List子接口是Collection常用的子接口,此接口对Collection接口进行了一些功能上的扩充。List接口下常用方法:
No. | 方法名称 | 类型 | 描述 |
1 | public E get(int index) | 普通 | 取得索引编号内容 |
2 | public E set(int index,E element) | 普通 | 修改指定索引编号内容 |
3 | public ListIterator<E> listIterator() | 普通 | 为ListIterator接口实例化 |
List属于接口,所以要使用此接口方法,必须使用其子类ArrayList实现操作。
新的子类:ArrayList
ArrayList类是List接口最为常用的一个子类。
范例1:List基本操作
import java.util.ArrayList;
import java.util.List;
public class Demo {
public static void main(String[] args) {
List<String> all = new ArrayList<>();
System.out.println("长度:" + all.size() + ",是否为空:" + all.isEmpty() + "。");
all.add("Hello");
all.add("Hello");// 重复
all.add("World");
System.out.println("长度:" + all.size() + ",是否为空:" + all.isEmpty() + "。");
for (int i = 0; i < all.size(); i++) {
String str = all.get(i);// 取得索引数据
System.out.println(str);
}
}
}
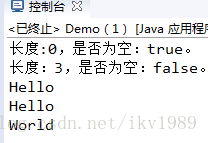
============分割线============
范例2:为Collection接口实例化
·ArrayList是List接口子类,而List又是Collection子接口,自然可以通过ArrayList为Collection接口实例化
import java.util.ArrayList;
import java.util.Collection;
public class Demo {
public static void main(String[] args) {
Collection<String> all = new ArrayList<>();
System.out.println("长度:" + all.size() + ",是否为空:" + all.isEmpty() + "。");
all.add("Hello");
all.add("Hello");// 重复
all.add("World");
System.out.println("长度:" + all.size() + ",是否为空:" + all.isEmpty() + "。");
Object[] obj = all.toArray();// 对象数组
for (int i = 0; i < obj.length; i++) {
System.out.println(obj[i]);
}
}
}
============分割线============
旧子类:Vector
范例:使用Vector
import java.util.List;
import java.util.Vector;
public class Demo {
public static void main(String[] args) throws Exception {
List<String> all = new Vector<>();
System.out.println("元素数量:" + all.size() + ",是否为空链表:" + all.isEmpty());
all.add("Hello");
all.add("world");
all.add("world");
System.out.println("元素数量:" + all.size() + ",是否为空链表:" + all.isEmpty());
for (int i = 0; i < all.size(); i++) {
System.out.println(all.get(i));
}
}
}
No. | 区别 | ArrayList | Vector |
1 | 推出时间 | JDK1.2(new) | JDK1.0(old) |
2 | 性能 | 异步处理 | 同步处理 |
3 | 数据安全 | 非线程安全 | 线程安全 |
4 | 输出 | Iterator,ListIterator,foreach | Iterator,ListIterator,foreach,Enumeration |