编程实现1到N个数的所有排列组合。
如:
n = 3
则得到的序列如下:123, 132, 213, 231, 312, 321
我的实现如下,大家看看如何:
如:
n = 3
则得到的序列如下:123, 132, 213, 231, 312, 321
我的实现如下,大家看看如何:
1
using
System;
2
using
System.Collections.Generic;
3
using
System.Text;
4
5
namespace
ConsoleApplication42
6
{
7
class Program
8
{
9
static void Main(string[] args)
10
{
11
int n = 4;
12
13
CreateList creator = new CreateList(n);
14
15
creator.Creat();
16
}
17
}
18
19
public class CreateList
20
{
21
private struct Num
22
{
23
public int num;
24
public State state;
25
}
26
27
private enum State { LEFT = -1, RIGHT = 1, STAY = 0 };
28
29
private int _n;
30
31
private int _totle;
32
33
private Num[] _nums;
34
35
public CreateList(int n)
36
{
37
if (n < 1)
38
{
39
throw new Exception("N should large than zero.");
40
}
41
42
_n = n;
43
44
_nums = new Num[_n];
45
46
for (int i = 0; i < _n; i++)
47
{
48
_nums[i].num = i + 1;
49
_nums[i].state = State.LEFT;
50
}
51
52
_totle = getTotole(_n);
53
}
54
55
private int getTotole(int n)
56
{
57
if (n == 1)
58
{
59
return n;
60
}
61
else
62
{
63
return n * getTotole(n - 1);
64
}
65
}
66
67
/// <summary>
68
/// Find the lagest num index which need move.
69
/// </summary>
70
/// <returns> the index of the lagest num which need move.</returns>
71
private int getLargestMoveNumIndex()
72
{
73
int maxNum = 0;
74
int index = -1;
75
76
for (int i = 0; i < _n; i++)
77
{
78
if (_nums[i].state == State.LEFT && i == 0)
79
{
80
continue;
81
}
82
83
if (_nums[i].state == State.RIGHT && i == _n - 1)
84
{
85
continue;
86
}
87
88
if (_nums[i].state != State.STAY)
89
{
90
if (maxNum < _nums[i].num)
91
{
92
maxNum = _nums[i].num;
93
index = i;
94
}
95
}
96
}
97
98
return index;
99
}
100
101
/// <summary>
102
/// Swap two nums.
103
/// </summary>
104
/// <param name="index1"> the 1st num's index.</param>
105
/// <param name="index2"> the 2nd num's index.</param>
106
private void swap(int index1, int index2)
107
{
108
Num tempNum = _nums[index1];
109
110
_nums[index1] = _nums[index2];
111
_nums[index2] = tempNum;
112
}
113
114
/// <summary>
115
/// Calculate nums state which are large than last move num.
116
/// </summary>
117
/// <param name="largestMoveNumIndex"></param>
118
private void caluState(int lastNum)
119
{
120
for (int i = 0; i < _n; i++)
121
{
122
if (_nums[i].num > lastNum)
123
{
124
if (_nums[i].state == State.LEFT)
125
{
126
_nums[i].state = State.RIGHT;
127
}
128
else if (_nums[i].state == State.RIGHT)
129
{
130
_nums[i].state = State.LEFT;
131
}
132
}
133
}
134
}
135
136
/// <summary>
137
/// Get the nums vaule.
138
/// </summary>
139
/// <returns>The nums vaule.</returns>
140
private System.Int64 getNumVaule()
141
{
142
System.Int64 numVaule = 0;
143
144
for (int i = 0; i < _n; i++)
145
{
146
numVaule += (System.Int64)(_nums[i].num * Math.Pow(10, _n - i - 1));
147
}
148
149
return numVaule;
150
}
151
152
public System.Int64 CreatOneNum()
153
{
154
int largestMoveNumIndex = getLargestMoveNumIndex();
155
156
if (largestMoveNumIndex != -1)
157
{
158
int lastNum = _nums[largestMoveNumIndex].num;
159
160
int index = largestMoveNumIndex + (int)_nums[largestMoveNumIndex].state;
161
162
swap(index, largestMoveNumIndex);
163
164
caluState(lastNum);
165
}
166
167
return getNumVaule();
168
}
169
170
public void Creat()
171
{
172
for (int i = 0; i < _totle; i++)
173
{
174
System.Int64 num = CreatOneNum();
175
176
System.Console.WriteLine(num);
177
}
178
179
System.Console.WriteLine("Totle:" + _totle);
180
}
181
}
182
}
183

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

152

153

154

155

156

157

158

159

160

161

162

163

164

165

166

167

168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

183

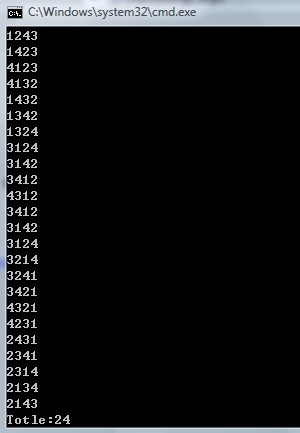