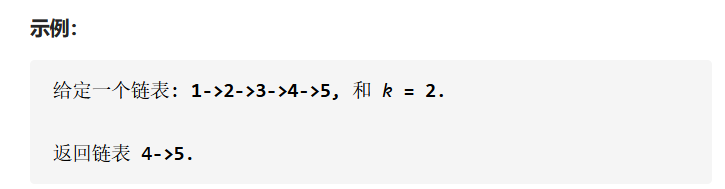
代码:
class Solution {
public:
ListNode* getKthFromEnd(ListNode* head, int k) {
if(head==nullptr)
return head;
ListNode*p1=head,*p2=head;
while(k&&p1){
p1=p1->next;
k--;
}
while(p1){
p1=p1->next;
p2=p2->next;
}
return p2;
}
};
题目:剑指 Offer 42. 连续子数组的最大和
题目描述:输入一个整型数组,数组中的一个或连续多个整数组成一个子数组。求所有子数组的和的最大值。
要求时间复杂度为O(n)。
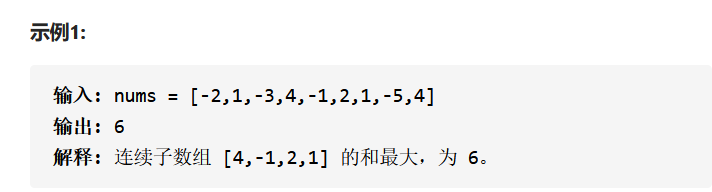
代码:
class Solution {
public:
int maxSubArray(vector<int>& nums) {
int pre=0,res=nums[0];
for(int &i:nums){
pre=max(pre+i,i);
res=max(res,pre);
}
return res;
}
};
题目:剑指 Offer 21. 调整数组顺序使奇数位于偶数前面
题目描述:输入一个整数数组,实现一个函数来调整该数组中数字的顺序,使得所有奇数位于数组的前半部分,所有偶数位于数组的后半部分。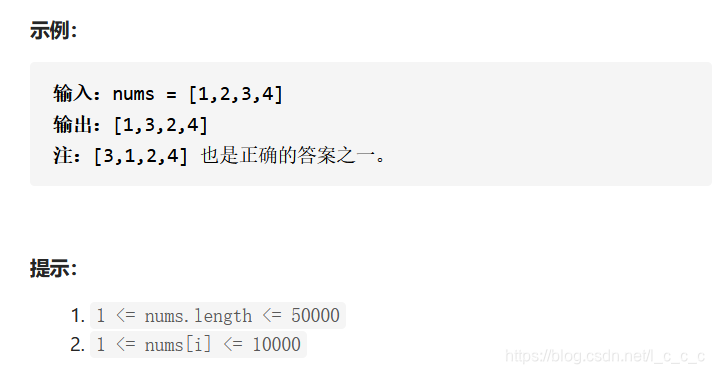
代码:
class Solution {
public:
vector<int> exchange(vector<int>& nums) {
vector<int>res(nums.size());
int i=0,j=nums.size()-1;
for(int n:nums){
if(n%2==1) res[i++]=n;
else res[j--]=n;
}
return res;
}
};
题目:剑指 Offer 10- I. 斐波那契数列
题目描述: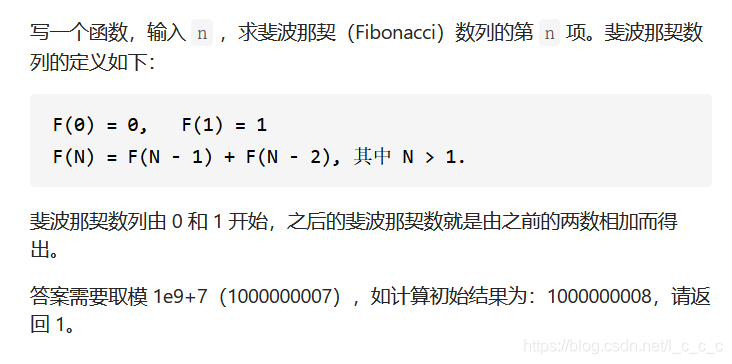
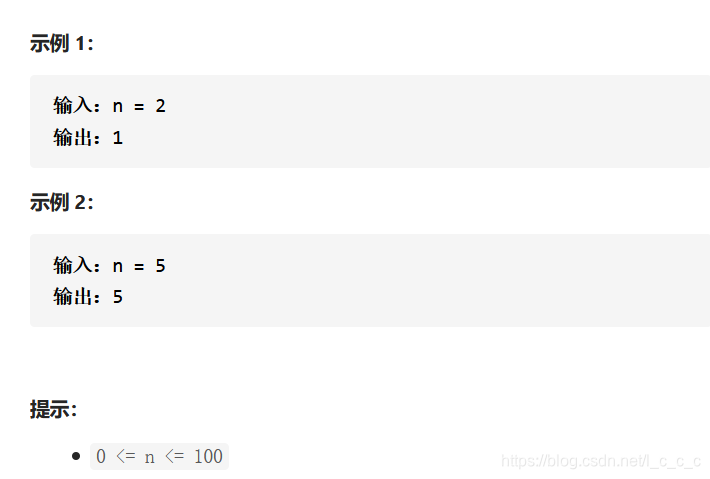
解题思路:递归问题。
代码:
class Solution {
public:
int fib(int n) {
if(n==0) return 0;
vector<int>dp(n+1);
dp[0]=0;
dp[1]=1;
for(int i=2;i<=n;i++)
dp[i]=(dp[i-1]+dp[i-2])%1000000007;
return dp[n];
}
};
题目:剑指 Offer 28. 对称的二叉树
题目描述:请实现一个函数,用来判断一棵二叉树是不是对称的。如果一棵二叉树和它的镜像一样,那么它是对称的。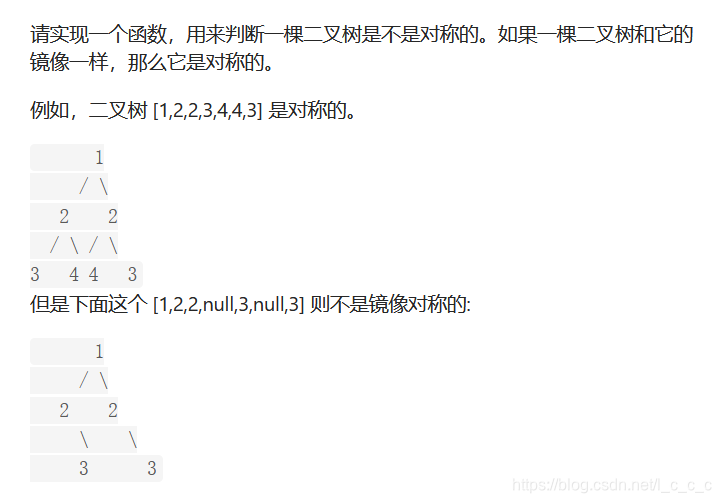
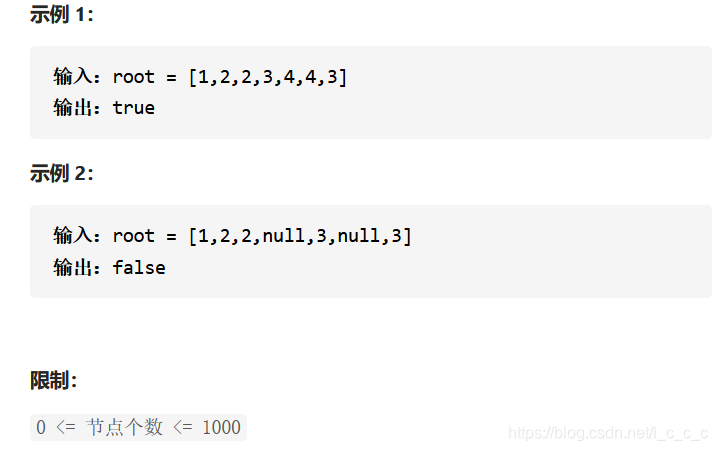
递归代码:
class Solution {
public:
bool isSymmetric(TreeNode* root) {
if(!root) return true;
else return isSymmetric(root->left,root->right);
}
bool isSymmetric(TreeNode*a,TreeNode*b){
if(!(a||b)) return true;
else if(!(a&&b)) return false;
else return a->val==b->val&&isSymmetric(a->left,b->right)&&isSymmetric(a->right,b->left);
}
};
非递归代码:
class Solution {
public:
bool isSymmetric(TreeNode* root) {
if(root==nullptr)
return true;
queue<TreeNode*>q;
q.push(root->left);
q.push(root->right);
while(!q.empty()){
TreeNode*A=q.front();
q.pop();
TreeNode*B=q.front();
q.pop();
if(A==nullptr&&B==nullptr)
continue;
if(A==nullptr||B==nullptr)
return false;
if(A->val!=B->val)
return false;
q.push(A->left);
q.push(B->right);
q.push(A->right);
q.push(B->left);
}
return true;
}
};
题目:剑指 Offer 32 - II. 从上到下打印二叉树 II
题目描述:从上到下按层打印二叉树,同一层的节点按从左到右的顺序打印,每一层打印到一行。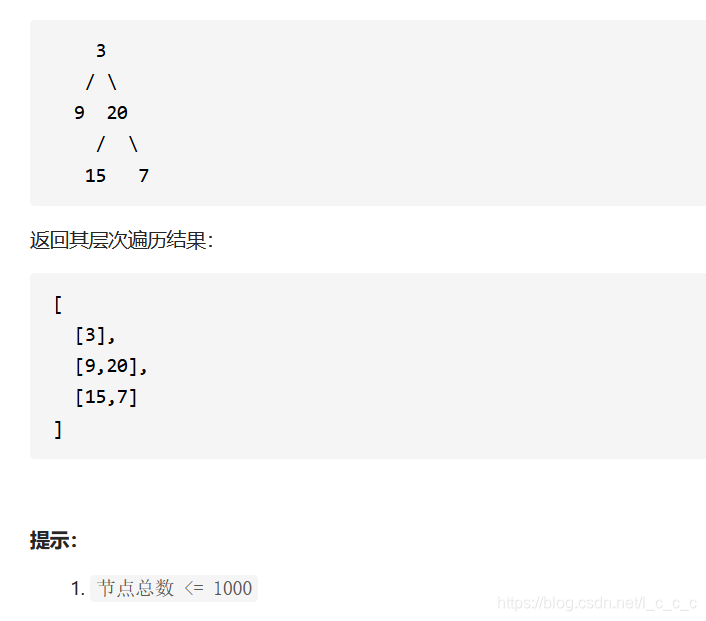
代码:
class Solution {
public:
vector<vector<int>> levelOrder(TreeNode* root) {
vector<vector<int>>res;
if(root==nullptr) return res;
queue<TreeNode*>q;
q.push(root);
while(!q.empty()){
vector<int>r;
int l=q.size();
for(int i=0;i<l;i++){
TreeNode*t=q.front();
q.pop();
r.push_back(t->val);
if(t->left) q.push(t->left);
if(t->right) q.push(t->right);
}
res.push_back(r);
}
return res;
}
};
题目:剑指 Offer 06. 从尾到头打印链表
题目描述:输入一个链表的头节点,从尾到头反过来返回每个节点的值(用数组返回)。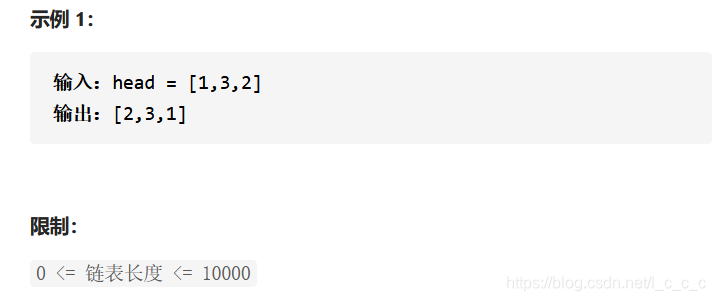
代码:
class Solution {
public:
vector<int> reversePrint(ListNode* head) {
vector<int>res;
stack<int>s;
ListNode*cur=head;
while(cur){
s.push(cur->val);
cur=cur->next;
}
while(!s.empty()){
res.push_back(s.top());
s.pop();
}
return res;
}
};
代码:
class Solution {
public:
vector<int> reversePrint(ListNode* head) {
vector<int>res;
while(head){
res.push_back(head->val);
head=head->next;
}
reverse(res.begin(),res.end());
return res;
}
};