迭代器
生成器(生成器的第1种实现方式: 列表生成式改为生成器;)
python中yield关键字
函数中如果有yield, 那么调用这个函数的返回值为生成器。
当生成器g调用next方法, 执行函数, 知道遇到yield就停止;
再执行next,从上一次停止的地方继续执行;
函数中遇return直接退出, 不继续执行后面代码;
生成器的第二种方式
生成器: 无缓冲区的生产者消费模型
import time import random def consumer(name): print "%s 准备买粉条...." %(name) while True: kind = yield print "客户[%s] 购买了 [%s] 口味的粉条. " %(name, kind) def producer(name): c1 = consumer("user1") c2 = consumer("user2") c1.next() c2.next() print "[%s]准备制作米线......" %(name) for kind in ['特辣', '三鲜', '香辣']: time.sleep(random.random()) print "[%s]制作了[%s]口味的粉条,卖给用户...." %(name, kind) c1.send(kind) c2.send(kind) c1.close() c2.close() producer("厨师user")
生成器:有缓冲区的生产者消费模型
import time import random cache = [] def consumer(name): print "%s 准备买粉条...." %(name) while True: kind = yield # cache.pop(0) cache.remove(kind) print "客户[%s] 购买了 [%s] 口味的粉条. " %(name, kind) def producer(name): print "[%s]准备制作粉条......" %(name) for kind in ['特辣', '三鲜', '香辣']: time.sleep(random.random()) print "[%s]制作了[%s]口味的粉条,卖给用户...." %(name, kind) cache.append(kind) producer("厨师user") c1 = consumer('user1') c1.next() c1.send('三鲜') print "本店现有的粉条口味: ", for i in cache: print i,
throw 方法:给生成器发送一个异常
迷你聊天机器人
def chat_robot(): res = '' while True: receive = yield res if 'hi' in receive or '你好' in receive: res = "你好" elif 'name' in receive or '姓名' in receive: res = "不告诉你." elif 'age' in receive or '年龄' in receive: res = "就不告诉你." else: res = "我现在想要睡觉了." Chat = chat_robot() next(Chat) while True: send_data = raw_input("请说出想要对人家说的话: ") if send_data == 'q' or send_data == 'quit': print "我要上天去了." break response = Chat.send(send_data) print "Robot>>: %s" %(response) Chat.close()
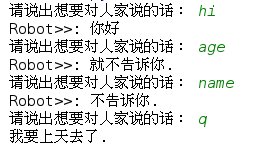