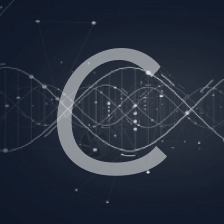
leetcode
hlang8160
这个作者很懒,什么都没留下…
展开
-
155. Min Stack
class MinStack { /** initialize your data structure here. */ private Stack stack=new Stack private Stack minStack=new Stack public MinStack() { } publ原创 2017-10-01 16:36:53 · 223 阅读 · 0 评论 -
70. Climbing Stairs
第一反应,递归求解,貌似很简单。但是不幸,超时[java] view plain copypublic int climbStairs1(int n) { if (n == 1 || n == 2) { return n; } return cli转载 2017-10-01 17:44:51 · 193 阅读 · 0 评论 -
674. Longest Continuous Increasing Subsequence
求最长的连续增长子序列。这是一道简单题,可以用 cnt来记录当前的最长增长子序列,用res来记录最后结果最大的最长增长子序列。class Solution { public int findLengthOfLCIS(int[] nums) { int res=0,cnt=0; for (int i=0; i if(i=原创 2017-10-02 11:50:44 · 166 阅读 · 0 评论 -
33. Search in Rotated Sorted Array
Suppose an array sorted in ascending order is rotated at some pivot unknown to you beforehand.(i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2).You are given a target value to search. If foun原创 2017-10-11 20:44:40 · 194 阅读 · 0 评论 -
234. Palindrome Linked List回文链表
回文是指从前往后读和从后往前读是一样的,这样有两种情况:偶数个:baccab奇数个:bacab因此需要考虑两种情况,找到中心,再反向遍历。而链表中找中心的办法通常是采用快慢指针,快指针走两步,慢指针走一步。(快慢指针还可以用在寻找最小找交叉节点)反向遍历: public ListNode reverseList(ListNode head){ ListNo原创 2017-10-01 10:28:18 · 219 阅读 · 0 评论 -
二叉树的镜像
/**public class TreeNode { int val = 0; TreeNode left = null; TreeNode right = null; public TreeNode(int val) { this.val = val; }}*//*1.先前序遍历这棵树,判断该树是否有叶子节点2.将所有非叶原创 2017-11-17 22:41:47 · 145 阅读 · 0 评论 -
二叉树的层次遍历
实现二叉树的层次遍历1.需要借助队列来保存二叉树中的每个节点。2.借助列表来保存每个节点的值。import java.util.LinkedList;import java.util.ArrayList;Class Solution{public ArrayList PrintFromTopToBottom(TreeNode root){ArraList list=ne原创 2017-11-18 15:34:14 · 150 阅读 · 0 评论 -
2.Add Two Number
1.设置标志位2.如何创建一个新链表来依次保存值创建一个首节点,并保存该首节点。创建下一个节点,将值保存在下一个节点中,并将当前节点指向下一个节点,最后返回首节点的下一节点链表就是我们所需要的链表。ListNode p=new ListNode(0);ListNode dummy=p;p.next=New ListNode(val)//val为计算后我们需要保存的值p=原创 2017-11-30 18:49:24 · 233 阅读 · 0 评论 -
203. Remove Linked List Elements
class Solution { public ListNode removeElements(ListNode head, int val) { ListNode dummy=new ListNode(0); dummy.next=head; ListNode p=dummy; ListNode q=head;原创 2017-10-01 11:52:37 · 156 阅读 · 0 评论 -
441. Arranging Coins
问题:You have a total of n coins that you want to form in a staircase shape, where every k-th row must have exactly k coins.Given n, find the total number of full staircase rows that can b转载 2017-10-08 15:34:15 · 179 阅读 · 0 评论 -
349. Intersection of Two Arrays
1.采用两个hashset 时间复杂度为O(n)class Solution { public int[] intersection(int[] nums1, int[] nums2) { //use two hashset Set set=new HashSet<>(); Set interset=new HashSet<>(原创 2017-10-08 09:39:32 · 186 阅读 · 0 评论 -
leetcode_链表总结
206.Reverse Linked ListReversea singly linked list链表反向;思路:定义一个个新链表的临时节点,依次遍历链表的每个节点,每遍历一个节点就逆置一个节点。原创 2017-09-24 17:40:41 · 376 阅读 · 0 评论 -
基本数据结构――堆的基本概念及其操作
基本数据结构――堆的基本概念及其操作 在我刚听到堆这个名词的时候,我认为它是一堆东西的集合... 但其实吧它是利用完全二叉树的结构来维护一组数据,然后进行相关操作,一般的操作进行一次的时间复杂度在 O(1)~O(logn)之间。 可谓是相当的引领时尚潮流啊(我不信学信息学的你看到log和1的时间复杂度不会激动一下下)!。转载 2017-09-24 19:21:57 · 995 阅读 · 0 评论 -
[LeetCode] Lowest Common Ancestor of a Binary Tree 二叉树的最小共同父节点
[LeetCode] Lowest Common Ancestor of a Binary Tree 二叉树的最小共同父节点 Given a binary tree, find the lowest common ancestor (LCA) of two given nodes in the tree.According to the definition o转载 2017-09-28 09:48:38 · 260 阅读 · 0 评论 -
225. Implement Stack using Queues
使用队列来实现栈class MyStack { Queue queue; /** Initialize your data structure here. */ public MyStack() { this.queue=new LinkedList(); } /** Push element x onto sta原创 2017-10-01 16:04:16 · 240 阅读 · 0 评论 -
50. Pow(x, n)
采用递归的方法,将Pow(x,n),转化为Pow(x,n/2)*Pow(x,n/2)。将时间从O(n)变成O(logn)关键是写出边界条件。并注意定义double类型的tmp=pow(x,n/2).对n的正负以及奇偶做判断。class Solution { public double myPow(double x, int n) { if (n==0) return原创 2017-10-07 10:30:22 · 247 阅读 · 0 评论 -
167. Two Sum II - Input array is sorted
Given an array of integers that is already sorted in ascending order, find two numbers such that they add up to a specific target number.The function twoSum should return indices of the two number原创 2017-10-07 11:05:18 · 232 阅读 · 0 评论 -
367. Valid Perfect Square
Given a positive integer num, write a function which returns True if num is a perfect square else False.Note: Do not use any built-in library function such as sqrt.Example 1:Input: 16Return原创 2017-10-06 17:46:56 · 190 阅读 · 0 评论 -
69 Sqrt(x)_二分查找与牛顿方法
1.采用二分搜索方法注意如何取中点值,并且注意mid要写在while中每次都要更新:mid=lo+(hi-lo)/2,最小值加上最大减最小除2;注意如何返回非正常值,return hiclass Solution { public int mySqrt(int x) { int lo=1; int hi=x; int mid;原创 2017-10-06 17:03:46 · 297 阅读 · 0 评论 -
二分查找_边界值的判定
278. First Bad VersionSuppose you have n versions [1, 2, ..., n] and you want to find out the first bad one, which causes all the following ones to be bad.给定一组数[1,2,...,n]如果一个数为bad则它后续的所有数都为ba原创 2017-10-11 17:35:06 · 1435 阅读 · 0 评论