线性表:有相同特性的元素构成的有限序列,物理上存储通常用数组或者链表
常见线性表:顺序表、链表、栈、队列、字符串
顺序表:元素存储在物理上连续,一般用数组实现
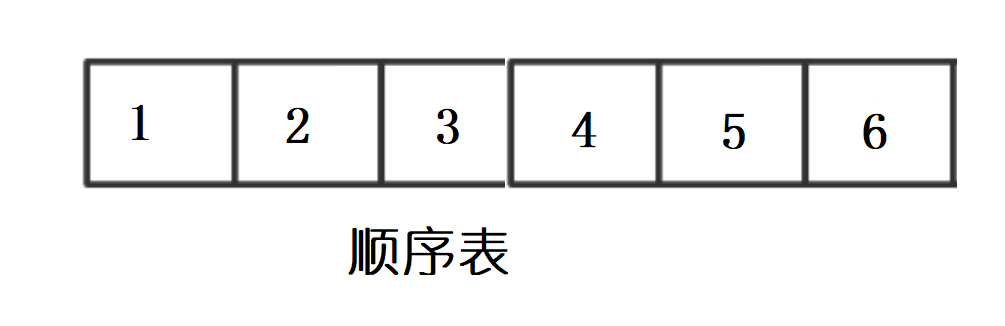
顺序表实现
以下代码可以看出顺序表不适合头插、头删,每次都需要移动全部数据
定义结构体
typedef int SLTDataType;
typedef struct SepList
{
SLDatatype* a;
int size;
int capacity;//数组容量
}SL;
初始化结构体
void SLInit(SL* ps)
{
assert(ps);
ps->a = (SLDataType*)malloc(sizeof(SLDataType)* 4);
if (ps->a == NULL)
{
perror("malloc fail");
return;
}
ps->size = 0;
ps->capacity = 4;
}
扩容
该函数是在插入之前检查数组是否需要扩容
void CheckCapcity(SL* ps)//
{
assert(ps);
if (ps->size == ps->capacity)
{
SLDatatype* tmp = (SLDatatype*)realloc(ps, sizeof(SLDatatype) * ps->capacity * 2);
if (ps == NULL)
{
perror(realloc);
}
ps->a = tmp;
ps->capacity *= 2;
}
}
尾插
void SLPushBack(SL* ps, SLDatatype x)
{
assert(ps);
CheckCapcity(ps);
ps->a[ps->size++] = x;
}
尾删
尾删不需要删除尾部数据,只要让数组大小减一,数组就不会去访问那个值
void SLPopBack(SL* ps)
{
assert(ps->size>0);//
ps->size--;
}
头插
把所有数据往后移动,再把a[0]位置赋值
void SLPushFront(SL* ps, SLDatatype x)
{
assert(ps);
CheckCapcity(ps);
int end = ps->size - 1;
while (end>=0)
{
ps->a[end+1] = ps->a[end];
end--;
}
ps->a[0] = x;
ps->size++;
}
头删
将第二个数据及其以后往前移动
void SLPopFront(SL* ps)
{
assert(ps);
int begain = 0;
while (begain < ps->size)
{
ps->a[begain] = ps->a[begain+1];
begain++;
}
ps->size--;
}
指定位置插入
这段是在指定位置之后插入,因为数组从0开始,pos会大1
void SLInsert(SL* ps, int pos, SLDatatype x)
{
assert(ps);
assert(pos >= 0 && pos <= ps->size);
int end = ps->size;
while (end > pos)
{
ps->a[end] = ps->a[end - 1];
end--;
}
ps->a[pos] = x;
ps->size++;
}
指定位置删除
将a[pos]之后的数据往前移动覆盖需要删除的值
void SLErase(SL* ps, SLDatatype pos)
{
assert(ps);
assert(pos >= 0 && pos < ps->size);
int begain = pos;
while (begain < ps->size)
{
ps->a[begain] = ps->a[begain+1];
begain++;
}
ps->size--;
}
销毁
void SLDestroy(SL* ps)
{
assert(ps);
free(ps->a);
ps->a = NULL;
ps->capacity = ps->size = 0;
}
查找
int SLFind(SL* ps, SLDatatype x)
{
assert(ps);
for (int i = 0; i < ps->size; i++)
{
if (ps->a[i] == x)
return i;
}
}
链表:逻辑上连续,但是物理存储结构上非连续、非顺序的存储结构 ,一般用指针链接
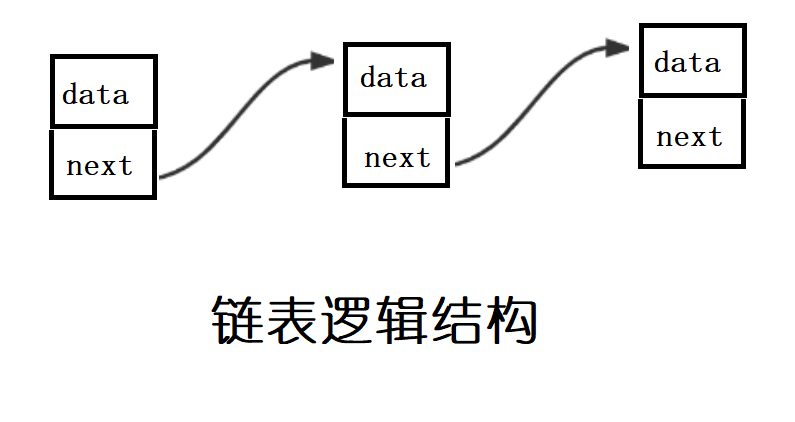
链表实现
单链表
单链表以下代码可以看出虽然单链表适合头插、头删,但是并不适合尾插、尾删,这需要遍历链表
单链表调用函数时,传的是结构体的二级指针,因为要改变结构体结点指向
定义结构体
typedef int SLTDataType;
typedef struct SLTNode
{
SLTDataType data;
struct SLTNode* next;
}SLTNode;
建立新节点
插入数据需要建立新节点
SLTNode* BuyNode(SLTDataType x)
{
SLTNode* newnode = (SLTNode*)malloc(sizeof(SLTNode));
if (newnode == NULL)
{
perror(malloc);
return 1;
}
newnode->data = x;
newnode->next = NULL;
return newnode;
}
头插
void SLTPushFront(SLTNode** pphead, SLTDataType x)
{
SLTNode* newnode = BuyNode(x);
SLTNode* first = *pphead;
newnode->next = first;
*pphead = newnode;
}
尾插
void SLTPushBack(SLTNode** pphead, SLTDataType x)
{
SLTNode* newnode= BuyNode(x);
if (*pphead == NULL)
{
*pphead = newnode;
}
else
{
SLTNode* tail = *pphead;
while (tail->next != NULL)
{
tail = tail->next;
}
tail->next = newnode;
}
}
头删
void SLTPopFront(SLTNode** pphead)
{
assert(*pphead);
SLTNode* first = *pphead;
*pphead = first->next;
free(first);
first = NULL;
}
尾删
void SLTPopBack(SLTNode** pphead)
{
assert(*pphead);
SLTNode* tail = *pphead;
while (tail->next->next != NULL)//找尾
{
tail = tail->next;
}
free(tail->next);
tail->next = NULL;
}
查找
SLTNode* SLTFind(SLTNode* phead, SLTDataType x)
{
assert(phead);
SLTNode* cur = phead;
while (cur)
{
if (cur->data == x)
{
return cur;
}
cur = cur->next;
}
return NULL;
}
指定位置插入
void SLTInsert(SLTNode** pphead, SLTNode* pos, SLTDataType x)
{
assert(pos);
assert(pphead);
SLTNode* newnode = BuyNode(x);
if (*pphead == pos)
{
SLTPushFront(pphead, x);
}
else
{
SLTNode* cur = *pphead;
while (cur->next != pos)
{
cur = cur->next;
}
newnode->next = pos;
cur->next = newnode;
}
}
指定位置删除
void SLTErase(SLTNode** pphead, SLTNode* pos)
{
assert(*pphead);
assert(pphead);
assert(pos);
SLTNode* cur = *pphead;
while (cur->next!=pos)
{
cur = cur->next;
}
SLTNode* del = cur->next;
cur->next = cur->next->next;
free(del);//在函数外置空
}
销毁
void SLTDestory(SLTNode** pphead)
{
assert(*pphead);
assert(pphead);
SLTNode* cur = *pphead;
while (cur)
{
SLTNode* pre = cur->next;
free(cur);
cur = NULL;
cur = pre;
}
}
循环链表
循环链表节点,需要一个指针指向前一个节点,一个指针指向后一个节点
在表达式中存在“->”和其他操作符时,编译器会优先计算“->”操作符的结果,所以(*pphead)要先用()再->
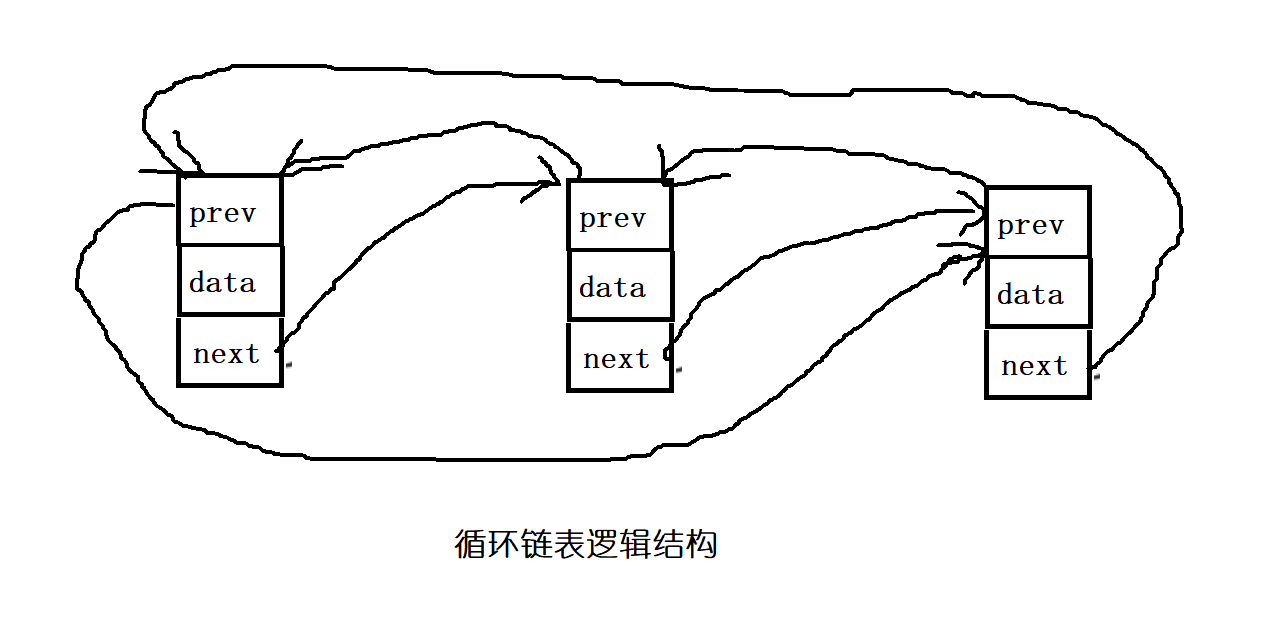
定义结构体
typedef int SLDataType;
typedef struct SLNode
{
struct SLNode* prev;
struct SLNode* next;
SLDataType x;
}SLNode;
初始化结构体
初始化结构体时,初始化一个头节点
SLNode* SLInit()
{
SLNode* phead = BuySLNode(-1);
phead->next = phead;
phead->prev = phead;
return phead;
}
头插
void SLPushFront(SLNode* phead, SLDataType x)
{
SLNode* newnode = BuySLNode(x);
SLNode* headnext = phead->next;
newnode->next = headnext;
headnext->prev = newnode;
phead->next = newnode;
newnode->prev = phead;
}
尾插
void SLPushback(SLNode* phead, SLDataType x)
{
assert(phead);
SLNode* newnode=BuySLNode(x);
SLNode* tail = phead->prev;
tail->next = newnode;
newnode->prev = tail;
phead->prev = newnode;
newnode->next = phead;
}
尾删
void SLPopback(SLNode* phead)
{
assert(phead);
SLNode* tail = phead->prev;
SLNode* prev = tail->prev;
prev->next = phead;
phead->prev = prev;
free(tail);
tail = NULL;
}
头删
void SLPopFront(SLNode* phead)
{
SLNode* pheadnext = phead->next;
SLNode* newhead = pheadnext->next;
phead->next = newhead;
newhead->prev = phead;
free(pheadnext);
pheadnext = NULL;
}
查找
SLNode* SLFind(SLNode* phead, SLDataType x)
{
assert(phead);
SLNode* cur = phead->next;
while (cur != phead)
{
if (cur->x==x)
{
return cur;
}
cur = cur->next;
}
return NULL;
}
指定位置删除
使用之前需要用上面SLFind函数找到删除的位置
void SLErase(SLNode* pos)
{
assert(pos);
SLNode* prev = pos->prev;
SLNode* next = pos->next;
prev->next = next;
next->prev = prev;
free(pos);
pos = NULL;
}
指定位置插入
使用之前需要用上面SLFind函数找到删除的位置
void SLInsert(SLNode* pos, SLDataType x)
{
SLNode* prev = pos->prev;
SLNode* newnode = BuySLNode(x);
pos->prev = newnode;
newnode->next = pos;
prev->next = newnode;
newnode->prev = prev;
}
销毁
void SLDestroy(SLNode**pphead)
{
assert(pphead);
assert(*pphead);
SLNode* cur = *pphead;
SLNode* prev = (*pphead)->prev;
prev->next = NULL;
while (cur)
{
cur = (*pphead)->next;
free(*pphead);
*pphead = cur;
}
}