python安装的版本是3.7.0
目录
第2章 数据类型,运算符和表达式
第1讲 标识符,常量和变量
标识符
包括 1.字母; 2.数字;3.下划线
注意 单独的下划线(_)用于表示上一次运算的结果
标识符不能与关键字一样
python中有33个关键字
>>> import keyword
>>> print(keyword.kwlist)
['False', 'None', 'True', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
keyword.iskeyword('and')
keyword.kwlist是一个列表,里面放着所有的关键字。
keyword.iskeyword()接收一个字符串参数,返回其是不是关键字(True/False)
id() 返回对象的内存地址
isinstance() 函数来判断一个对象是否是一个已知的类型
第2讲 基本数据类型
数据类型包括
整型(int),实型(float),字符型(str),布尔型(bool),复数类型
整形类型
十进制整数:由0到9组成
二进制整数:以ob或0B为前缀,由0或1组成
八进制整数:以0o或0O为前缀,其后由0至7的数字组成。
十六进制整数:以0x或0X开头,其后由0至9的数字和a至f字母或A至F字母组成。
实数又称浮点型,一般有两种表示形式:
十进制小数形式:由数字和小数点组成(必须有小数点)
指数形式,用科学计数法表示的浮点数。用字母e(或E)表示以10为底的指数,e之前为数字部分,之后为指数部分。
字符型数据可以使用一对单引号,双引号或者三引号。
布尔类型有两个值:True和False
布尔型还可以与其他数据类型进行逻辑运算,
Python规定:0,空字符串,None为False,其他数字和非空字符串为True
复数由两部分组成:实部和虚部
复数的形式为:实部+虚部j
>>> x=3+5j
>>> x.real
3.0
>>> x.imag
5.0
第3讲 算术运算符和赋值运算符
算术运算符:
+ 加法运算符或正值运算符, 两个变量相加求和
- 减法运算符或负值运算符, 两个变量相减求差
* 乘法运算符, 变量相乘求积
/ 除法运算符, 变量相除求商
// 取整除运算符, 取除法后的整数部分
** 求幂运算符, 次方,求变量(a)的n次幂->(a)?,可以用pow()函数代替
% 取模运算符, 取模,得到除法结果第一位余数
赋值运算符
=
可以通过链式赋值将同一个值赋给多个变量
x=y=5
多变量并行赋值
变量1,变量2,...,变量n=表达式1,表达式2,...,表达式n
例如:
x,y,z=2,5,8
两个变量交换数据
x,y=y,x
符合的赋值运算符
+=,-=,*=,/=,//=,**=,%=,>>=,&=,|=和^=
例如 a+=3 等价于 a=a+3
第4讲 关系运算符和逻辑运算符
关系运算符:>,>=,<,<=,==,!=
逻辑运算符: and,or,not
第5讲 成员运算符合同一运算符
成员运算符:in ,not in
同一运算符:is,is not
==用来比较判断两个对象的值是否相等
is比较判断的是对象间的唯一身份标识
第3章 输入与输出
第2讲 程序的基本结构
例2:编程计算1+2+3+....+100
代码1
>>> while i<=100:
sum+=i;
i=i+1;
>>> print("sum=%d"%sum)
sum=5050
代码2
>>> sum,i=0,1
>>> for i in range(1,101):
sum+=i
>>> print("sum=%d"%sum)
sum=10100
第3讲 标准输入输出(Input and Output)
标准输入input()
一般格式:input([提示字符串])
Python2.x中提供raw_input(),该函数将所有用户的输入都作为字符串看待,返回字符串类型
Python3.x中,仅保留了input()函数,将所有输入默认为字符串处理
>>> x=input("Please enter your input:")
Please enter your input:5
>>> x
'5'
>>> x=input("Please enter your input:")
Please enter your input:[1,2,3]
>>> x
'[1,2,3]'
>>>
如果要输入数值类型数据,可以使用类型转换函数将字符串转换为数组
>>> x=input("Please enter your input:")
Please enter your input:5
>>> x
'5'
input可以给多个变量赋值
>>> x,y=eval(input())
3,4
>>> x
3
>>> y
4
eval([字符串]) 将字符串str当成有效地表达式来求值,并返回计算结果
>>> x=8
>>> eval('2*x')
16
>>> '2*x'
'2*x'
>>> 'print(x)'
'print(x)'
>>> eval('print(x)')
8
print()函数地一般形式为:
print([输入项1,输入项2,...,输入项n],[,sep=分隔符],[,end=结束符])
>>> a,b=10,22
>>> print(a,b)
10 22
>>> print(a,b,sep=":")
10:22
>>> print(a,b,sep=":",end="%")
10:22%
标准输出--表达式
>>> str="ok"
>>> str
'ok'
>>> 2
2
>>> 2*4
8
第4讲格式化输出
1.格式控制字符串%(输出项1,输出项2,...输出项)
常用格式说明符
d或i | 十进制格式 |
o | 八进制格式 |
x或者X | 十六进制格式 |
c | 一个字符格式 |
s | 字符串格式 |
e或E | 指数格式 |
g或G | e和f中较短一种格式 |
f | 实数格式 |
>>> print("sum=%o"%123)
sum=173
>>> print("sum=%o,c=%o"%(1,2))
sum=1,c=2
>>> print("sum=%o,aa=%o,ddd=%o"%(1,2,88))
sum=1,aa=2,ddd=130
>>> print("sum=%d"%9)
sum=9
>>> print("sum=%o"%9)
sum=11
>>> print("sum=%x"%9)
sum=9
>>> print("sum=%d"%9)
sum=9
>>> print("sum=%s"%9)
sum=9
>>> print("sum=%e"%9)
sum=9.000000e+00
>>> print("sum=%g"%9)
sum=9
>>> print("sum=%f"%9)
sum=9.000000
附加格式说明符
一般形式: %[附加格式说明符]格式符
附加格式说明符 | 格式说明 |
m | 域宽,十进制整数,用以描述输出项数据所占宽度 |
n | 附加域宽,十进制整数,用于指定实型数据小数部分的输出位数 |
- | 输出数据左对齐,默认时为右对齐 |
+ | 输出正数时,以 +号开头 |
# | 作为o,x的前缀时,输出结果前面加上前导符号0,0x |
>>> print("%f"%8.123)
8.123000
>>> print("%6.2f"%8.123)
8.12
>>> print("% 6.2f"%8.123)
8.12
>>> print("%06.2f"%8.123)
008.12
>>> print("%X%d"%(10,100))
A100
>>> print("%4X%d"%(10,100))
A100
>>> print("%04X%d"%(10,100))
000A100
>>> print("%4X%2d"%(10,100))
A100
>>> print("%-4X%2d"%(10,100))
A 100
>>> print("%e"%1.2888)
1.288800e+00
>>> print("%.2e"%1.2888)
1.29e+00
format
格式字符串.format()(输出项1,输出项2,....,输出项n)
其中格式字符串使用大括号括起来,一般形式为:
{[序号或键]:格式说明符}
1.使用“{序号}”形式的格式说明符
>>> "{}{}".format("hello","world")
'helloworld'
>>> "{0}{1}".format("hello","world")
'helloworld'
>>> "{1}{0}{1}".format("hello","world")
'worldhelloworld'
2.使用"{序号:格式说明符}"形式的格式说明符
>>> "{0},{1}".format(3.1234455333,100)
'3.1234455333,100'
>>> "{0:.2f},{1}".format(3.1234455333,100)
'3.12,100'
3.使用“{序号/键:格式说明符}”形式的格式说明符
>>> "{0},pi={x}".format("圆周率",x=3.14)
'圆周率,pi=3.14'
第4章选择结构程序设计
第1讲分支结构
1.单分支if语句
形式:if 表达式:
语句
>>> a,b=eval(input("请输入两个数(a,b)"))
请输入两个数(a,b)4,3
>>> max=a
>>> if b>max:
max=b
>>> print("max=%d" %max)
max=4
2.if/else
形式:if 表达式:
语句1
else:
语句2
3.if 表达式1:
语句1
elif 表达式2:
语句2
elif 表达式3:
语句3
....
elif 表达式n-1:
语句n-1
[else:表达式n:
语句n]
第5章 循环结构程序设计
while 表达式:
语句
>>> sum=0
>>> i=1
>>> while i<=200:
sum+=i
i=i+1
>>> print("sum= %d"%sum)
sum= 20100
while 表达式:
循环体
else:
语句
for 目标变量 in 序列对象:
循环体
一般形式:
for 目标变量 in 序列对象:
语句块
else:
语句
range()一般形式:
range([start,]end[,step])
>>> for i in range(5):
print(i)
0
1
2
3
4
>>> for i in range(2,4):
print(i)
2
3
>>> for i in range(2,20,3):
print(i)
2
5
8
11
14
17
第1讲循环语句
>>> n=7 #打印7行
>>> for j in range(1,n+1):
for i in range(1,2*j):
print("*",end="")
print()
*
***
*****
*******
*********
***********
*************
>>> n=7 #打印7行的金字塔
>>> for j in range(1,n+1):
for i in range(1,n-j+1):
print(" ",end="")
for i in range(1,2*j):
print("*",end="")
print()
*
***
*****
*******
*********
***********
*************
>>> for j in range(1,10,1):
for i in range(1,j+1,1):
print("%d*%d=%2d "%(i,j,i*j),end="")
print()
1*1= 1
1*2= 2 2*2= 4
1*3= 3 2*3= 6 3*3= 9
1*4= 4 2*4= 8 3*4=12 4*4=16
1*5= 5 2*5=10 3*5=15 4*5=20 5*5=25
1*6= 6 2*6=12 3*6=18 4*6=24 5*6=30 6*6=36
1*7= 7 2*7=14 3*7=21 4*7=28 5*7=35 6*7=42 7*7=49
1*8= 8 2*8=16 3*8=24 4*8=32 5*8=40 6*8=48 7*8=56 8*8=64
1*9= 9 2*9=18 3*9=27 4*9=36 5*9=45 6*9=54 7*9=63 8*9=72 9*9=81
第6章 序列
第1讲 列表的基本操作
- 列表是包含0个或多个对象引用的有序序列
- 列表的长度和内容都是可变的
- 列表没有长度限制,元素类型可以不同
- 所有元素放在一对方括号“[”和“]”中,相邻元素之间用逗号分割开
>>> a_list=['physics','chemistry',2017,2.5] #使用赋值运算符"="创建列表
>>> a_list[1] #使用列表索引的方法读取元素 列表名[索引]
'chemistry'
>>> a_list[3:6] # 列表名[开始索引:结束索引:步长]
[2.5]
>>> a_list=[1,2,3,4,5,6,7,8]
>>> a_list=a_list+[5,6] #使用“+"运算符将一个新列表添加在原列表的尾部
>>> a_list
[1, 2, 3, 4, 5, 6, 7, 8, 5, 6]
>>> a_list.append("Python")#使用append()方法向列表尾部添加一个新的元素
>>> a_list
[1, 2, 3, 4, 5, 6, 7, 8, 5, 6, 'Python']
>>> a_list.extend([2017,"C"])
>>> a_list # 使用extend()方法向将一个列表添加导原列表的尾部
[1, 2, 3, 4, 5, 6, 7, 8, 5, 6, 'Python', 2017, 'C']
>>> a_list.insert(1,3.5) #用insert()方法向将一个元素插入导列表的指定位置
>>> a_list
[1, 3.5, 2, 3, 4, 5, 6, 7, 8, 5, 6, 'Python', 2017, 'C']
>>> a_list.index(5) #index()方法可以获取指定元素首次出现的下标 index(value,[,start,[,end]]
5
>>> a_list.index(5,6)
9
>>>
>>> a_list.count(6) #count()方法统计列表中指定元素出现的次数
2
>>> a_list.in(6)
SyntaxError: invalid syntax
>>> 6.in(a_list)
True
>>> 6.not in(a_list) # in 或not in 运算符检索某个元素是否在该列表中
False
>>> del a_list[3] #用del删除列表中指定位置的元素
>>> a_list
[1, 3.5, 2, 4, 5, 6, 7, 8, 5, 6, 'Python', 2017, 'C']
>>> b_list=[10,7,1.5]
>>> b_list
[10, 7, 1.5]
>>> del b_list #用del删除整个列表
>>> b_list
Traceback (most recent call last):
File "<pyshell#153>", line 1, in <module>
b_list
NameError: name 'b_list' is not defined
>>> a_list.remove(5) #remove()删除首次出现的指定元素
>>> a_list
[1, 3.5, 2, 4, 6, 7, 8, 5, 6, 'Python', 2017, 'C']
>>> a_list.pop()
'C'
>>> a_list.pop(3) # pop()方法删除并返回指定位置上的元素
4
>>> a_list
[1, 3.5, 2, 6, 7, 8, 5, 6, 'Python', 2017]
第2讲 列表的常用函数和列表应用
cmp(列表1,列表2) 比较两个列表
>>> len([1,2.0,"hello"])# 返回列表中的元素个数
3
>>> a_list=['123','xyz','zara','abc']
>>> max(a_list)#返回列表中的最大元素
'zara'
>>> min(a_list)#返回列表中的最小元素
'123'
>>> a_list=[23,-12,33,2.5]
>>> sum(a_list) # 对数值型列表的元素进行求和运算
46.5
>>> sorted(a_list)#对列表进行排序,默认是按照升序排列
[-12, 2.5, 23, 33]
>>> sorted(a_list,reverse=True)#对列表进行降序排序
[33, 23, 2.5, -12]
>>> a_list.sort()#对列表进行排序,默认是升序排序
>>> a_list
[-12, 2.5, 23, 33]
>>> a_list.sort(reverse=True)
>>> a_list
[33, 23, 2.5, -12]
>>> a_list.reverse()#对列表中的元素进行翻转存放
>>> a_list
[-12, 2.5, 23, 33]
第3讲 元组
- 列表是可变序列,而元组是不可变序列
- 元组的处理速度和访问速度比列表快
- 元组在字典中科院作为关键字使用,而列表不能作为关键字使用,因为列表不是不可改变的
>>> #创建元组
>>> a_tuple=("physics","chemistry",2017,2.5)
>>> b_tuple=(1,2,(3.0,"hello world"))
>>> c_tuple=('wade',3.0,81,[])
>>> d_tuple=()
>>> e_tuple=tuple()
>>> ''' 创建只包含一个1个元素的元组
'''
' 创建只包含一个1个元素的元组\n'
>>> x=(1)
>>> x
1
>>> y=(1,)
>>> y
(1,)
>>> #用序列索引的方法读取元素 元组名[索引]
>>> a_tuple[1]
'chemistry'
>>> a_tuple[-1]
2.5
>>> a_tuple[4]#下标超过
Traceback (most recent call last):
File "<pyshell#38>", line 1, in <module>
a_tuple[4]#下标超过
IndexError: tuple index out of range
>>> a_tuple[1:3]# 元组名[开始索引:结束索引:步长]
('chemistry', 2017)
>>> a_tuple[::3]
('physics', 2.5)
>>> a_tuple.index(2017) #index可以获取指定元素首次出现的下标 index(value,[,start[,end]])
2
>>> a_tuple.count(2017) #统计列表中指定元素出现的次数
1
>>> "chemistry" in a_tuple #in 或not in 运算符检索某个元素是否在该列表中
True
>>> 0.5 in a_tuple
False
>>> del a_tuple #del命令删除元组
>>> a_tuple
Traceback (most recent call last):
File "<pyshell#51>", line 1, in <module>
a_tuple
NameError: name 'a_tuple' is not defined
>>> #list()函数tuple()实现相互转换
>>> aa_list=["physics","chemistry",2017,2.5,[0.5,3]]
>>> type(aa_list)
<class 'list'>
>>> tuple(aa_list)
('physics', 'chemistry', 2017, 2.5, [0.5, 3])
>>> type(tuple(aa_list))
<class 'tuple'>
>>> type(b_tuple)
<class 'tuple'>
>>> list(b_tuple
)
[1, 2, (3.0, 'hello world')]
>>> type(list(b_tuple))
<class 'list'>
第4讲 字符串基本操作
Python中的字符串用一对单引号或双引号括起来
三重引号表示,可以保留所有字符串的格式信息
使用赋值运算符“=”或str()函数
>>> str1="Hello"
>>> str1
'Hello'
>>> str2='p\'ddd\''
>>> str2
"p'ddd'"
>>> str3=str()
>>> str3
''
>>>
读取字符:字符名[索引]
>>> str1="hello"
>>> str1[0]
'h'
>>> str1[-1]
'o'
字符串切片:字符串名[开始索引:结束索引:步长]
>>> str[0:5:2]
'Pto'
>>> str[:]
'Python Program'
>>> str[-1:-20]
''
>>> str[-1:-20:-1]
'margorP nohtyP'
"+"将两个字符串对象连接起来
>>> "11"+"22" #+
'1122'
>>> "11"*3 #*
'111111'
>>> 3*"dd"
'dddddd'
>>> "A"=="A"
True
>>> "A"=="a"
False
第5讲 字符串常用函数及其应用
方法 | 功能 |
str.find(substr,[start,[,end]]) | 定位子串substr在str中第一次出现的位置 |
str.replace(old,new(,max)) | 用字符串new替代str中的old |
str.split([sep]) | 以sep为分隔符,把str分隔出一个列表 |
str.join(sequence) | 把str的元素用连接符sequence连接起来 |
str.count(substr,[start,[,end]]) | 统计str中有多少个sbustr |
str.strip() | 去掉str中两端空格 |
str.lstrip() | 去掉str中左边空格 |
str.rstrip() | 去掉str中右边空格 |
str.lsalpha() | 判断str是否全是字母 |
str.isdigit() | 判断str是否全是数字 |
str.isupper() | 判断str是否全是小写字母 |
str.islower() | 判断str是否全是小写字母 |
str.lower() | 转换str中所有大写字母为小写 |
str.upper() | 转换str中所有小写字母为大写 |
>>> str1="beijing xi'an tianjin beijing chongqing"
>>> str1.find("beijing")#子串查找 str.find(substr,[start,[,end]])
0
>>> str1.find("beijing",3)
22
>>> str1.find("beijing",3,20)
-1
>>> str1.replace("beijing","shoudou")#字符串替换,str.replace(old,new(,max))
"shoudou xi'an tianjin shoudou chongqing"
>>> str1.replace("shoudou","renkou",1)
"beijing xi'an tianjin beijing chongqing"
>>> str1.replace("beijing","renkou",1)
"renkou xi'an tianjin beijing chongqing"
>>> str1.split("beijing")
['', " xi'an tianjin ", ' chongqing']
>>> str1.split()#字符串分离 str.split([sep])
['beijing', "xi'an", 'tianjin', 'beijing', 'chongqing']
>>> str1.split(",")
["beijing xi'an tianjin beijing chongqing"]
>>> s4=["beijing","xi'an","tianjin","chongqing"]
>>> sep="-->"
>>> str=sep.join(s4)
>>> str
"beijing-->xi'an-->tianjin-->chongqing"
>>> #join()字符串连接,sep.join(sequence)
第7章 字典和集合
第1讲 字典
形式:
{<键1>:<值1>,<键2>:<值2>,...,<键n>:<值n>}
字典的键:不可变类型,例如整数,实数,复数,字符串,元组等。
值可以重复,一个键只能对应一个值,但多个键可以对应相同的值
方法 | 功能 |
---|---|
dic(seq) | 用(键,值)对的(或者映射和关键字参数)建立字典 |
get(key[,returnvalue]) | 返回key的值,若无key而指定了returnvalue,则返回returnvalue值,若无此值则返回None |
has_key(key) | 如果key存在于字典中南,就返回1(真);否则返回0(假) |
items() | 返回一个由元组构成的列表,每个元组包含一对键-值对 |
popitem() | 删除任意键-值对,并作为两个元素的元组返回。如字典为空,则返回KeyError异常 |
update(newDictionary) | 将来自newDictionary的所有键-值添加导当前字典,并覆盖同名键的值 |
values() | 以列表的方式返回字典的所有“值” |
clear() | 从字典删除所有项 |
>>> #创建字典
>>> a_dict={"Alice":95,"Beth":82,"Tom":65.5,"Emily":95}
>>> a_dict
{'Alice': 95, 'Beth': 82, 'Tom': 65.5, 'Emily': 95}
>>> b_dict={}
>>> b_dict
{}
>>> c_dict=dict(zip(["one","two","three"],[1,2,3]))#使用内建函数dict()创建字典
>>> c_dict
{'one': 1, 'two': 2, 'three': 3}
>>> d_dict=dict(one=1,two=2,three=3)
>>> e_dict=dict([("one",1),("two",2),("three",3)])
>>> f_dict=dict((("one",1),("two",2),("three",3)))
>>> g_dict=dict()
>>> h_dict={}.fromkeys((1,2,3),"student")#使用内建函数fromkeys创建字典
>>> h_dict
{1: 'student', 2: 'student', 3: 'student'}
>>> i_dict={}.fromkeys((1,2,3))
>>> i_dict
{1: None, 2: None, 3: None}
>>> j_dict={}.fromkeys(())
>>> j_dict
{}
>>> a_dict["Tom"]#使用下标的方法读取字典元素的读取
65.5
>>> a_dict[100]
Traceback (most recent call last):
File "<pyshell#9>", line 1, in <module>
a_dict[100]
KeyError: 100
>>> a_dict.get("Alice")#使用get方法获取执行键对应得值,dict.get(key,default=None)
95
>>> a_dict.get("a","address")
'address'
>>> a_dict.get("a")
>>> a_dict
{'Alice': 95, 'Beth': 82, 'Tom': 65.5, 'Emily': 95}
>>> a_dict["Tom"]=79#使用“字典名[键名]=[键值]”对字典元素的修改和添加
>>> a_dict
{'Alice': 95, 'Beth': 82, 'Tom': 79, 'Emily': 95}
>>> a_dict["Eric"]=98
>>> a_dict
{'Alice': 95, 'Beth': 82, 'Tom': 79, 'Emily': 95, 'Eric': 98}
>>> b_dict={"Eric":100,"Tom":79}
>>> a_dict.update(b_dict)#update对字典元素的添加和修改
>>> a_dict
{'Alice': 95, 'Beth': 82, 'Tom': 79, 'Emily': 95, 'Eric': 100}
>>> del a_dict["Emily"]#del删除字典中指定“键”对应的元素
>>> a_dict
{'Alice': 95, 'Beth': 82, 'Tom': 79, 'Eric': 100}
>>> del a_dict[0]
Traceback (most recent call last):
File "<pyshell#23>", line 1, in <module>
del a_dict[0]
KeyError: 0
>>> a_dict.pop("Alice")#pop方法删除并返回指定“键”的元素
95
>>> a_dict
{'Beth': 82, 'Tom': 79, 'Eric': 100}
>>> a_dict.popitem()#使用popitem()方法,随机元素
('Eric', 100)
>>> a_dict
{'Beth': 82, 'Tom': 79}
>>> >>> a_dict.clear()#使用clear
>>> a_dict
{}
>>> del a_dict
>>> a_dict #使用del删除整个字典
Traceback (most recent call last):
File "<pyshell#35>", line 1, in <module>
a_dict #使用del删除整个字典
NameError: name 'a_dict' is not defined
>>> a_dict={"Alice":95,"Beth":82,"Tom":65.5,"Emily":95}
>>> a_dict.keys()#遍历字典的关键字
dict_keys(['Alice', 'Beth', 'Tom', 'Emily'])
>>> a_dict.values()#遍历字典中的值
dict_values([95, 82, 65.5, 95])
>>> a_dict.items()#遍历字典的元素
dict_items([('Alice', 95), ('Beth', 82), ('Tom', 65.5), ('Emily', 95)])
集合
0个或多个对应引用的无序排列的,不重复的数据集合体
>>> #创建集合,使用“="将一个集合赋值给一个变量
>>> a_set={0,1,2,3,4,5,6,7,8,9,10}
>>> a_set
{0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
>>> #使用集合对象的set()方法创建集合
>>> b_set=set(["physics","chemistry",2017,2.5])
>>> b_set
{2.5, 2017, 'physics', 'chemistry'}
>>> #集合中不允许有相同的元素
>>> g_set={0,0,0,0,1,1,1,1,13,4,5,6}
>>> g_set
{0, 1, 4, 5, 6, 13}
>>> #使用frozenset()方法创建一个冻结的集合
>>> e_set=frozenset("a")
>>> a_dict={e_set:1,"b":2}
>>> a_dict
{frozenset({'a'}): 1, 'b': 2}
>>> f_set=set("a")
>>> b_dict={f_set:1,"b":2}
Traceback (most recent call last):
File "<pyshell#14>", line 1, in <module>
b_dict={f_set:1,"b":2}
TypeError: unhashable type: 'set'
>>> #使用in或者循环遍历访问集合元素
>>> 1 in a_set
True
>>> 13 in set
Traceback (most recent call last):
File "<pyshell#17>", line 1, in <module>
13 in set
TypeError: argument of type 'type' is not iterable
>>> 13 in a_set
False
>>> #使用del命令
>>> del a_set
>>> a_set
Traceback (most recent call last):
File "<pyshell#21>", line 1, in <module>
a_set
NameError: name 'a_set' is not defined
>>> #add()增加元素
>>> b_set
{2.5, 2017, 'physics', 'chemistry'}
>>> b_set.add("math")
>>> b_set
{2017, 2.5, 'physics', 'chemistry', 'math'}
>>> #update增加元素
>>> b_set.update({1,2,3},{"Wade","Nash"},{0,1,2})
>>> b_set
{0, 2017, 2.5, 'physics', 'chemistry', 1, 2, 3, 'Nash', 'Wade', 'math'}
>>> #remove删除元素
>>> b_set.remove(0)
>>> b_set
{2017, 2.5, 'physics', 'chemistry', 1, 2, 3, 'Nash', 'Wade', 'math'}
>>> b_set.remove("hello")
Traceback (most recent call last):
File "<pyshell#32>", line 1, in <module>
b_set.remove("hello")
KeyError: 'hello'
>>> #discard删除元素
>>> b_set.remove(2017)
>>> b_set
{2.5, 'physics', 'chemistry', 1, 2, 3, 'Nash', 'Wade', 'math'}
>>> b_set.discard(2.5)
>>> b_set
{'physics', 'chemistry', 1, 2, 3, 'Nash', 'Wade', 'math'}
>>> b_set.discard(0)
>>>
>>> b_set
{'physics', 'chemistry', 1, 2, 3, 'Nash', 'Wade', 'math'}
>>> #pop删除任意一个元素
>>> b_set.pop()
'physics'
>>> b_set
{'chemistry', 1, 2, 3, 'Nash', 'Wade', 'math'}
>>> #clear删除所有
>>> b_set.clear()
>>> b_set
set()
>>> #交集 s1&s2&....&sn
>>> {0,1,2,3,4,5}&{0,2,3333}
{0, 2}
>>> {4,5}&{1,2}
set()
>>> #并集 s1|s2|...|sn
>>> {0,1,2,3,4,5,6,7,8,9}|{0,2,3,11,22}
{0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 11, 22}
>>> #差集
>>> {0,1,2,3,4,5,6,7,8,9}-{0,2,4,6,8}
{1, 3, 5, 7, 9}
>>> #对称差集: S1^s2^....^sn
>>> {0,1,2,3,4,5,6,7,8,9}^{0,2,4,6,8}
{1, 3, 5, 7, 9}
>>> #差集,返回左边集合有的,但是在右边集合没有的元素
>>> {1,3,5}-{1,2,3}
{5}
>>> #对称差集,返回两个集合中不重复的元素
>>> {1,3,5}^{1,2,3}
{2, 5}
>>>
第8章 函数
第1讲函数概念,定义及调用
函数地定义
一般形式:
def 函数名([形式参数表]):
函数体
[return 表达式]
>>> def print_info():
print("=======")
>>> print_info()
=======
在函数定义时,直接在函数形参后面使用赋值运算符=为其设置默认值
>>> range(2)
range(0, 2)
>>> def func(x,n=2):
f=1
for i in range(n):
f*=x
return f
>>> print(func(5))
25
>>> print(func(5,3))
125
第2讲函数参数传递
参数必须出现在参数列表的最后面
>>> def funa(a=1,b,c=2):
return a+b+c
SyntaxError: non-default argument follows default argument
>>> def funa(a,b,c=2):
return a+b+c
>>> print(funa(11,2))
15
默认参数的值只在定义时被设置计算一次,如果函数修改了对象,默认值就被修改了。
>>> def func(x,a_list=[]):
a_list.append(x)
return a_list
>>> print(func(1))
[1]
>>> print(func(2))
[1, 2]
>>> print(func(3))
[1, 2, 3]
>>> print(func(a_list=[3,4],x=44)) #指定参数 键=值
[3, 4, 44]
可变长参数
- 边长参数可以是元组或者字典类型
- 变量名前加星号*或**,以区分一般参数
- 变量名前加星号*——元组
- 变量名前加星号**——字典
可变长参数——元组
>>> def func(*para_t):
print("可变长参数数量为:")
print(len(para_t))
print("参数依次为:")
for x in range(len(para_t)):
print(para_t[x])
>>> func("a")
可变长参数数量为:
1
参数依次为:
a
>>> func("a","b")
可变长参数数量为:
2
参数依次为:
a
b
>>> func("dd","ee","tt")
可变长参数数量为:
3
参数依次为:
dd
ee
tt
可变参数——字典
>>> def func(**para_t):
print(para_t)
>>> func(a=1,b=2,c=3)
{'a': 1, 'b': 2, 'c': 3}
默认参数,可变参数,位置参数应用如下
>>> def func(x,*para,y=1):
print(x)
print(para)
print(y)
>>> func(1,2,3,4,5,6,7,8,9,10,y=100)
1
(2, 3, 4, 5, 6, 7, 8, 9, 10)
100
第3讲函数递归调用
第4讲变量的作用域
局部变量和全局变量
在同一个程序文件中,如果全局和局部变量同名,在局部变量的作用范围内,全局变量不起作用
>>> def f():
global x
x=30
y=40
print("No2:",x,y)
>>> x=10
>>> y=20
>>> print("No1:",x,y)
No1: 10 20
>>> f()
No2: 30 40
>>> print("No3:",x,y)
No3: 30 20
第5讲模块
从用户的角度看,模块也分为标准库模块和用户自定义模块
标准库模块是Python自带的函数模块
用户自定义模块
用户建立一个模块就是建立扩展名为.py的Python程序
导入模块中的函数
from 模块名 import 函数名
导入模块中所有函数
from 模块名 import *
第9章文件
第1讲 文件概述
第2讲 文本文件的读写
c:\python.txt
one:apple,water malte,desk ,chair
two=teacher,house,da,father,mather
three=tianjing,man,woman
four=pencil,yuanyuan,liuliu
>>> fp=open("c:\\python.txt","r")
>>> str=fp.read()#读取文件
>>> str
'one:apple,water malte,desk ,chair\ntwo=teacher,house,da,father,mather\nthree=tianjing,man,woman\nfour=pencil,yuanyuan,liuliu'
>>> str=fp.read()
>>> str
''
>>> str=fp.read(10)
>>> str
''
>>> fp1=open("c:\\python.txt","r")
>>> str=fp1.read(10)
>>> str
'one:apple,'
>>> str=fp1.readline()
>>> str
'water malte,desk ,chair\n'
>>> str=fp1.readline()
>>> str
'two=teacher,house,da,father,mather\n'
>>> str=fp1.readline()
>>> str
'three=tianjing,man,woman\n'
>>> str=fp1.readlines()
>>> str
['four=pencil,yuanyuan,liuliu']
>>> fp2=open("c:\\python.txt","r")
>>> str=fp2.readlines()
>>> str
['one:apple,water malte,desk ,chair\n', 'two=teacher,house,da,father,mather\n', 'three=tianjing,man,woman\n', 'four=pencil,yuanyuan,liuliu']
>>> fp3=open("c:\\python.txt","w")#写入内容
>>> fp3.write("Python")
6
>>> fp.close()
>>> fp3.close()
>>> fp3.writelines(["Python","Python programming"])
Traceback (most recent call last):
File "<pyshell#28>", line 1, in <module>
fp3.writelines(["Python","Python programming"])
ValueError: I/O operation on closed file.
>>> fp3=open("c:\\python.txt","w")
>>> fp3.writelines(["Python","Python programming"])
>>> fp3.close()
第3讲 二进制文件的读写
二进制文件写入——pack方法
pack(格式串,数据对象表)
格式字符
>>> import struct
>>> x=100
>>> y=struct.pack("i",x)
>>> y
b'd\x00\x00\x00'
>>> len(y)
4
>>> fp=open("c:\\file2.txt","wb")#w写入,b二进制。如果这个文件不存在,会创建一个
>>> fp.write(y)
4
>>> fp.close()
二进制文件写入——dump方法
第10章 Python标准库
第1讲 random库
>>> import random
>>> random.random()
0.5520555463266628
>>> random.seed()
>>> random.seed(5)
>>> random.random()
0.6229016948897019
>>> random.random()
0.7417869892607294
>>> random.randint(3,10) #返回3和10之间的整数
3
>>> random.randrange(1,10,2)#按指定步长2
7
>>> random.choice([1,2,3,4,5])#从非空序列中随机选取一个元素
2
>>> list=[20,16,10,5]
>>> random.shuffle(list)
>>> #shuffle随机打乱
>>> list
[16, 10, 5, 20]
>>> random.sample([1,2,3,4,5,6,7],k=5)#从样本或集合中随机抽取k个不重复的元素形成新的序列
[3, 4, 2, 6, 7]
>>> random.uniform(10,20)#返回一个介于10,20的浮点数
11.019744021739154
第2讲turtle库
设置画布
>>> import turtle
>>> turtle.screensize(800,600,"blue")#设置画布screensize(width,height,bg) width设置画布宽度(单位像素),height设置画布高度(单位像素),bg设置的画布背景颜色
>>> #setup(width,height,startx,starty)
>>> turtle.setup(width=0.6,height=0.6)
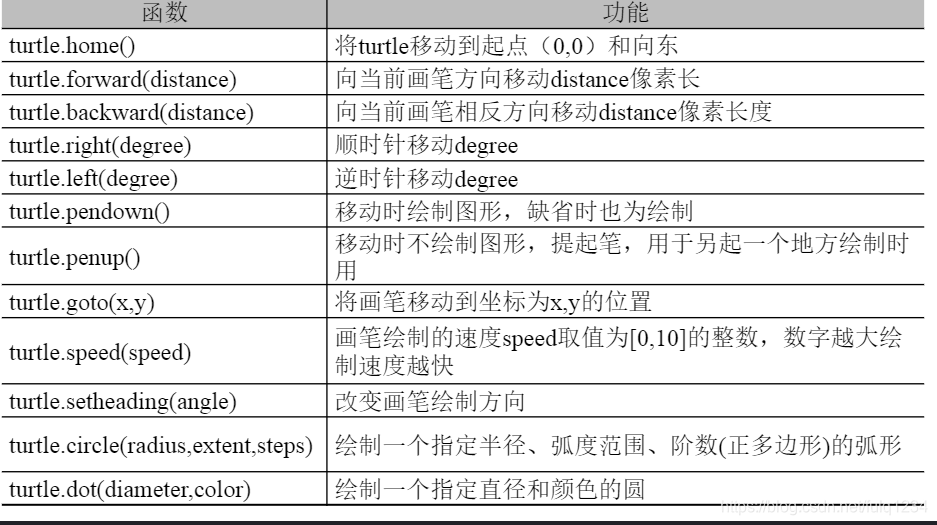
第3讲 time库
>>> import time
>>> time.time()
1626783509.6915078
>>> time.ctime()
'Tue Jul 20 20:18:33 2021'
>>> time.gmtime()
time.struct_time(tm_year=2021, tm_mon=7, tm_mday=20, tm_hour=12, tm_min=19, tm_sec=14, tm_wday=1, tm_yday=201, tm_isdst=0)
>>> time.localtime()
time.struct_time(tm_year=2021, tm_mon=7, tm_mday=20, tm_hour=20, tm_min=19, tm_sec=39, tm_wday=1, tm_yday=201, tm_isdst=0)
>>> time.strftime("%b %d %Y %H",time.gmtime())#格式化时间
'Jul 20 2021 12'
>>> time.strptime("30 Nov 17","%d %b %y")#字符串转换成时间对象
time.struct_time(tm_year=2017, tm_mon=11, tm_mday=30, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=3, tm_yday=334, tm_isdst=-1)
>>> time.asctime()#将一个tuple或struct_time形式的时间(转换为时间字符串)time.struct_time(tm_year=2017, tm_mon=11, tm_mday=30, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=3, tm_yday=334, tm_isdst=-1)
'Tue Jul 20 20:24:14 2021'