数据结构-链表 学习笔记
典型的链表结构,每个结点都应包括以下两部分:
数据部分:保存的是该结点的实际数据;
地址部分:保存的是下一个结点的地址;
链表结构是一种动态存储分配的结构形式,可根据需要动态申请所需的内存单元。
链表由许多结点构成,在进行链表操作时,首先需要定义一个“头引用”变量,该引用变量指向链表结构的第一个结点,第一个结点
的地址部分又指向第二个结点...,直到最后一个结点。最后一个结点不再指向其他结点,称为“表尾”,一般在表尾的地址部分放一个
空地址“null”,链表到此结束。
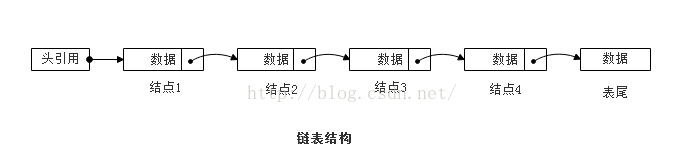
图1
Java代码实现:
/**
* 线性表->链表()
*
* 初始化
* 计算表长
* 获取结点
* 查找结点
* 插入结点
* 删除结点
* @see java.util.LinkedList
*/
public class MyLinkedList {
Node first; // 第一个结点
Node last; // 最后一个结点
private int size;
/** 初始化 */
public MyLinkedList() {
}
/** 初始化 */
public MyLinkedList(Collection<?> c) {
this();
Object[] objs = c.toArray();
int numNew = objs.length;
if (numNew == 0)
return;
for (Object o : objs) {
Node newNode = new Node(last, o, null);
if (last == null) {
first = newNode;
} else {
last.next = newNode; // last指向上一个节点,last.next指向null,没有设置.
}
last = newNode; // 将新添加的节点设为last.
}
size += numNew; // 计数
}
/** 计算长度 */
public int size() {
return size;
}
/** 获取结点 */
public Node get(int index) {
if (index < (size >> 1)) {
Node n = first;
for (int i = 0; i < index; i++)
n = n.next; // 向后找
return n;
} else {
Node n = last;
for (int i = size - 1; i > index; i--)
n = n.prev; // 向前找
return n;
}
}
/** 查找结点 */
public int getIndex(Object o) {
int index = 0;
if (o == null) {
for (Node n = first; n != null; n = n.next) {
if (n.data == null)
return index;
index++;
}
} else {
for (Node n = first; n != null; n = n.next) {
if (n.data.equals(o))
return index;
index++;
}
}
return -1;
}
/** 插入结点 */
public boolean add(Object o) {
final Node e = new Node(last, o, null);
if (first == null)
first = e;
else
last.next = e;
last = e;
size++;
return true;
}
/** 删除结点 */
public boolean remove(Object o) {
if (o == null) {
for (Node n = first; n != null; n = n.next) {
if(n.data == null){
unlink(n);
return true;
}
}
} else {
for (Node n = first; n != null; n = n.next) {
if(n.data.equals(o)){
unlink(n);
return true;
}
}
}
return false;
}
/** 解除结点间关联 */
void unlink(Node x) {
final Node next = x.next;
final Node prev = x.prev;
if (prev == null) {
first = next;
} else {
prev.next = next;
x.prev = null;
}
if (next == null) {
last = prev;
} else {
next.prev = prev;
x.next = null;
}
x.data = null;
size--;
}
/** 结点 */
private static class Node {
Object data;
Node prev; // 上一个结点
Node next; // 下一个结点
Node(Node prev, Object element, Node next) {
this.data = element;
this.prev = prev;
this.next = next;
}
}
/**转换为数组*/
public Object[] toArray() {
final Object[] o = new Object[size];
int i = 0;
for(Node n = first; n != null; n = n.next)
o[i++] = n.data;
return o;
}
public String toString() {
if(size==0)
return "[]";
Object[] o = toArray();
return "[" + Arrays.toString(o) + "]";
}
}
注: