1.源程序
#include <iostream>
using namespace std;
class Animal
{
public:
Animal()
{
cout << "animal construct" << endl;
cout << "******************\n";
}
Animal(int height,int weight)
{
cout << "animal construct with two parameters" << endl;
cout << "******************\n";
}
~Animal()
{
cout << "animal deconstruct" << endl;
cout << "******************\n";
}
void eat()
{
cout << "animal eat" << endl;
}
void breathe()
{
cout << "animal breathe" << endl;
}
virtual void run()
{
cout << "animal run" << endl;
}
};
class Fish : public Animal
{
public:
Fish():Animal(400,300),a(1)
{
cout << "fish construct" << endl;
cout << "******************\n";
}
~Fish( )
{
cout << "fish deconstruct" << endl;
cout << "******************\n";
}
void breathe()
{
Animal::breathe();
cout << "fish bubble" << endl;
}
void run()
{
cout << "fish run" << endl;
}
void sleep()
{
cout << "fish sleep" << endl;
}
private:
const int a;
};
void fn(Animal* pAn)
{
pAn->breathe();
}
void fn2(Animal* pAn2)
{
pAn2->run();
}
void main()
{
Animal an;
an.eat();
cout << "******************\n";
Fish fh;
fh.breathe();
cout << "******************\n类型转换(内存模型要一致)\n";
Animal* pAn;
pAn = &fh;
fn(pAn);
cout << "******************\nC++的多态性:(virtual)传递子类的地址,子类有的调用子类的,子类没有的再调用父类的\n";
Animal* pAn2;
pAn2 = &fh;
fn2(pAn2);
cout << "******************\n引用(变量的别名)\n";
int a = 6;
cout << "a = " << a <<endl;
int& b = a;
b = 5;
cout << "a = " << a << endl;
}
2.运行结果
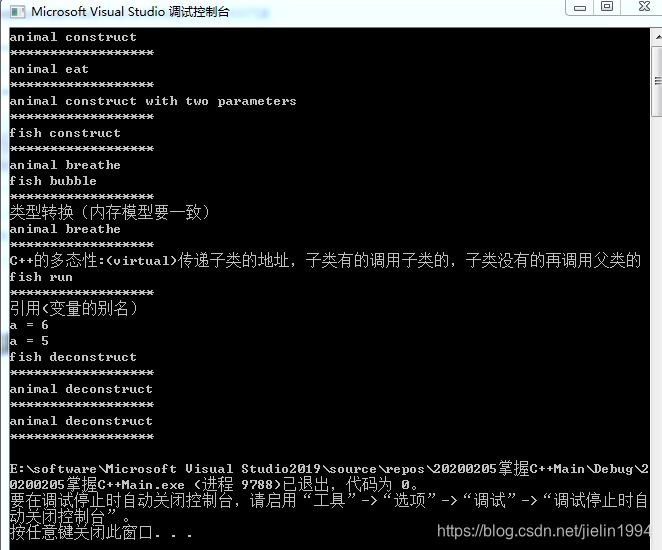
3.整理源程序
main.cpp
#include "Animal.h"
#include "Fish.h"
#include <iostream>
using namespace std;
void fn(Animal* pAn)
{
pAn->breathe();
}
void fn2(Animal* pAn2)
{
pAn2->run();
}
void main()
{
Animal an;
an.eat();
cout << "******************\n";
Fish fh;
fh.breathe();
cout << "******************\n类型转换(内存模型要一致)\n";
Animal* pAn;
pAn = &fh;
fn(pAn);
cout << "******************\nC++的多态性:(virtual)传递子类的地址,子类有的调用子类的,子类没有的再调用父类的\n";
Animal* pAn2;
pAn2 = &fh;
fn2(pAn2);
cout << "******************\n引用(变量的别名)\n";
int a = 6;
cout << "a = " << a <<endl;
int& b = a;
b = 5;
cout << "a = " << a << endl;
}
animal.h
#ifndef ANIMAL_H_H
#define ANIMAL_H_H
class Animal
{
public:
Animal();
Animal(int height, int weight);
~Animal();
void eat();
void breathe();
virtual void run();
};
#endif
animal.cpp
#include "Animal.h"
#include <iostream>
using namespace std;
Animal::Animal()
{
cout << "animal construct" << endl;
cout << "******************\n";
}
Animal::Animal(int height, int weight)
{
cout << "animal construct with two parameters" << endl;
cout << "******************\n";
}
Animal::~Animal()
{
cout << "animal deconstruct" << endl;
cout << "******************\n";
}
void Animal::eat()
{
cout << "animal eat" << endl;
}
void Animal::breathe()
{
cout << "animal breathe" << endl;
}
void Animal::run()
{
cout << "animal run" << endl;
}
fish.h
#include "Animal.h"
#ifndef FISH_H_H
#define FISH_H_H
class Fish : public Animal
{
public:
Fish();
~Fish();
void breathe();
void run();
void sleep();
private:
const int a;
};
#endif
fish.cpp
#include "Fish.h"
#include <iostream>
using namespace std;
Fish::Fish() :Animal(400, 300), a(1)
{
cout << "fish construct" << endl;
cout << "******************\n";
}
Fish::~Fish()
{
cout << "fish deconstruct" << endl;
cout << "******************\n";
}
void Fish::breathe()
{
Animal::breathe();
cout << "fish bubble" << endl;
}
void Fish::run()
{
cout << "fish run" << endl;
}
void Fish::sleep()
{
cout << "fish sleep" << endl;
}
4.课程链接
孙鑫
5.讲义
