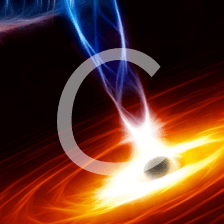
Algorithm
gyafdxis
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
展开
-
插入排序递归版
//递归式的insert_sort#include#includeusing namespace std;void insert(int Array[],int _size_t,int value){ while(Array[_size_t] > value && _size_t >= 0) { Array[_size_t+1] = Array[_size_t];原创 2015-04-15 17:07:32 · 635 阅读 · 0 评论 -
树那里代码总结
//树的非递归三种遍历算法//先序遍历算法void PreOrder(BiTree* bt){ Stack s; init(s) p = bt; while(p&&!IsEmpty(s)) { if(p) //当p不为空的时候一直往左走并且访问 { visit(p->data); push(s,p)原创 2017-03-13 19:19:27 · 547 阅读 · 0 评论 -
图那里的算法总结
//这里整理的为图那里的算法//邻接矩阵和邻接表的图的表示很重要//这里就不说明MAXSIZE就为最大值的默认了typedef struct ArcCell{ VRType adj; //这里是点中的值 InfoType *info; //这里是有关点的信息比如这里可以放原创 2017-03-13 19:20:24 · 406 阅读 · 0 评论 -
排序算法总结
//几个排序//快排 堆排序 希尔排序 归并排序 插入排序,选择排序 //快速排序//以下所有的排序都是从小到大排序//不稳定 时间复杂度O(nlgn)空间复杂度O(1) 最坏时间复杂度O(n^2)当序列基本或者完全趋于有序void qsort(int a[].int low,int high){ int privot = partition(a,low,high);原创 2017-03-13 19:16:50 · 359 阅读 · 0 评论 -
STL的copy真是是做的了极致的效率
inline char* unitialized_copy(const char* first, const char* last, char* result){ std::memmove(result, first, last - first); return result + (last - first); } inline wchar_t* unitialized_copy(c原创 2015-11-30 21:00:46 · 1344 阅读 · 0 评论 -
自己实现的一个list双链表
#ifndef __LIST__#define __LIST__#ifndef __IOSTREAM__#include #endif#ifndef __ALLOCATOR__#include "allocator.h"#endif#ifndef __DEFAULT_ALLOC_TEMPLATE__#include "__default_alloc_templa原创 2015-11-30 20:50:53 · 432 阅读 · 0 评论 -
自己实现的简单heap max_heap和min_heap 还有sort
#ifndef __HEAP__#define __HEAP__#include "allocator.h"#include "__default_alloc_template.h"#include "Vector.h"templateclass heap{public: typedef typename Vector::iterator iterator;//底层用vector原创 2015-11-30 20:47:12 · 624 阅读 · 0 评论 -
Word Pattern
Word PatternGiven a pattern and a string str, find if str follows the same pattern.Here follow means a full match, such that there is a bijection between a letter in pattern and a non-em原创 2015-11-30 22:34:18 · 487 阅读 · 0 评论 -
RBT
前面一篇文章介绍了2-3查找树,可以看到,2-3查找树能保证在插入元素之后能保持树的平衡状态,最坏情况下即所有的子节点都是2-node,树的高度为lgN,从而保证了最坏情况下的时间复杂度。但是2-3树实现起来比较复杂,本文介绍一种简单实现2-3树的数据结构,即红黑树(Red-Black Tree)定义红黑树的主要是像是对2-3查找树进行编码,尤其是对2-3查找树中的3-node转载 2015-11-23 20:25:22 · 463 阅读 · 0 评论 -
STL之allocator
内存池分配器(pool allocator)的主要代码如下(实现详见《STL源码剖析》)[cpp] view plaincopytemplateclass T> class pool_alloc{ public: typedef size_t size_type; typedef ptrdif转载 2015-08-26 21:49:44 · 643 阅读 · 0 评论 -
MemoryPool的简单实现(内存池)
这是可以时常切换的MemoryPool#ifndef MEMORY_POOL#define MEMORY_POOLtemplateclass GD_MemoryPool{ public: enum{m_nBlock = 32}; GD_MemoryPool(size_t nBlock = m_nBlock) { m_Expand(nBlo原创 2015-06-27 20:28:18 · 784 阅读 · 0 评论 -
RBT
Left-Leaning Red-Black Trees, Dagstuhl Workshop on Data Structures, Wadern, Germany, February, 2008,直接下载:http://www.cs.princeton.edu/~rs/talks/LLRB/RedBlack.pdf。本文的github优化版:https://github.com/julycod转载 2015-08-26 21:43:15 · 476 阅读 · 0 评论 -
time
std::chrono::time_point p1, p2, p3; p2 = std::chrono::system_clock::now(); p3 = p2 - std::chrono::minutes(23); char* c_str = new char[100]; std::time_t epoch_time = std::chrono::system_clock::原创 2015-09-04 17:23:05 · 455 阅读 · 0 评论 -
简单的面试题
实现一个算法确定一个字符串中所有的字符串是否都不相同,假设不允许使用额外的数据结构一般的解法是如下:#includeusing namespace std;bool noSame(const char* c_str){bool flat = true;if (*c_str == NULL || *(c_str + 1) == NULL)ret原创 2015-09-03 21:00:38 · 426 阅读 · 0 评论 -
leetcode twoSum
Given an array of integers, return indices of the two numbers such that they add up to a specific target.You may assume that each input would have exactly one solution, and you may not use the sam原创 2018-01-11 11:15:34 · 369 阅读 · 0 评论