目录
CDC::DrawText
语法
参数
返回值
例子:
多行文字的竖直居中
获取多行文本(超过width后自动换行)的高度
附录
CDC::DrawText
调用该成员函数的格式在给定矩形的文本。 若要指定附加格式设置选项,请使用 CDC::DrawTextEx。
语法
复制
virtual int DrawText(
LPCTSTR lpszString,
int nCount,
LPRECT lpRect,
UINT nFormat
);
int DrawText(
const CString& str,
LPRECT lpRect,
UINT nFormat
);
参数
-
lpszString
指向要绘制的字符串。 如果 nCount 是–必须对停止点1,字符串。
-
nCount
在字符串指定字符数。 如果 nCount 为– 1,则 lpszString 假定为较长的指针到一个Null终止的字符串,并 DrawText 自动计算字符数。
-
lpRect
指向 RECT 包含矩形的结构或 CRect 对象(以逻辑坐标)文本会进行格式设置。
-
str
包含要绘制的指定字符的 CString 对象。
-
nFormat
指定将该文本的格式设置方法。 它可以是 uFormat 参数描述的值的任意组合。DrawText 在 Windows SDK。 (请按位组合使用或运算符):
备注
某些 uFormat 标志组合可能会导致该传递的字符串进行修改。 使用 DT_MODIFYSTRING 和 DT_END_ELLIPSIS 或 DT_PATH_ELLIPSIS 可导致该字符串被修改,导致断言在 CString 重写。 值 DT_CALCRECT、 DT_EXTERNALLEADING、 DT_INTERNAL、 DT_NOCLIP和 DT_NOPREFIX 不能与 DT_TABSTOP 值。
返回值
文本的高度,如果函数运行成功。
例子:
CString str = L"我是来自非洲的姑娘。心中向往神秘的东方,背起行囊寻找梦想,那是龙的故乡。这里的人纯朴善良, 淡淡微笑挂脸庞。";
CRect temp(0, 0, rect.Width(), 0);
// int h1 = pDC->DrawText(str, temp, DT_CALCRECT | DT_WORDBREAK | DT_EDITCONTROL);//144 文本折行后的文本高度
// int h2 = pDC->DrawText(str, temp, DT_CALCRECT | DT_WORDBREAK);//144
// int h3 = pDC->DrawText(str, temp, DT_CALCRECT | DT_EDITCONTROL);//16 单行文本的高度
// int h4 = pDC->DrawText(str, temp, DT_CALCRECT);//16
多行文字的竖直居中
//多行文字的竖直居中
// 思路:根据显示中心,重新计算要求的显示范围
// 具体方法:
// ======================================
// 把str内容显示到客户区的中间,但是每行宽度限定为200,让其自动换行
void CtestDialogDlg::test(CDC* pDC)
{
CFont ft;
ft.CreatePointFont(120,L"楷体"); // 大小与实际大小相差10倍
CFont* pOldFont = pDC->SelectObject(&ft);
CRect clientRect;
GetClientRect(clientRect); // 获得客户区范围
CRect rect;
rect.left = rect.top = 10;
rect.right = 200;
//rect.bottom = clientRect.bottom; // 限定宽度
rect.bottom = 200;
pDC->SelectStockObject(NULL_BRUSH);
pDC->Rectangle(rect);
CString str = L"我是来自非洲的姑娘。心中向往神秘的东方,背起行囊寻找梦想,那是龙的故乡。这里的人纯朴善良, 淡淡微笑挂脸庞。";
CRect temp(0, 0, rect.Width(), 0);
int height = pDC->DrawText(str, temp, DT_CALCRECT | DT_WORDBREAK | DT_EDITCONTROL); // 获得文本高度 144
rect.DeflateRect(2, (rect.Height() - height) / 2); // 改变rect
CPen p1(PS_DASH, 1, RGB(255, 0, 0));
CPen* pOldPen = pDC->SelectObject(&p1);
pDC->Rectangle(rect);
pDC->SelectObject(pOldPen);
pDC->SetBkMode(TRANSPARENT);
//pDC->DrawText(str, rect, DT_CENTER | DT_EDITCONTROL | DT_WORDBREAK);
pDC->DrawTextW(str, rect, DT_LEFT | DT_EDITCONTROL | DT_WORDBREAK);
pDC->SelectObject(pOldFont);
}
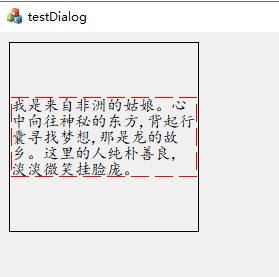
正常使用是这样的:
CRect rc(140, 20, 240, 100);
rc.DeflateRect(4, 4);
CString str = L"我是来自非洲的姑娘。心中向往神秘的东方,背起行囊寻找梦想,那是龙的故乡。这里的人纯朴善良, 淡淡微笑挂脸庞。";
dc.DrawTextW(str, rc, DT_LEFT | DT_EDITCONTROL | DT_WORDBREAK);
若rect的大小不能放下该字符串,则只显示能显示的部分。如图
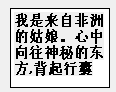
若rect能放的下,就显示全了。故最好先计算一下height,构造一个合适的rect,再DrawText。如下:
CRect rc(140, 20, 240, 100);
CString str = L"我是来自非洲的姑娘。心中向往神秘的东方,背起行囊寻找梦想,那是龙的故乡。这里的人纯朴善良, 淡淡微笑挂脸庞。";
int height = dc.DrawText(str, CRect(0,0,rc.Width(),0), DT_CALCRECT | DT_WORDBREAK | DT_EDITCONTROL);
CRect rc2 = rc;
rc2.bottom = rc2.top + height;
dc.Rectangle(rc2);
dc.DrawTextW(str, rc2, DT_LEFT | DT_EDITCONTROL | DT_WORDBREAK);
获取多行文本(超过width后自动换行)的高度
//通过width获取多行文本(超过width后自动换行)的高度
int GetTextHeightByWidth(CDC* pDC, const CString& strText, int width)
{
CRect temp(0, 0, width, 0);
int height = pDC->DrawText(strText, temp, DT_CALCRECT | DT_WORDBREAK | DT_EDITCONTROL); // 获得文本高度 144
return height;
}
附录
DrawText 中的参数 nFormat 可以取一下的值
The method of formatting the text. This parameter can be one or more of the following values.
表 1
Value | Meaning |
---|
DT_BOTTOM | Justifies the text to the bottom of the rectangle. This value is used only with the DT_SINGLELINE value. |
DT_CALCRECT | Determines the width and height of the rectangle. If there are multiple lines of text, DrawText uses the width of the rectangle pointed to by the lpRect parameter and extends the base of the rectangle to bound the last line of text. If the largest word is wider than the rectangle, the width is expanded. If the text is less than the width of the rectangle, the width is reduced. If there is only one line of text, DrawText modifies the right side of the rectangle so that it bounds the last character in the line. In either case, DrawText returns the height of the formatted text but does not draw the text. |
DT_CENTER | Centers text horizontally in the rectangle. |
DT_EDITCONTROL | Duplicates the text-displaying characteristics of a multiline edit control. Specifically, the average character width is calculated in the same manner as for an edit control, and the function does not display a partially visible last line. |
DT_END_ELLIPSIS | For displayed text, if the end of a string does not fit in the rectangle, it is truncated and ellipses are added. If a word that is not at the end of the string goes beyond the limits of the rectangle, it is truncated without ellipses. The string is not modified unless the DT_MODIFYSTRING flag is specified. Compare with DT_PATH_ELLIPSIS and DT_WORD_ELLIPSIS. |
DT_EXPANDTABS | Expands tab characters. The default number of characters per tab is eight. The DT_WORD_ELLIPSIS, DT_PATH_ELLIPSIS, and DT_END_ELLIPSIS values cannot be used with the DT_EXPANDTABS value. |
DT_EXTERNALLEADING | Includes the font external leading in line height. Normally, external leading is not included in the height of a line of text. |
DT_HIDEPREFIX | Ignores the ampersand (&) prefix character in the text. The letter that follows will not be underlined, but other mnemonic-prefix characters are still processed. Example: input string: "A&bc&&d" normal: "Abc&d" DT_HIDEPREFIX: "Abc&d" Compare with DT_NOPREFIX and DT_PREFIXONLY. |
DT_INTERNAL | Uses the system font to calculate text metrics. |
DT_LEFT | Aligns text to the left. |
DT_MODIFYSTRING | Modifies the specified string to match the displayed text. This value has no effect unless DT_END_ELLIPSIS or DT_PATH_ELLIPSIS is specified. |
DT_NOCLIP | Draws without clipping. DrawText is somewhat faster when DT_NOCLIP is used. |
DT_NOFULLWIDTHCHARBREAK | Prevents a line break at a DBCS (double-wide character string), so that the line breaking rule is equivalent to SBCS strings. For example, this can be used in Korean windows, for more readability of icon labels. This value has no effect unless DT_WORDBREAK is specified. |
DT_NOPREFIX | Turns off processing of prefix characters. Normally, DrawText interprets the mnemonic-prefix character & as a directive to underscore the character that follows, and the mnemonic-prefix characters && as a directive to print a single &. By specifying DT_NOPREFIX, this processing is turned off. For example, Example: input string: "A&bc&&d" normal: "Abc&d" DT_NOPREFIX: "A&bc&&d" Compare with DT_HIDEPREFIX and DT_PREFIXONLY. |
DT_PATH_ELLIPSIS | For displayed text, replaces characters in the middle of the string with ellipses so that the result fits in the specified rectangle. If the string contains backslash (\) characters, DT_PATH_ELLIPSIS preserves as much as possible of the text after the last backslash. The string is not modified unless the DT_MODIFYSTRING flag is specified. Compare with DT_END_ELLIPSIS and DT_WORD_ELLIPSIS. |
DT_PREFIXONLY | Draws only an underline at the position of the character following the ampersand (&) prefix character. Does not draw any other characters in the string. For example, Example: input string: "A&bc&&d"n normal: "Abc&d" DT_PREFIXONLY: " _ " Compare with DT_HIDEPREFIX and DT_NOPREFIX. |
DT_RIGHT | Aligns text to the right. |
DT_RTLREADING | Layout in right-to-left reading order for bidirectional text when the font selected into the hdc is a Hebrew or Arabic font. The default reading order for all text is left-to-right. |
DT_SINGLELINE | Displays text on a single line only. Carriage returns and line feeds do not break the line. |
DT_TABSTOP | Sets tab stops. Bits 15-8 (high-order byte of the low-order word) of the uFormat parameter specify the number of characters for each tab. The default number of characters per tab is eight. The DT_CALCRECT, DT_EXTERNALLEADING, DT_INTERNAL, DT_NOCLIP, and DT_NOPREFIX values cannot be used with the DT_TABSTOP value. |
DT_TOP | Justifies the text to the top of the rectangle. |
DT_VCENTER | Centers text vertically. This value is used only with the DT_SINGLELINE value. |
DT_WORDBREAK | Breaks words. Lines are automatically broken between words if a word would extend past the edge of the rectangle specified by the lpRect parameter. A carriage return-line feed sequence also breaks the line. If this is not specified, output is on one line. |
DT_WORD_ELLIPSIS | Truncates any word that does not fit in the rectangle and adds ellipses. Compare with DT_END_ELLIPSIS and DT_PATH_ELLIPSIS. |