桥接模式:将抽象部分与它的实现部分分离,使它们都可以独立地变化。使得每种实现的变化不会影响其他实现,从而达到应对变化的目的。【组合】
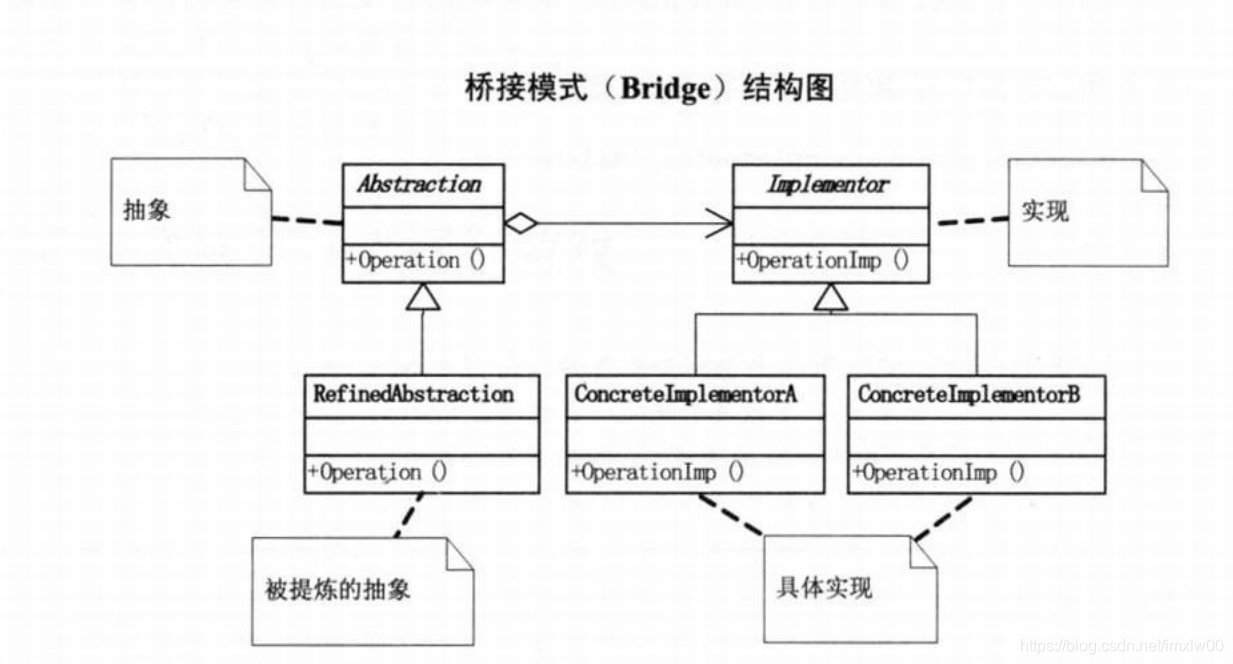
public abstract class Abstraction {
protected Implementor implementor;
private String name;
public Abstraction(String name) {
this.setName(name);
}
public void setImplementor(Implementor implementor) {
this.implementor = implementor;
}
public void operation() {
System.out.print("Abstraction-" + this.getName() + ": ");
implementor.operation();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
class AbstractionA extends Abstraction {
public AbstractionA(String name) {
super(name);
}
@Override
public void operation() {
super.operation();
}
}
class AbstractionB extends Abstraction {
public AbstractionB(String name) {
super(name);
}
@Override
public void operation() {
super.operation();
}
}
public abstract class Implementor {
public abstract void operation();
}
class ConcreteImplemtorA extends Implementor {
@Override
public void operation() {
System.out.println("ConcreteImplemtorA的方法执行");
}
}
class ConcreteImplemtorB extends Implementor {
@Override
public void operation() {
System.out.println("ConcreteImplemtorB的方法执行");
}
}
public class BridgeClient {
public static void main(String[] args) {
Abstraction a = new AbstractionA("A");
a.setImplementor(new ConcreteImplemtorA());
a.operation();
a.setImplementor(new ConcreteImplemtorB());
a.operation();
Abstraction b = new AbstractionB("B");
b.setImplementor(new ConcreteImplemtorA());
b.operation();
b.setImplementor(new ConcreteImplemtorB());
b.operation();
}
}
案例
public abstract class PhoneBrand {
protected PhoneSoft soft;
public void setPhoneSoft(PhoneSoft soft) {
this.soft = soft;
}
public abstract void run();
}
public class PhoneBrandM extends PhoneBrand {
@Override
public void run() {
soft.run();
}
}
public class PhoneBrandN extends PhoneBrand {
@Override
public void run() {
soft.run();
}
}
public abstract class PhoneSoft {
public abstract void run();
}
public class PhoneGame extends PhoneSoft {
@Override
public void run() {
System.out.println("运行手机游戏");
}
}
public class PhoneAddressList extends PhoneSoft {
@Override
public void run() {
System.out.println("运行手机通讯录");
}
}
public class Client {
public static void main(String[] args) {
PhoneBrand phoneBrand;
phoneBrand = new PhoneBrandN();
phoneBrand.setPhoneSoft(new PhoneGame());
phoneBrand.run();
phoneBrand.setPhoneSoft(new PhoneAddressList());
phoneBrand.run();
phoneBrand = new PhoneBrandM();
phoneBrand.setPhoneSoft(new PhoneGame());
phoneBrand.run();
phoneBrand.setPhoneSoft(new PhoneAddressList());
phoneBrand.run();
}
}