第一题:P1403 [AHOI2005]约数研究 - 洛谷 | 计算机科学教育新生态 (luogu.com.cn)
package 蓝桥算法训练__普及组.Day12;
import java.io.*;
/**
* @author snippet
* @data 2023-02-16
* P1403 [AHOI2005]约数研究 - 洛谷 | 计算机科学教育新生态 (luogu.com.cn)
*/
public class T1 {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static StreamTokenizer st = new StreamTokenizer(br);
static PrintWriter pw = new PrintWriter(new OutputStreamWriter(System.out));
static int n,ans;
static int[] a = new int[1000100];
// 筛法:j能被i整除时 a[j]++
static void solve() {
for (int i = 1; i <= n; i++) {
for (int j = i; j <= n; j+=i) {
a[j]++;
}
ans += a[i];
}
}
public static void main(String[] args) throws IOException {
n = nextInt();
solve();
pw.println(ans);
pw.flush();
}
static int nextInt() throws IOException {
st.nextToken();
return (int)st.nval;
}
}
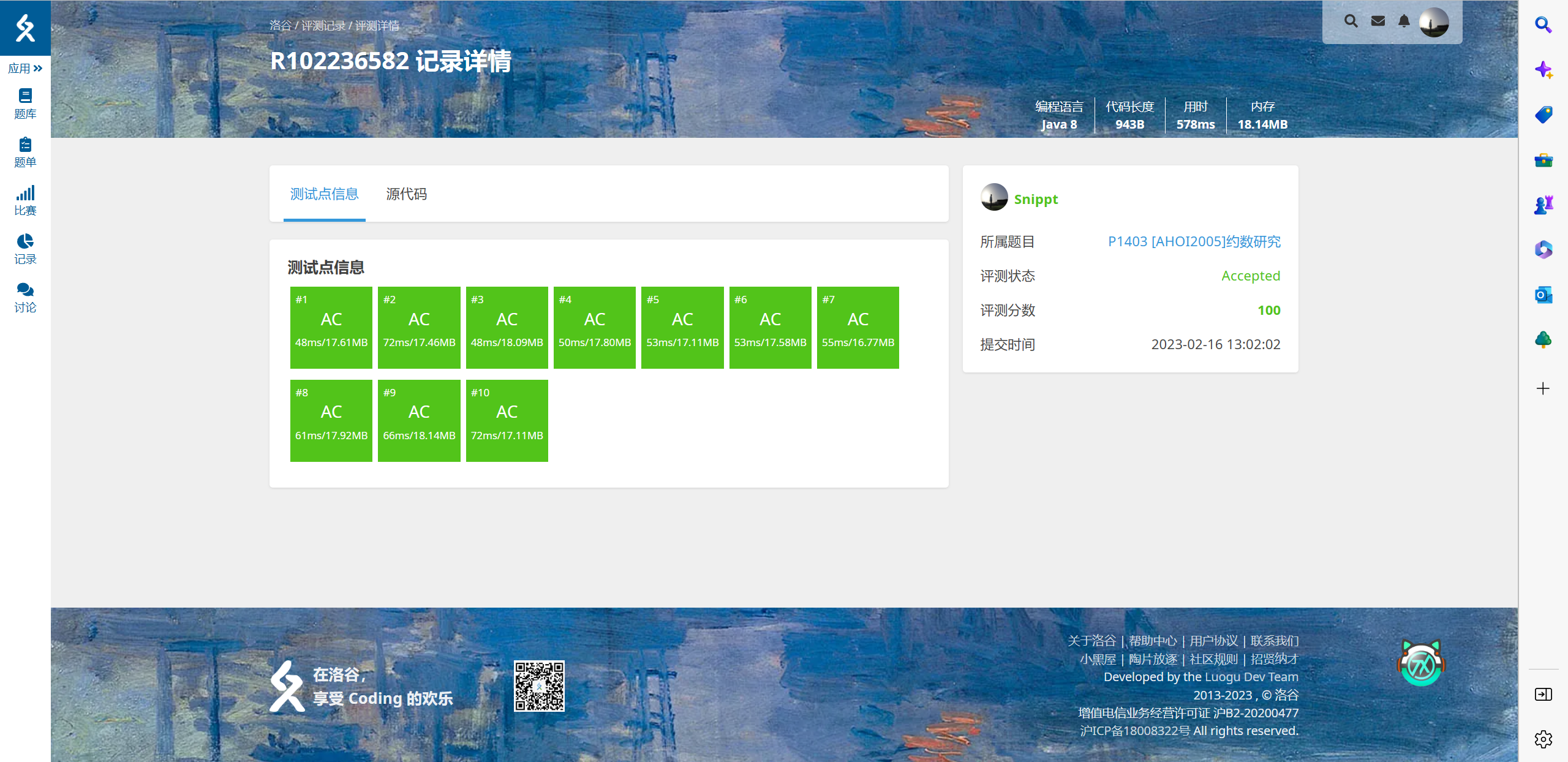
第二题:P1842 [USACO05NOV]奶牛玩杂技 - 洛谷 | 计算机科学教育新生态 (luogu.com.cn)
package 蓝桥算法训练__普及组.Day12;
import java.io.*;
import java.util.*;
/**
* @author snippet
* @data 2023-02-16
* P1842 [USACO05NOV]奶牛玩杂技 - 洛谷 | 计算机科学教育新生态 (luogu.com.cn)
*/
// 贪心
public class T2 {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static StreamTokenizer st = new StreamTokenizer(br);
static PrintWriter pw = new PrintWriter(new OutputStreamWriter(System.out));
static int n,sum,ans = (int)(-1e9);// n表示牛的个数 sum表示前面所有牛的体重 ans表示总压扁指数(所有压扁指数的最大压扁指数)
static int[][] arr;// 二维数组arr的列 第一个位置存牛的重量 第二个位置存牛的力量 第三个位置存牛的重量+力量
static ArrayList<ArrayList<Integer>> list = new ArrayList<>();
public static void main(String[] args) throws IOException {
n = nextInt();
arr = new int[n][3];
for (int i = 0; i < n; i++) {
arr[i][0] = nextInt();
arr[i][1] = nextInt();
arr[i][2] = arr[i][0]+arr[i][1];
}
// 排序按牛的重量+力量 从小到大排序
Arrays.sort(arr, ((a,b)-> {
return a[2]-b[2];
}));
for (int i = 0; i < n; i++) {
ans = Math.max(ans, sum-arr[i][1]);
sum += arr[i][0];
}
pw.println(ans);
pw.flush();
}
static int nextInt() throws IOException {
st.nextToken();
return (int)st.nval;
}
}
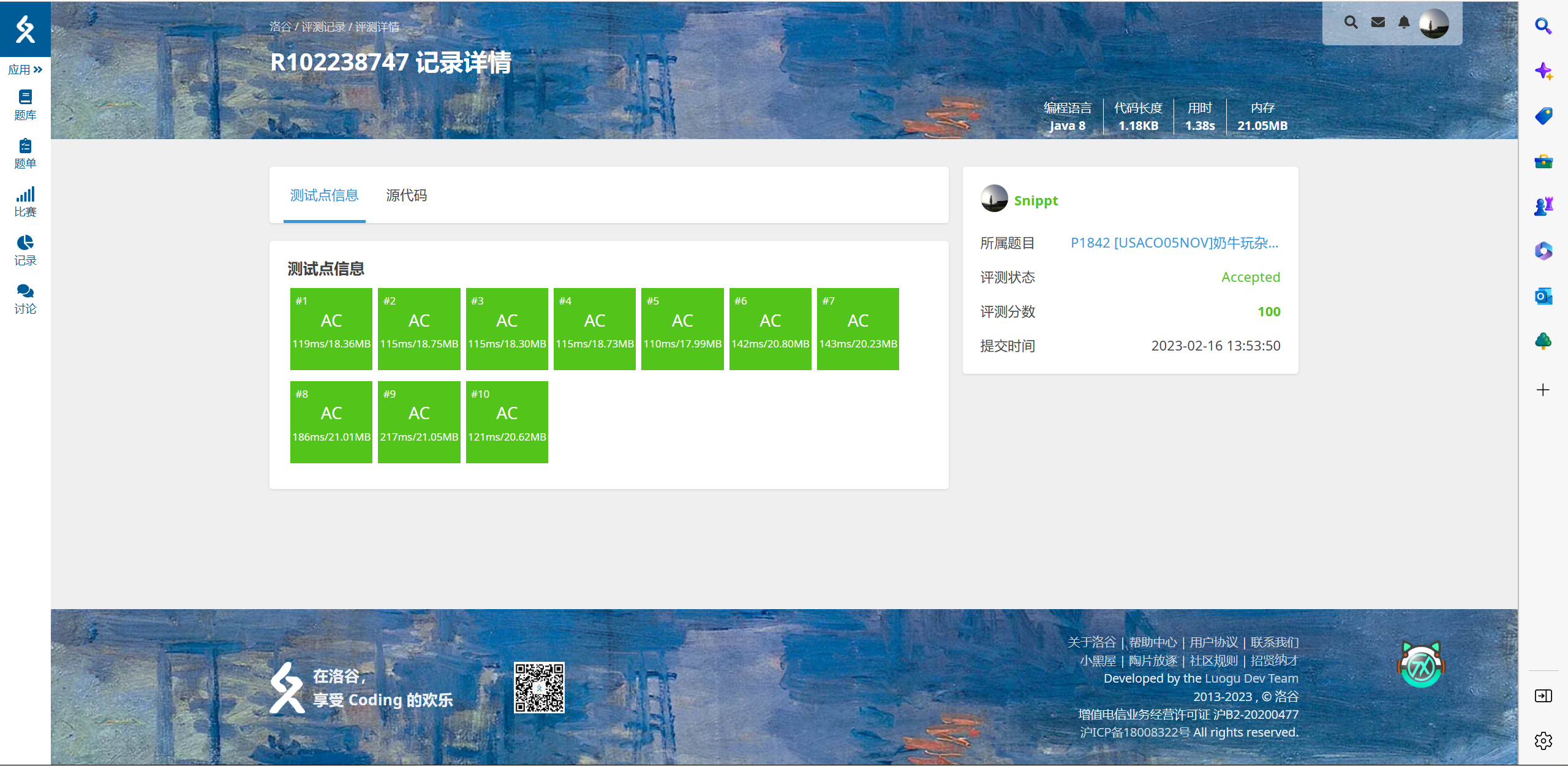
第三题:P1507 NASA的食物计划 - 洛谷 | 计算机科学教育新生态 (luogu.com.cn)
package 蓝桥算法训练__普及组.Day12;
import java.io.*;
/**
* @author snippet
* @data 2023-02-16
* P1507 NASA的食物计划 - 洛谷 | 计算机科学教育新生态 (luogu.com.cn)
*/
// 01背包
public class T3 {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static StreamTokenizer st = new StreamTokenizer(br);
static PrintWriter pw = new PrintWriter(new OutputStreamWriter(System.out));
static int n,hm,tm;// n表示食物的个数 hm表示最大体积 tm表示最大质量
static int[] h = new int[51];// 数组h表示每个食物的体积
static int[] t = new int[51];// 数组t表示每个食物的质量
static int[] k = new int[51];// 数组k表示每个食物的卡路里
static int[][] dp = new int[410][410];// 二维数组dp表示食用 体积为h 质量为t的最大卡路里的值
public static void main(String[] args) throws IOException {
hm = nextInt();
tm = nextInt();
n = nextInt();
for (int i = 1; i <= n; i++) {
h[i] = nextInt();
t[i] = nextInt();
k[i] = nextInt();
}
// 01背包 每个食物只使用一次
for (int i = 1; i <= n; i++) {
for (int j = hm; j >= h[i]; j--) {
for (int l = tm; l >= t[i]; l--) {
dp[j][l] = Math.max(dp[j][l], dp[j-h[i]][l-t[i]]+k[i]);
}
}
}
pw.println(dp[hm][tm]);
pw.flush();
}
static int nextInt() throws IOException {
st.nextToken();
return (int)st.nval;
}
}
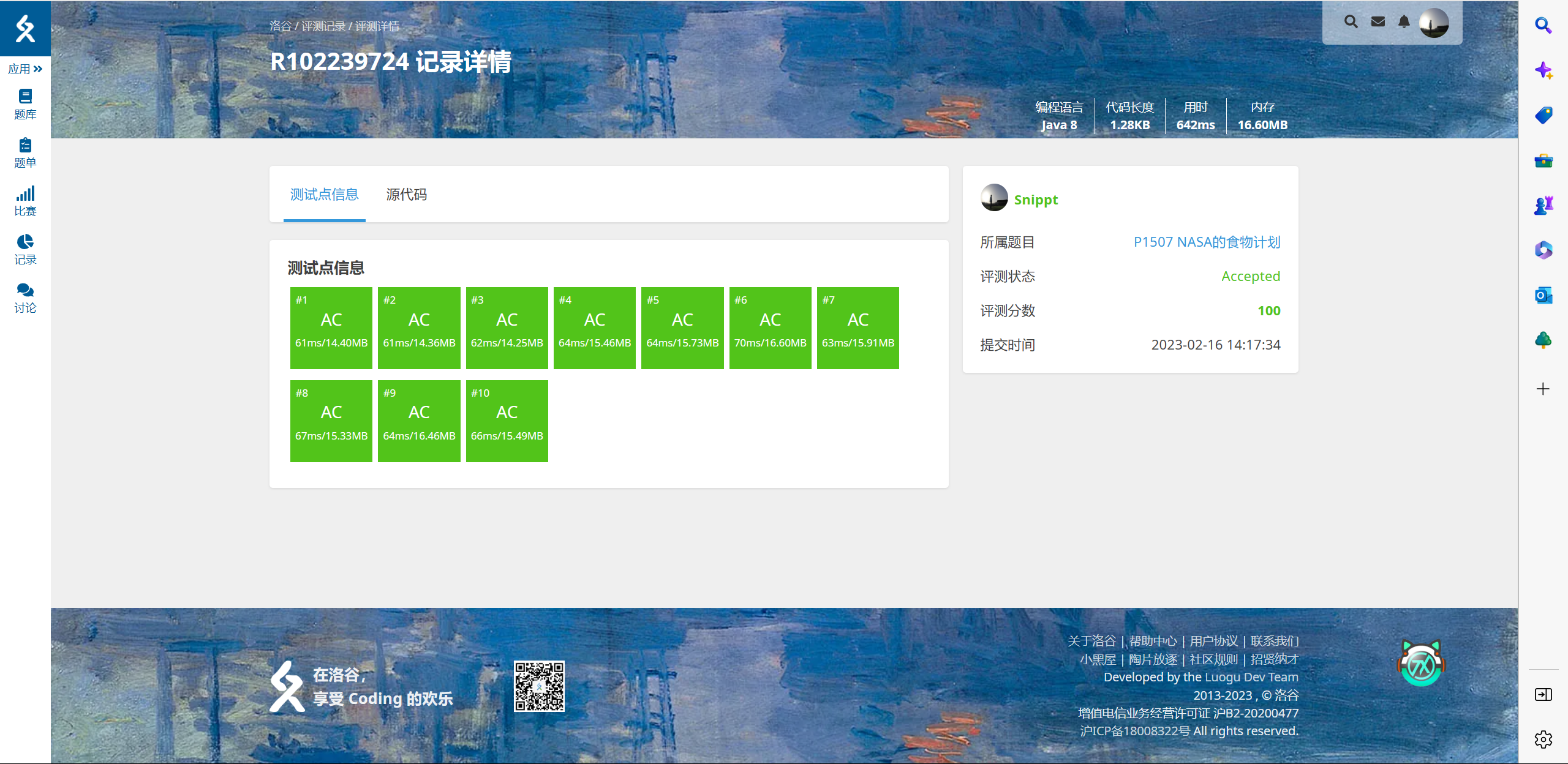