范例1:实现字符串的替换
public class Demo {
public static void main(String[] args) {
String str = "woighasksgoi3423siofa5+5+4asdga55sd3488";
String regex = "[^a-z]";
System.out.println(str.replaceAll(regex, ""));
}
}
===========分割线===========
范例2:字符串拆分
public class Demo {
public static void main(String[] args) {
String str = "woighasksgoi3423siofa5+5+4asdga55sd3488";
String regex = "\\d+";
String result[] = str.split(regex);
for (int i = 0; i < result.length; i++) {
System.out.println(result[i]);
}
}
}
===========分割线===========
范例3:判断字符串是否是数字,如果是则转换为double类型
public class Demo {
public static void main(String[] args) {
String str = "10.2";
String regex = "\\d+(\\.\\d+)";
System.out.println(str.matches(regex));// 判断
if (str.matches(regex)) {
System.out.println(Double.parseDouble(str));// 转换为double类型
}
}
}
===========分割线===========
范例4:判断IP地址
public class Demo {
public static void main(String[] args) {
String str = "192.168.1.3";
String regex = "\\d{1,3}\\.\\d{1,3}\\.\\d{1,3}\\.\\d{1,3}";
System.out.println("IP地址是否正确:" + str.matches(regex));
}
}
===========分割线===========
范例5:判断是否日期格式,如果是日期格式转换为Date类型
import java.text.SimpleDateFormat;
import java.util.Date;
public class Demo {
public static void main(String[] args) throws Exception {
String str = "2018-05-18";
String regex = "\\d{4}-\\d{2}-\\d{2}";
if (str.matches(regex)) {
Date date = new SimpleDateFormat("yyyy-MM-dd").parse(str);
System.out.println(date);
}
}
}
===========分割线===========
范例6:判断电话格式
·格式1:8964852的验证格式:(\\d{7,8})
·格式2:020-8964852的验证格式:(\\d{3,4}-)?\\d{7,8}
·格式3:(020)-8964852的验证格式:((\\d{3,4}-)|(\\(\\d{3,4}\\) ))?\\d{7,8}
import java.util.Scanner;
public class Demo {
public static void main(String[] args) throws Exception {
Scanner in = new Scanner(System.in);
System.out.print("请输入电话号码:");
String str = in.next();
String regex = "((\\d{3,4}-)|(\\(\\d{3,4}\\)-))?\\d{7,8}";
if (str.matches(regex)) {
System.out.println("电话号码格式正确!");
}
}
}
===========分割线===========
范例7:验证email地址
public class Demo {
public static void main(String[] args) throws Exception {
boolean flag = true;
Scanner in = new Scanner(System.in);
String result;
String regex = "\\w+@\\w+\\.\\w+";
System.out.println("请输入email:");
while (flag) {
result = in.next();
if (result.matches(regex)) {
System.out.println("格式正确!");
flag = false;
} else {
System.out.println("格式不正确!请重新输入:");
}
}
}
}
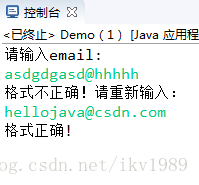