Go开发之旅
第二章 Iris的MVC框架使用
一、Iris是什么?
Iris 是一个快速、简单但功能齐全且非常高效的 Go Web 框架。
二、使用步骤
1.安装命令
go get github.com/kataras/iris/v12
2.使用
// 创建实例
app := iris.New()
// 配置日志级别
app.Logger().SetLevel("debug")
// 启用Recover
app.Use(recover.New())
// 配置 HttpRequst
app.Use(cors.New(cors.Options{
AllowedOrigins: []string{"*"},
AllowCredentials: true,
MaxAge: 600,
AllowedMethods: []string{iris.MethodGet, iris.MethodPost, iris.MethodOptions, iris.MethodHead, iris.MethodDelete, iris.MethodPut},
AllowedHeaders: []string{"*"},
}))
// 配置Http请求方式
app.AllowMethods(iris.MethodOptions)
// 配置错误
app.OnAnyErrorCode(func(ctx iris.Context) {
path := ctx.Path()
var err error
if strings.Contains(path, "/api/admin/") {
err = ctx.JSON(web.JsonErrorCode(ctx.GetStatusCode(), "Http error"))
}
if err != nil {
logrus.Error(err)
}
})
// 配置license检查
licenseInfo := license.DeEncrypt()
var licenseMap map[string]interface{}
json.Unmarshal([]byte(licenseInfo), &licenseMap)
deadline, _ := dates.Parse(licenseMap["expireTime"].(string), dates.FmtDate)
// 创建一个中间件来检查访问有效期
licenseMiddleware := func(ctx iris.Context) {
skipFlag := false
if strings.Contains(ctx.Path(), "license") {
skipFlag = true
}
// 如果当前时间超过截止日期
if !skipFlag && time.Now().After(deadline) {
ctx.StatusCode(iris.StatusForbidden) // 设置状态码为 403 Forbidden
ctx.Writef("License 失效")
return // 不再处理后续的请求
}
ctx.Next() // 继续处理请求
}
// 应用中间件
app.Use(licenseMiddleware)
// 配置特殊的地址
app.Any("/", func(i iris.Context) {
_, _ = i.HTML("<h1>Powered by Iris</h1>")
})
// 配置日志
skipPaths := []string{"/health-check"}
var shouldSkips []logger.SkipperFunc
shouldSkip := logger.SkipperFunc(func(ctx iris.Context) bool {
//fmt.Printf("请求路径:%s\n", ctx.Path())
for _, path := range skipPaths {
if strings.HasSuffix(path, ctx.Path()) {
return true
}
}
return false
})
shouldSkips = append(shouldSkips, shouldSkip)
// 使用跳过函数作为条件
customLogger := logger.New(logger.Config{
// 当返回 true 时, 跳过日志
Skippers: shouldSkips,
IP: true,
Method: true,
Path: true,
Query: false,
})
app.Use(customLogger)
// MVC配置
mvc.Configure(app.Party("/"), func(m *mvc.Application) {
m.Party("/login").Handle(new(system.LoginController))
})
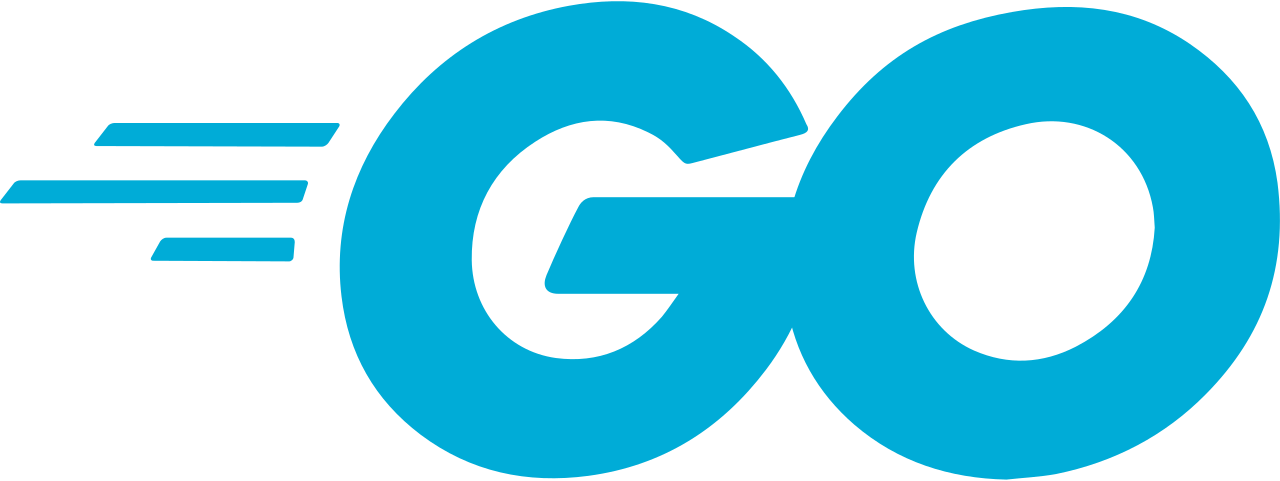
// 配置启动参数
if err := app.Listen(config.Instance.Host+":"+config.Instance.Port,
iris.WithConfiguration(iris.Configuration{
VHost: config.Instance.Host,
DisableStartupLog: false,
DisableInterruptHandler: false,
DisablePathCorrection: false,
EnablePathEscape: false,
FireMethodNotAllowed: false,
DisableBodyConsumptionOnUnmarshal: false,
DisableAutoFireStatusCode: false,
EnableOptimizations: true,
TimeFormat: "2006-01-02 15:04:05",
Charset: "UTF-8",
}),
); err != nil {
logrus.Error(err)
os.Exit(-1)
}