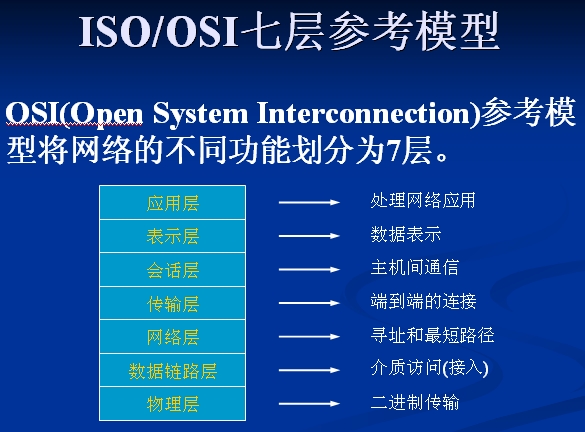
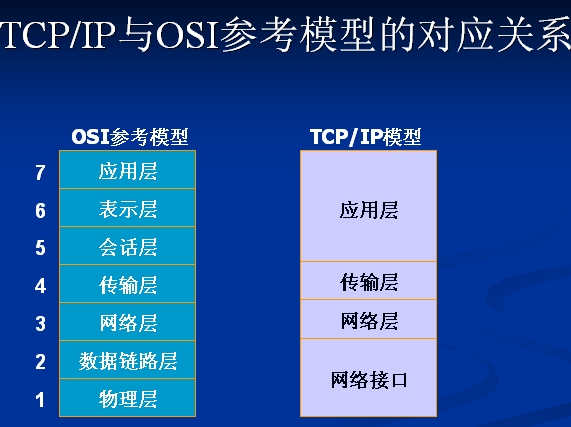
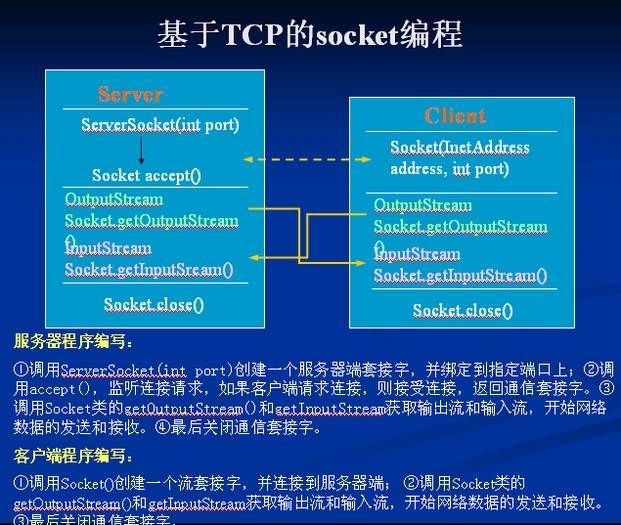
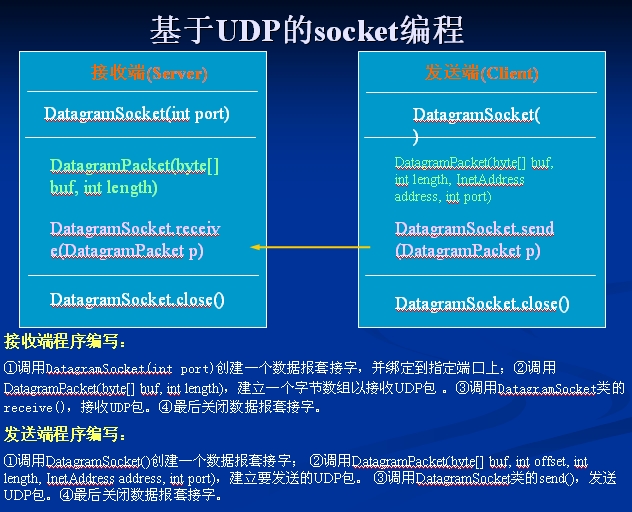
java 代码
- package Lesson10;
- import java.net.*;
- import java.io.*;
- public class Lesson10 {
- public static void main(String[] args) {
- if(args.length>0)
- server();
- else client();
- }
- public static void server() {
- try {
- ServerSocket ss = new ServerSocket(6001);
- Socket s = ss.accept();
- OutputStream os = s.getOutputStream();
- InputStream is = s.getInputStream();
- os.write("welcome!".getBytes());
- byte[] buf = new byte[100];
- int len = is.read(buf);//输入流写到buf中
- System.out.println(new String(buf,0,len));
- os.close();
- is.close();
- s.close();
- ss.close();
- }
- catch (Exception e) {
- e.printStackTrace();
- }
- }
- public static void client() {
- try{
- Socket s = new Socket(InetAddress.getByName(null),6001);
- InputStream is = s.getInputStream();
- OutputStream os = s.getOutputStream();
- byte[] buf = new byte[100];
- int len = is.read(buf);
- System.out.println(new String(buf,0,len));
- os.write("zhangsan online".getBytes());
- is.close();
- os.close();
- s.close();
- }
- catch(Exception e) {
- e.printStackTrace();
- }
- }
- }
- //运行参数配置:Run -> Run... ->选择好Project 和 Main Class -> Name 为server
- //(X)Arguments 随便输入一个字符(串) -> Name :client ->运行server 运行client
java 代码
- package lesson10;
- import java.net.*;
- import java.io.*;
- public class Lesson10 extends Thread
- {
- public static void main(String[] args)
- {
- if(args.length>0)
- recv();
- else
- send();
- }
- public static void recv()
- {
- try {
- DatagramSocket ds=new DatagramSocket(6000);
- byte[] buf=new byte[100];
- DatagramPacket dp=new DatagramPacket(buf,100);
- ds.receive(dp);
- System.out.println(new String(buf,0,dp.getLength()));
- String str="Welcome you!";
- DatagramPacket dpSend=new DatagramPacket(str.getBytes(),str.length(),
- dp.getAddress(),dp.getPort());
- ds.send(dpSend);
- ds.close();
- }
- catch (Exception ex) {
- ex.printStackTrace();
- }
- }
- public static void send()
- {
- try {
- DatagramSocket ds=new DatagramSocket();
- String str="Hello,this is zhangsan";
- DatagramPacket dp=new DatagramPacket(str.getBytes(),str.length(),
- InetAddress.getByName("localhost"),
- 6000);
- ds.send(dp);
- byte[] buf=new byte[100];
- DatagramPacket dpRecv=new DatagramPacket(buf,100);
- ds.receive(dpRecv);
- System.out.println(new String(buf,0,dpRecv.getLength()));
- ds.close();
- }
- catch (Exception ex) {
- ex.printStackTrace();
- }
- }
java 代码
- package Lesson10;
- import java.net.*;
- import javax.swing.*;
- import java.awt.event.*;
- import java.io.*;
- public class Download
- {
- public static void main(String[] args)
- {
- JFrame jf=new JFrame("维新下载程序");
- jf.setSize(600,400);
- jf.setLocation(100,100);
- JPanel p=new JPanel();
- JLabel l=new JLabel("Please input URL:");
- final JTextField tf=new JTextField(30);
- p.add(l);
- p.add(tf);
- jf.getContentPane().add(p,"North");
- final JTextArea ta=new JTextArea();
- jf.getContentPane().add(ta,"Center");
- JButton btn=new JButton("Download");
- jf.getContentPane().add(btn,"South");
- btn.addActionListener(new ActionListener() {
- public void actionPerformed(ActionEvent e) {
- String str=tf.getText();
- try {
- URL url=new URL(str);
- URLConnection urlConn=url.openConnection();
- String line=System.getProperty("line.separator");//行分隔符
- ta.append("Host: "+url.getHost());
- ta.append(line);
- ta.append("Port: "+url.getDefaultPort());
- ta.append(line);
- ta.append("ContentType: "+urlConn.getContentType());
- ta.append(line);
- ta.append("ContentLength: "+urlConn.getContentLength());
- InputStream is=urlConn.getInputStream();
- //InputStreamReader isr=new InputStreamReader(is);//字节流转化为字符流
- //BufferedReader br=new BufferedReader(isr);//字符流方式读取
- FileOutputStream fos=new FileOutputStream("1.html");
- //String strLine;
- //while((strLine=br.readLine())!=null)
- int data;
- while((data=is.read())!=-1)//以字节流的方式读取
- {
- // fos.write(strLine.getBytes());//字符流方式读取
- // fos.write(line.getBytes());
- fos.write(data);
- }
- //br.close();
- is.close();
- fos.close();
- }
- catch (Exception ex) {
- ex.printStackTrace();
- }
- }
- });
- jf.addWindowListener(new WindowAdapter() {
- public void windowClosing(WindowEvent e) {
- System.exit(0);
- }
- });
- jf.setVisible(true);
- }
- }