适配器
//An highlighted block
class My_Print:public binary_function<int,int,void>
{
public:
void operator()(int v,int val) const
{
cout <<"第一个参数是:" << v << " 第二个参数是:" << val << "两个数的和是:" << v + val << endl;
}
};
void test1()
{
vector<int> v;
for (int i =0;i<10;i++)
{
v.push_back(i);
}
cout << "请输入起始值:" << endl;
int num;
cin >> num;
for_each(v.begin(),v.end(),bind2nd( My_Print(),num ));
cout << "-------------------------------" << endl;
for_each(v.begin(),v.end(),bind1st( My_Print(),num ));
}
//第一步 绑定 数据 bind2nd 绑定第二个参数 bind1st 绑定第一个参数
//继承类 binary_function<参数1,参数2,返回值类型>
//加const修饰 operator()
//An highlighted block
class greaterThan5 : public unary_function<int,bool>
{
public:
bool operator()(int a) const
{
return a >5;
}
};
//取反配置器
void test2()
{
//一元取反
vector<int>v;
for (int i =0;i<10;i++)
{
v.push_back(i);
}
//查找大于5的数
vector<int>::iterator pos = find_if(v.begin(),v.end(),greaterThan5());
if(pos != v.end())
{
cout << "大于5的数字为:" << *pos << endl;
}
else
{
cout << "没有找到" << endl;
}
//查找小于5的数
pos = find_if(v.begin(),v.end(),not1(greaterThan5()));
//pos = find_if(v.begin(),v.end(),not1(bind2nd(greater<int>(),5)));
if(pos != v.end())
{
cout << "小于5的数字为:" << *pos << endl;
}
else
{
cout << "没有找到" << endl;
}
}
//一元取反适配器 not1 二元 not2 not2(less<int>()) 相当于greater<int>()
//继承unary_function<参数1,返回值类型>
//const 修饰operator()
//An highlighted block
//函数指针适配器
void my_print(int v , int val)
{
cout << v + val<< endl;
}
void test3()
{
vector<int>v;
for (int i =0;i<10;i++)
{
v.push_back(i);
}
//for_each(v.begin(),v.end(),my_print);
//将函数指针 适配为 函数对象
//ptr_fun
for_each(v.begin(),v.end(),bind2nd(ptr_fun(my_print) ,100));
}
//An highlighted block
//成员函数适配器
//如果容器中存放的是对象指针,那么用mem_fun
//如果容器中存放的是对象实体,那么用mem_fun_ref
class Person
{
public:
Person(string name,int age)
{
this ->_name = name;
this ->_age = age;
}
void showPerson()
{
cout << "姓名:" << this->_name << " 年龄:" << this->_age<< endl;
}
void plusAge()
{
this ->_age = this ->_age + 100;
}
string _name;
int _age;
};
void my_print_person(Person p)
{
cout << "姓名:" << p._name << " 年龄:" << p._age << endl;
}
void test4()
{
vector<Person> v;
Person p1("lilei",19);
Person p2("hanmeimei",29);
Person p3("tom",17);
Person p4("bob",66);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
for_each(v.begin(),v.end(),my_print_person);
//成员函数适配器
//mem_fun_ref
cout << "成员函数中:" << endl;
for_each(v.begin(),v.end(),mem_fun_ref(&Person::showPerson));
cout << "---------------------------------------------" << endl;
for_each(v.begin(),v.end(),mem_fun_ref(&Person::plusAge));
for_each(v.begin(),v.end(),mem_fun_ref(&Person::showPerson));
}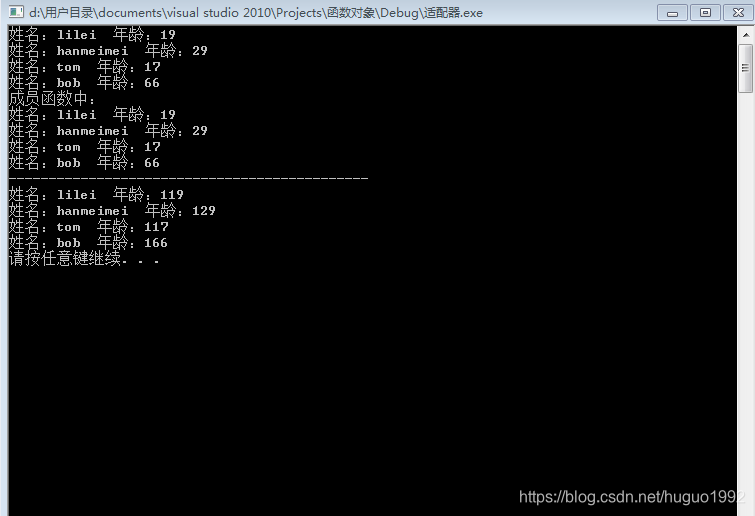