一、先在QQ邮箱中开启POP3/SMTP服务,然后会得到一个授权码,这个邮箱和授权码将用作登陆认证。
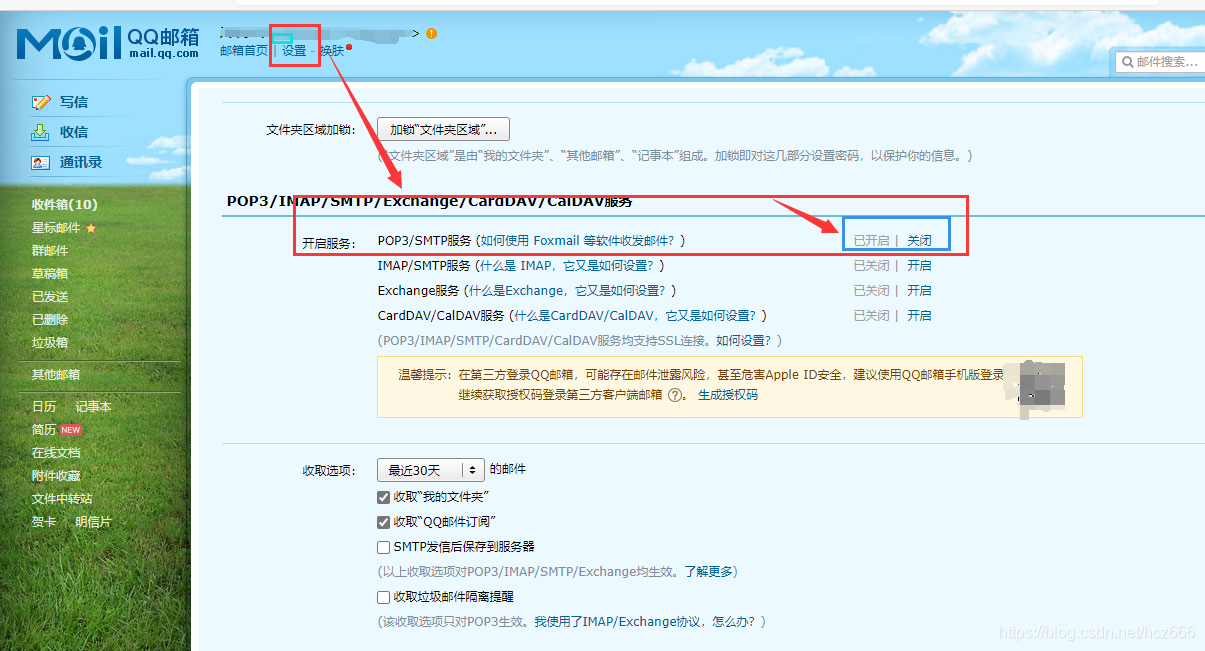
二、导入相关jar包:javax.mail.jar
三、引入配置文件:applicationContext-mail.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://www.springframework.org/schema/beans"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:cache="http://www.springframework.org/schema/cache"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.0.xsd
http://www.springframework.org/schema/cache
http://www.springframework.org/schema/cache/spring-cache-4.0.xsd">
<context:component-scan base-package="com.hcz.util"/>
<bean id="mailSender" class="org.springframework.mail.javamail.JavaMailSenderImpl">
<property name="host" value="smtp.qq.com" />
<property name="port" value="587" />
<property name="username" value="xxxxx@qq.com" />
<property name="password" value="第一步获取到的授权码" />
<property name="protocol" value="smtp" />
<property name="defaultEncoding" value="utf-8" />
<property name="javaMailProperties">
<props>
<prop key="mail.smtp.auth">true</prop>
</props>
</property>
</bean>
</beans>
四、创建工具类
@Component
public class EmailUtil {
@Autowired
private JavaMailSender sender;
public void sendMail(String to,String context,String subject){
SimpleMailMessage msg = new SimpleMailMessage();
msg.setTo(to);
msg.setFrom("xxxxx@qq.com");
msg.setSubject(subject);
msg.setText(context);
sender.send(msg);
System.out.println("发送邮件成功");
}
}
五、在service层调用发送Email的工具类
@Service
public class WzServiceImpl implements WzService {
@Autowired
private CarDao carDao;
@Autowired
private WzDao wzDao;
@Autowired
private RuleDao ruleDao;
@Autowired
private EmailUtil emailUtil;
@Override
public int add(String carno, Integer ruleid, String wzaddress,
Date wztime, String phone, String remark, Integer adminid) {
System.out.println("车牌号:"+carno);
Car car = carDao.selectByNo(carno);
System.out.println("car信息"+car);
if (car == null){
return 0;
}
Rule rule = ruleDao.selectById(ruleid);
System.out.println("rule信息"+rule);
int n = wzDao.insert(car.getId(),rule.getId(),wzaddress,wztime,
rule.getPay(),rule.getScore(),phone,remark, adminid);
System.out.println("插入信息"+n);
emailUtil.sendMail(car.getUserEmail(),car.getNo()+":违章通知","尊敬的"
+car.getUserName()+":你有一笔违章记录,请您及时确认违章内容:"+rule.getName());
System.out.println(car.getUserEmail());
return n;
}
}