Time类常用变量
time
//程序运行后持续的时间
Debug.Log(Time.time);
deltaTime
//每帧的时
Debug.Log(Time.deltaTime);
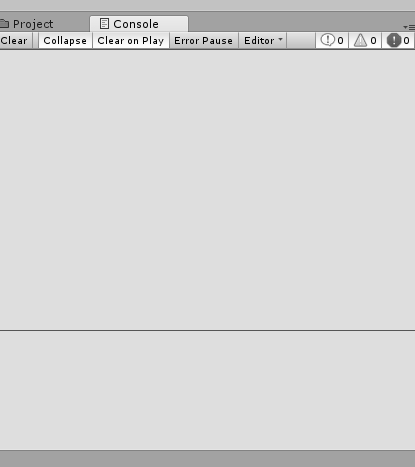
{
}
void Update () {
timer += Time.deltaTime;
if (timer >= timeInterval)
{
timer = 0;
Debug.Log("发射子弹");
}
}
}
fixedDeltaTime
//固定的增量时间(默认每次0.02 可以在Eidt - project settings - Time - Fixed Timestep修改)
Debug.Log(Time.fixedDeltaTime);
timeScale
//控制时间的快慢
Debug.Log(Time.timeScale);
public class part05 : MonoBehaviour {
private void Start()
{
//控制时间的快慢,值为0停止,值为1正常运行,大于1速度变快,小于1速度变慢,该值值作用于Time有关的代码
Time.timeScale = 0;
}
void Update () {
transform.Translate(new Vector3(1, 0, 0) * Time.deltaTime);
}
}
//上面的代码,transform是不会通过x轴移动的,如果把 *Time.deltaTime 删除掉,
//即使Time.timeScale=0 ,transform通过x轴移动,因为x轴移动的值不再跟Time有关
Vector3
magnitude
//得到向量的长度
Debug.Log(direction.magnitude);
//比如A 到B之间的距离就叫向量长度
normalized
public class part06 : MonoBehaviour {
public Transform sphere;
//立方体指向目标位置的方向向量
Vector3 direction;
//标准化向量
Vector3 nor;
float angle;
void Start () {
direction = sphere.position - transform.position;
//标准化向量,方向不变,长度为1
nor = direction.normalized;
Debug.Log(nor);
}
}
Lerp 插值
public class part06 : MonoBehaviour {
public Transform sphere;
void Update () {
//使用差值,让目标有快到慢的移动到目标位置,但是达不到目标位置
transform.position = Vector3.Lerp(transform.position, sphere.position, Time.deltaTime);
}
}
MoveTowards 移向
public Transform sphere;
void Update () {
//匀速移动到目标位置,并且可以到达目标位置
transform.position = Vector3.MoveTowards(transform.position, sphere.position, Time.deltaTime);
}
Vector简写方法
//(0,1,0)简写
Debug.Log(Vector3.up);
//(0,-1,0)简写
Debug.Log(Vector3.down);
//(1,0,0)简写
Debug.Log(Vector3.right);
//(-1,0,0)简写
Debug.Log(Vector3.left);
//(0,0,1)简写
Debug.Log(Vector3.forward);
//(0,0,-1)简写
Debug.Log(Vector3.back);
//(1,1,1)简写
Debug.Log(Vector3.one);
//(0,0,0)简写
Debug.Log(Vector3.zero);
也可以这样写
Debug.Log(Vector3.up * 5);
Vector简写应用
平时在写一个代码旋转的时候可能会这样写
void Update () {
//使用标准化向量让立方体按照指定的速度朝某个方向移动
transform.Translate(nor * Time.deltaTime * 1.5f);
//绕着Y轴旋转
transform.Rotate(new Vector3(0, 60,0) * Time.deltaTime);
}
但是unity中能不用new 尽量不要用new ,而且代码又长,该怎么样优化呢?
void Update () {
//使用标准化向量让立方体按照指定的速度朝某个方向移动
transform.Translate(nor * Time.deltaTime * 1.5f);
//绕着Y轴旋转,这样代码及少 又不用new
transform.Rotate(Vector3.up*60 * Time.deltaTime);
}
Quaternion 四元数
LookRotation 注视旋转
//指向目标的向量
Vector3 dir;
//要旋转的角度四元数
Quaternion qua;
void Start () {
//dir 等于 target 的位置 减 transform 的位置 获得一个vector3
dir = target.position - transform.position;
//吧指向目标的向量转换成四元数
qua= Quaternion.LookRotation(dir);
Debug.Log(qua);
}
Lerp 插值
//四元素
public Transform target;
//指向目标的向量
Vector3 dir;
//要旋转的角度四元数
Quaternion qua;
void Start () {
}
void Update () {
//dir 等于 target 的位置 减 transform 的位置 获得一个vector3
dir = target.position - transform.position;
//吧指向目标的向量 转化为四元数
qua = Quaternion.LookRotation(dir);
//使用四元数的插值,让目标由快到慢的旋转到目标方向
transform.rotation = Quaternion.Lerp(transform.rotation, qua, Time.deltaTime);
}
Euler 欧拉角
void Start () {
//沿Y轴旋转60度
transform.rotation = Quaternion.Euler(0, 60, 0);
}
indentity 角度归零
void Start () {
//表示没有任何旋转 旋转角度归0
transform.rotation = Quaternion.identity;
}
Mathf
Clamp
void Start () {
//如果给定的值在最大值和最小值之间,返回给定值
//如果给定的值小于最小的值,则返回最小的值,.如果给定的值大于最大的值,则返回最大值
float f = Mathf.Clamp(2.5f, 1f, 3f);
Debug.Log(f);
}
设置给定值大于最大值
void Start () {
//如果给定的值在最大值和最小值之间,返回给定值
//如果给定的值小于最小的值,则返回最小的值,.如果给定的值大于最大的值,则返回最大值
float f = Mathf.Clamp(5f, 1f, 3f);
Debug.Log(f);
}
`
设置给定值小于最小值
void Start () {
//如果给定的值在最大值和最小值之间,返回给定值
//如果给定的值小于最小的值,则返回最小的值,.如果给定的值大于最大的值,则返回最大值
float f = Mathf.Clamp(0.5f, 1f, 3f);
Debug.Log(f);
}
Clamp 练习
比如我想给 一个物体修改x的值23.5f
可能第一时间会想到下面的代码
void Start () {
transform.position.x = 23.5f;
}
//他会报错,这样直接赋值的话,他会认为你直接赋值给了结构体
//因为结构体赋值的时候只能整体赋值(要赋值的话X,Y,Z都要赋值)
这是我们需要这样写
void Start () {
transform.position = new Vector3(23.5f, transform.position.y, transform.position.z);
}
那现在我们需要让 x轴在10 和-10的区域内 该怎么办呢?
//因为要实施判断 把代码放到update里面
void Update () {
transform.position = new Vector3(Mathf.Clamp(transform.position.x, -10, 10), transform.position.y, transform.position.z);
}
Random 随机
Range
//[2,100) 大于等于2 小于 不等于100
float f= Random.Range(1, 101);
Value
// 取值在0.0--1.0之间
float f = Random.value;
Debug.Log(f);