#include <iostream>
using namespace std;
class Point{
private:
int x,y;
static int count;
public:
Point(int x = 0,int y = 0):x(x),y(y){
count++;
}
Point(Point &p){ //复制构造函数
x = p.x;
y = p.y;
count++;
}
~Point(){ count--; }
int getX() {return x;}
int getY() {return y;}
void showCount(){ //输出静态数据成员
cout << " Object count = " << count << endl;
}
static void showCount1(){ //静态函数成员
cout << " Object count = " << count << endl;
}
};
int Point::count = 0; //静态数据成员定义和初始化,使用类名限定
int main(){
Point a(4,5); //定义对象a,其构造函数会使count增1
cout << "Point A: " << a.getX() << "," << a.getY() << endl;
a.showCount();
Point c = a;
cout << "Point C: " << c.getX() << "," << c.getY() << endl;
c.showCount1();
Point b; //定义对象b,其构造函数会使count增1
cout << "Point B: " << b.getX() << "," << b.getY() << endl;
Point::showCount1();
return 0;
}
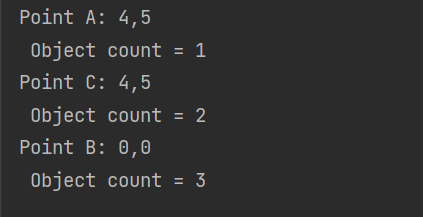