一个简单的基于C/S架构的模拟图片文件上传DEMO
首先要明白上传文件的I/O流的详细过程
如图所示
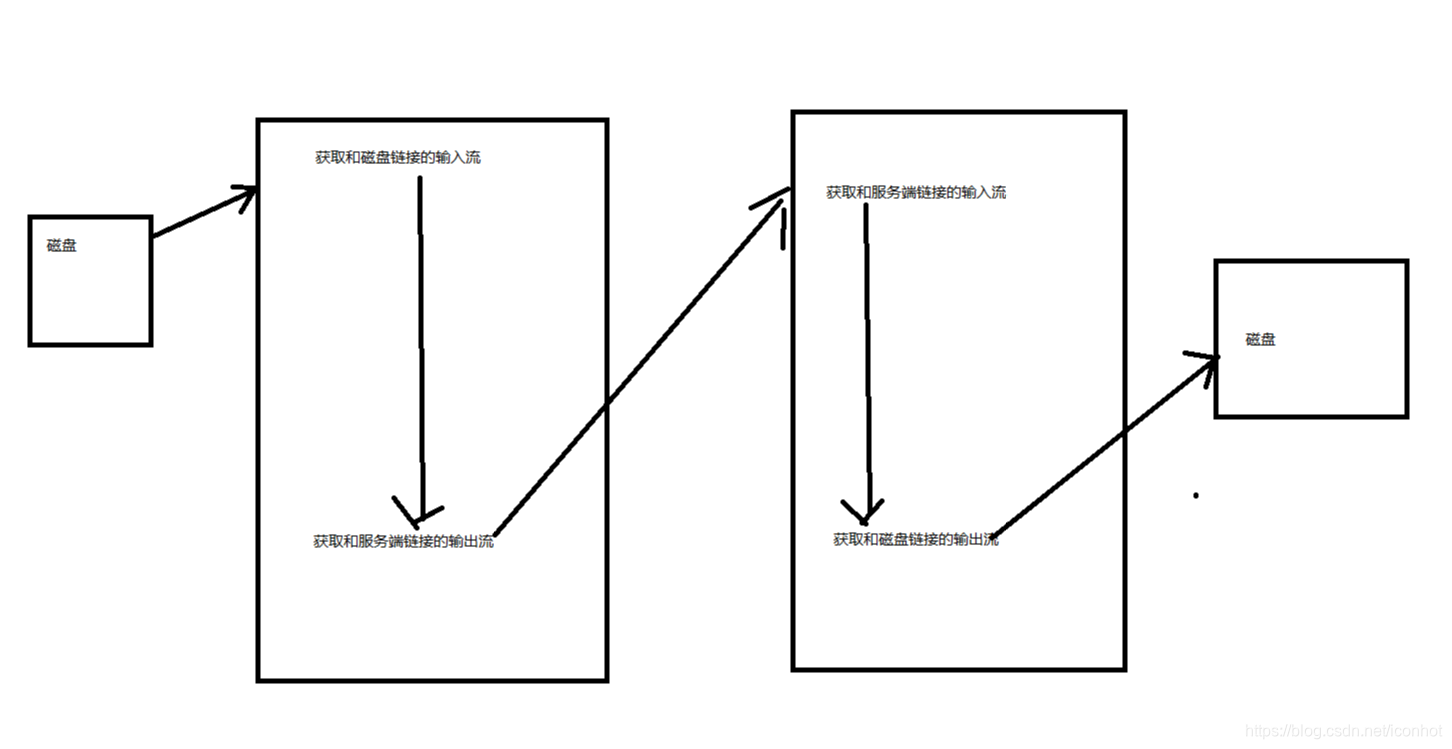
服务端代码
public class Server {
public static void main(String[] args) throws Exception {
ServerSocket ss = new ServerSocket(8888);
while (true) {
Socket socket = ss.accept();
new Thread(() -> {
try {
BufferedInputStream bis = new BufferedInputStream(socket.getInputStream());
String name = "xxxx\\" + System.currentTimeMillis() + ".jpg";
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(name));
int c;
byte[] b = new byte[1024 * 8];
while ((c = bis.read(b)) != -1) {
bos.write(b, 0, c);
}
OutputStream os = socket.getOutputStream();
os.write("上传成功".getBytes());
bos.close();
bis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}).start();
}
}
}
public class Client {
public static void main(String[] args) throws Exception {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("day18\\1.jpg"));
Socket socket = new Socket("127.0.0.1", 8888);
BufferedOutputStream bos = new BufferedOutputStream(socket.getOutputStream());
byte[] b = new byte[1024 * 8];
int len;
while ((len = bis.read(b)) != -1) {
bos.write(b, 0, len);
}
bos.flush();
socket.shutdownOutput();
InputStream is = socket.getInputStream();
System.out.println(new String(is.readAllBytes()));
is.close();
socket.close();
bis.close();
}
}
- 问题:
单线程的情况下上传大文件, 一直接收, 下一次客户端不能连接
因此这里使用多线程
的方式来解决此问题。