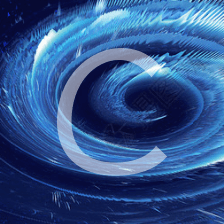
LeetCode
風中塵埃
这个作者很懒,什么都没留下…
展开
-
LeetCode 19. Remove Nth Node From End of List
这道题我看了答案,使用了双指针法来解决问题, class Solution { public: ListNode* removeNthFromEnd(ListNode* head, int n) { ListNode* dummy = new ListNode(0, head); ListNode* first = head; ListNode* second = dummy; for (int i = 0; i ...原创 2021-08-17 23:13:31 · 294 阅读 · 0 评论 -
剑指 Offer 35. 复杂链表的复制 LCOF
这道题看答案了,哈希做法很不错。 class Solution { public: Node* copyRandomList(Node* head) { if (head == nullptr) return nullptr; Node* cur = head; unordered_map<Node*, Node*> map; while (cur != nullptr) {原创 2021-08-13 12:25:35 · 208 阅读 · 0 评论 -
剑指 Offer 24. 反转链表 LCOF
用迭代的方法, class Solution { public: ListNode* reverseList(ListNode* head) { ListNode* prev = nullptr; ListNode* curr = head; while (curr) { ListNode* temp = curr->next; curr->next = prev;原创 2021-08-11 21:36:53 · 233 阅读 · 0 评论 -
剑指 Offer 06. 从尾到头打印链表 LCOF
利用一个堆栈,现将数组中的数值依次放入堆栈中,之后从堆栈中依次取出就是从尾到头顺序了。 class Solution { public: vector<int> reversePrint(ListNode* head) { stack<int> stack; vector<int> temp; while (head) { stack.push(head->val);原创 2021-08-11 20:43:40 · 95 阅读 · 0 评论 -
剑指 Offer 30. 包含min函数的栈 LCOF
class MinStack { public: MinStack() { } void push(int x) { stack.push(x); if (min_stack.empty() || min_stack.top() >= x) { min_stack.push(x); } } void pop() { if (!stack.empty().原创 2021-08-10 16:27:35 · 98 阅读 · 0 评论 -
剑指 Offer 09. 用两个栈实现队列 LCOF
这道题就是一个堆栈出,另一个堆栈进,来模拟一个队列。 class CQueue { public: CQueue() { while (!stack1.empty()) stack1.pop(); while (!stack2.empty()) stack2.pop(); } void appendTail(int value) { stack1.push(value); } int delet原创 2021-08-10 16:10:18 · 178 阅读 · 0 评论 -
LeetCode 876. Middle of the Linked List
这道题不会直接看答案,快慢指针法很爽,后面好好研究研究快慢指针法。 class Solution { public: ListNode* middleNode(ListNode* head) { ListNode* slow = head; ListNode* fast = head; while (fast != NULL && fast->next != NULL) { slow = slo...原创 2021-08-05 23:27:09 · 100 阅读 · 0 评论 -
LeetCode 557. Reverse Words in a String III
这道题参考答案,主要是要处理字符里的空格。 class Solution { public: string reverseWords(string s) { const int n = s.length(); int i = 0; while (i < n) { int start = i; while (i < n && s[i] != ' ') i++; ..原创 2021-08-04 21:55:48 · 105 阅读 · 0 评论 -
LeetCode 344. Reverse String
双指针解法: class Solution { public: void reverseString(vector<char>& s) { int p = 0, q = s.size() - 1; while (p <= q) { swap(s[p], s[q]); ++p; --q; } } };原创 2021-08-04 21:40:16 · 171 阅读 · 0 评论 -
LeetCode 283. Move Zeros
遍历数组,找出不是0的数,将他们覆盖给另一个都为0的数组中即可。 class Solution { public: void moveZeros(vector<int>& nums) { const int n = nums.size(); vector<int> newAddr(n); int j = 0; for (int i = 0; i < n; ++i) { ...原创 2021-08-03 19:54:12 · 182 阅读 · 0 评论 -
LeetCode 189. Rotate Array
class Solution { public: void rotate(vector<int>& nums, int k) { const int n = nums.size(); vector<int> newArr(n); for (int i = 0; i < n; ++i) { newArr[(k + i) % n] = nums[i];...原创 2021-08-03 17:57:56 · 192 阅读 · 0 评论 -
LeetCode 977.Squares of a Sorted Array 做题记录
这道题暴力破解的解法: class Solution { public: vector<int> sortedSquares(vector<int>& A) { vector<int> B; for (int x : A) { B.push_back(x * x); } sort(B.begin(), B.end()); ...原创 2021-08-03 17:47:50 · 232 阅读 · 0 评论 -
LeetCode 18. 4Sum 做题记录
思路: 一般会用到hash,这道题参考花花酱的。 class Solution { public: vector<vector<int>> fourSum(vector<int>& nums, int target) { sort(nums.begin(), nums.end()); if (target > 0 && target > 4 * nums.back()) retu...原创 2021-07-14 11:09:51 · 211 阅读 · 0 评论 -
LeetCode 669. Trim a Binary Search Tree 做题记录
题目: /** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode() : val(0), left(nullptr), right(nullptr) {} * TreeNode(int x) : val(x), left(nullptr), right(nullp原创 2021-01-21 09:30:28 · 126 阅读 · 0 评论 -
LeetCode 122. Best Time to Buy and Sell Stock II
题目: 采用动态规划来做这道题。 class Solution { public: int maxProfit(vector<int>& prices) { const int len = prices.size(); if (len < 2) return 0; vector<vector<int>> dp(len, vector<int>(2, 0));原创 2021-01-20 16:34:26 · 82 阅读 · 0 评论 -
LeetCode 718. Maximum Length of Repeated Subarray 做题记录
题目: 利用动态规划,来解这道题 class Solution { public: int findLength(vector<int>& A, vector<int>& B) { const int m = A.size(); const int n = B.size(); vector<vector<int>> dp(n + 1, vector<int>(m原创 2021-01-19 16:32:20 · 111 阅读 · 0 评论 -
LeetCode 101. Symmetric Tree 做题记录
题目: 利用深度优先算法,依次对数组进行遍历。 /** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */ class Solution { public: b原创 2021-01-12 15:24:24 · 90 阅读 · 0 评论 -
LeetCode 561.Array Partition I 做题记录
题目: 先对数组进行排序,然后进行数的累加,隔一个数累加一个。 class Solution { public: int arrayPairSum(vector<int>& nums) { sort(nums.begin(), nums.end()); const int n = nums.size(); int sum = 0; for (int i = 0; i < n; i += 2) {原创 2021-01-12 15:08:57 · 94 阅读 · 0 评论 -
LeetCode 442. Find All Duplicates in an Array 做题记录
题目: 我的思路是先对数组排序,然后看其中的数和它的下一个数是不是相等的,如果相等,我就将这个数放进一个新创建的数组中,最后再返回它。 class Solution { public: vector<int> findDuplicates(vector<int>& nums) { vector<int> res; sort(nums.begin(), nums.end()); const int原创 2021-01-12 14:57:39 · 83 阅读 · 0 评论 -
LeetCode 199. Binary Tree Right Side View 做题记录
题目: Given a binary tree, imagine yourself standing on the right side of it, return the values of the nodes you can see ordered from top to bottom. 我用深度优先算法来做的这道题。 /** * Definition for a binary tree node. * struct TreeNode { * int val; * .原创 2021-01-12 14:40:55 · 88 阅读 · 0 评论 -
LeetCode 48.Rotate Image 做题记录
题目: You are given an n x n 2D matrix representing an image, rotate the image by 90 degrees (clockwise). You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotat原创 2021-01-11 13:52:21 · 117 阅读 · 0 评论