版本一
效果图(有保存按钮 antd3)
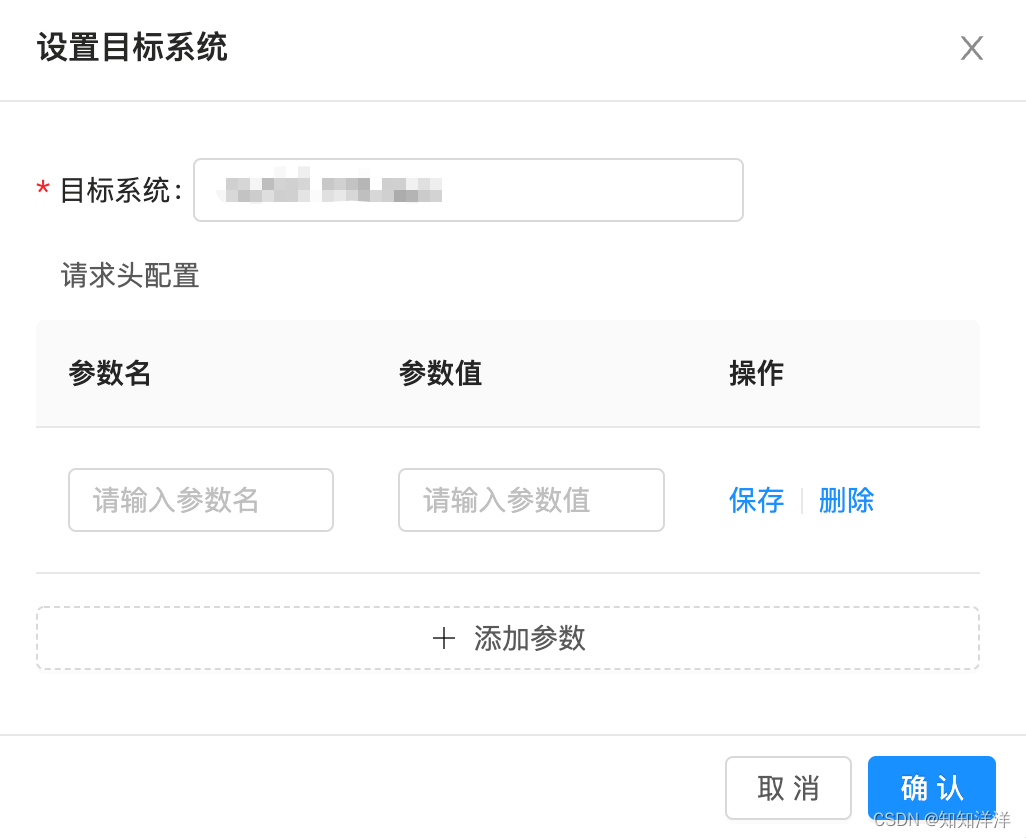
代码
import React, { Component } from "react";
import { Form, Input, Button, Table, Divider, Popconfirm, Tooltip } from 'antd';
import "./targetform.less";
class TargetForm extends Component {
state = {
targetIp: this.props.targetIp,
dataSource: [],
count: 0,
};
componentDidMount() {
}
handleAdd = () => {
const { count, dataSource } = this.state;
const newData = {
key: count,
name: "",
example: "",
save: false,
editable: false
};
this.setState({
dataSource: [...dataSource, newData],
count: count + 1
});
};
toggleEditable = (
e,
key,
name
) => {
let newData = [];
newData = [...this.state.dataSource];
const index = newData.findIndex(item => key === item.key);
newData[index].save = false;
newData[index].editable = false;
this.setState({
dataSource: newData
});
};
saveRow = (e, key) => {
this.props["form"].validateFields(
[
`table[${key}].name`,
`table[${key}].example`
],
(err, values) => {
if (!err) {
let newData = [];
newData = [...this.state.dataSource];
const index = newData.findIndex(item => key === item.key);
newData[index].save = true;
newData[index].editable = true;
this.setState({
dataSource: newData
});
}
}
);
};
handleDelete = (key) => {
const newData = [...this.state.dataSource];
this.setState({
dataSource: newData.filter((item) => item.key !== key)
});
};
handleFieldChange = (
e,
keyName,
key
) => {
let newData = [];
newData = [...this.state.dataSource];
const index = newData.findIndex(item => key === item.key);
if (keyName === "name" || keyName === "example") {
newData[index][keyName] = e.target.value;
} else {
newData[index][keyName] = e;
}
this.setState({
dataSource: newData
});
};
render() {
const { targetIp, dataSource } = this.state
const { getFieldDecorator } = this.props.form;
const columns = [
{
title: "参数名",
dataIndex: "name",
width: '35%',
render: (text, record, index) => {
if (!record.save) {
return (
<Form.Item key={index}>
{getFieldDecorator(`table[${record.key}].name`, {
initialValue: text ? text : undefined,
rules: [
{
required: true,
message: "请输入参数名!",
min: 1,
max: 64
}
]
})(
<Input
placeholder="请输入参数名"
autoFocus
onChange={e =>
this.handleFieldChange(e, "name", record.key)
}
/>
)}
</Form.Item>
);
}
return (
<Form.Item key={index}>
{getFieldDecorator(`table[${record.key}].name`, {
initialValue: text ? text : undefined
})(
<Tooltip placement="topLeft" title={text}>
<span>{text.length > 10 ? text.substr(0, 10) + '...' : text}</span>
</Tooltip>
)}
</Form.Item>
);
}
},
{
title: "参数值",
dataIndex: "example",
width: '35%',
render: (text, record, index) => {
if (!record.save) {
return (
<Form.Item key={index}>
{getFieldDecorator(`table[${record.key}].example`, {
initialValue: text ? text : undefined,
rules: [{ required: true, message: "请输入参数值!" }]
})(
<Input
placeholder="请输入参数值"
autoFocus
onChange={e =>
this.handleFieldChange(e, "example", record.key)
}
/>
)}
</Form.Item>
);
}
return (
<Form.Item key={index} >
{getFieldDecorator(`table[${record.key}].example`, {
initialValue: text ? text : undefined
})(
<Tooltip placement="topLeft" title={text}>
<span>{text.length > 10 ? text.substr(0, 10) + '...' : text}</span>
</Tooltip>
)}
</Form.Item>
);
}
},
{
title: "操作",
dataIndex: "operation",
width: '30%',
render: (text, record) => {
if (record.editable) {
return (
<span>
<a
onClick={e =>
this.toggleEditable(e, record.key, "removeheader")
}
>
编辑
</a>
<Divider type="vertical" />
<Popconfirm
title="是否要删除此行?"
onConfirm={() => this.handleDelete(record.key)}
okText="确定" cancelText="取消"
>
<a>删除</a>
</Popconfirm>
</span>
);
} else {
return (
<span>
<a
onClick={e => {
this.saveRow(e, record.key);
}}
>
保存
</a>
<Divider type="vertical" />
<Popconfirm
title="是否要删除此行?"
onConfirm={() => this.handleDelete(record.key)}
okText="确定" cancelText="取消"
>
<a>删除</a>
</Popconfirm>
</span>
);
}
}
}
];
return (
<Form >
<Form.Item label="目标系统" labelCol={{ span: 4 }}
wrapperCol={{ span: 14 }}
style={{ marginBottom: 10 }}
>
{getFieldDecorator('ip', {
initialValue: targetIp ? targetIp : undefined,
rules: [{ required: true, message: '请输入目标系统IP地址!' }]
})(<Input placeholder="请输入目标系统IP地址" />)}
</Form.Item>
<span className="tabletop">请求头配置</span>
<div >
<Table
className="target"
pagination={false}
columns={columns}
dataSource={dataSource}
/>
</div>
<Button
style={{ width: "100%", marginTop: 16, marginBottom: 8 }}
type="dashed"
onClick={() => {
this.handleAdd();
}}
icon="plus"
>
添加参数
</Button>
</Form>
);
}
}
export default Form.create()(TargetForm);
版本二
效果图(无保存按钮 antd4)
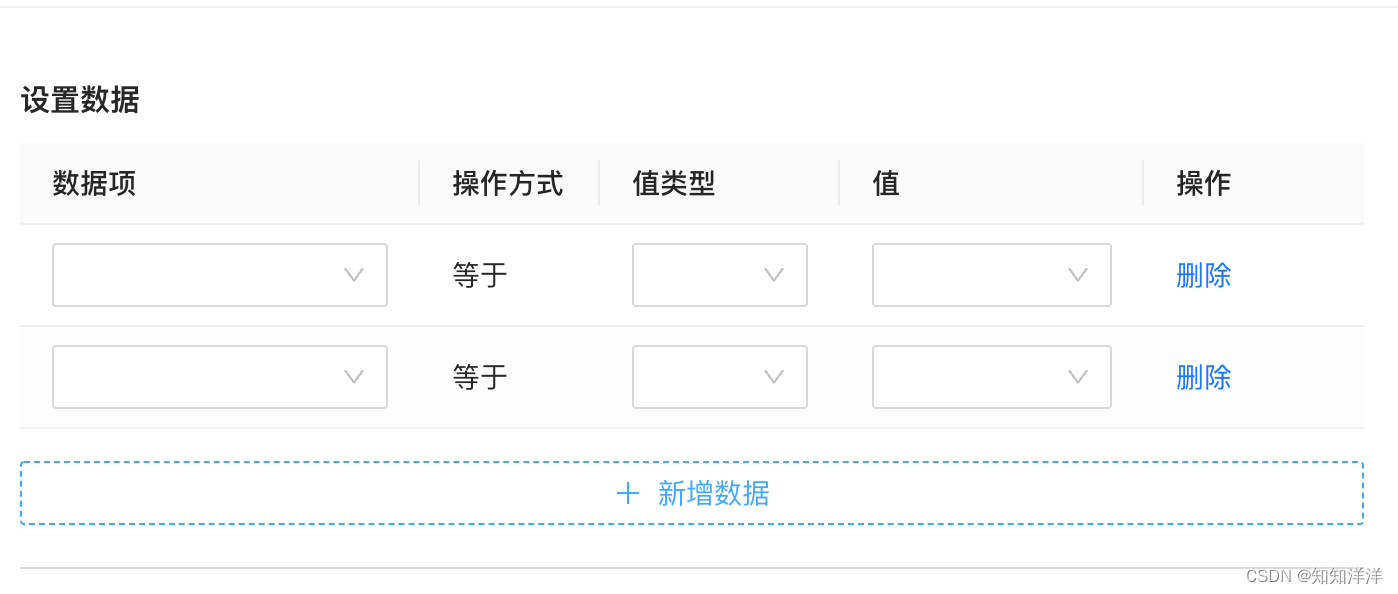
代码
import React, { Component } from 'react';
import { connect } from 'umi';
import styles from './index.less';
import { PlusOutlined } from '@ant-design/icons';
import {
Drawer,
Button, Form, Input, Table, Divider, Popconfirm, Tooltip, Select
} from 'antd';
class ActivationAfter extends Component {
formRef = React.createRef();
state = {
dataSource: [],
ValueList: [],
businessList: [],
valuetype: 0,
ActivationAfterData: this.props.ActivationAfterData,
lookType: true
};
componentDidMount() {
this.getModelList();
this.quertBusinessList()
this.echoData()
}
echoData = () => {
const { ActivationAfterData } = this.props;
console.log(ActivationAfterData);
if (ActivationAfterData.businessRuleIds || ActivationAfterData.dataInfos) {
this.formRef.current.setFieldsValue({
'businessRule': ActivationAfterData.businessRuleIds ? ActivationAfterData.businessRuleIds.split(',') : []
})
this.setState({
lookType: false,
dataSource: this.getdataSource(ActivationAfterData.dataInfos),
})
}
}
getdataSource = (obj) => {
let aa = []
for (let key in obj) {
let b = obj[key]
aa.push({
key: parseInt(this.randParamId()),
name: key,
type: b.type,
value: b.value,
})
}
return aa
}
getTypeList = (key) => {
const value = this.formRef.current.getFieldValue(`name_${key}`)
let valueList = []
if (value) {
const { propertyList } = this.props;
const typeName = propertyList.filter(item => {
return item.id == value
})[0].propertyTypeName
const list = propertyList.filter(item => {
return item.id !== value
})
valueList = list.filter(item => {
return item.propertyTypeName == typeName
})
}
return valueList
}
getModelList = () => {
const { dispatch } = this.props;
dispatch({
type: 'dataModel/fetch',
payload: {
modelId: window.sessionStorage.getItem('modelId'),
sortType: this.state.sortType,
},
})
};
quertBusinessList() {
const { dispatch } = this.props;
const modelId = window.sessionStorage.getItem('modelId');
const appId = window.sessionStorage.getItem('appId');
dispatch({
type: 'dataModel/queryBusinessList',
payload: {
modelId: modelId,
appId: appId,
},
callback: (res) => {
if (res.result && res.data.length !== 0) {
let businessList = []
businessList = res.data.map((item, index) => {
return {
key: index + 1,
ruleCold: item.ruleCode,
ruleName: item.ruleName,
ruleType: item.ruleType,
ruleId: item.ruleId,
defaultRule: item.defaultRule
}
})
sessionStorage.setItem('businessList', JSON.stringify(res.data))
this.setState({
businessList: businessList
})
}
}
})
}
onClose = () => {
this.props.noticeonCloseDrawer();
};
getStandDataInfos = (obj) => {
let aa = {}
let keyList = Object.keys(obj)
let nameList = keyList.filter(item => {
return item.indexOf('name') != -1
})
let list = []
nameList.forEach(
item => {
list.push(item.substr(5,))
}
)
list.forEach(item => {
aa[obj[`name_${item}`]] = {
type: obj[`type_${item}`],
value: obj[`value_${item}`]
}
}
)
return aa
}
saveParams = () => {
const { current } = this.formRef
current.validateFields().then((values) => {
const params = {
dataInfos: this.getStandDataInfos(values),
businessRuleIds: values.businessRule ? values.businessRule.join(',') : ''
}
this.props.getactiveAfterParams(params)
this.props.noticeonCloseDrawer();
});
};
randParamId = () => {
let num = '';
for (let i = 0; i < 13; i++) {
const val = parseInt(Math.random() * 10, 10);
if (i === 0 && val === 0) {
i--;
continue;
}
num += val;
}
return num;
};
getNameText = (value) => {
const { propertyList } = this.props;
let text = ''
const target = propertyList.filter(item => {
return item.id == value
})
text = target[0].name
return text
}
handleAdd = () => {
const { dataSource } = this.state;
const num = parseInt(this.randParamId())
const newData = {
key: num,
type: '',
value: '',
name: '',
};
this.setState({
dataSource: [...dataSource, newData],
lookType: true
});
};
handleDelete = (key) => {
const newData = [...this.state.dataSource];
this.setState({
dataSource: newData.filter((item) => item.key !== key)
});
};
handleFieldChange = (
e,
keyName,
key
) => {
let newData = [];
newData = [...this.state.dataSource];
const index = newData.findIndex(item => key === item.key);
if (keyName === 'value') {
newData[index][keyName] = e.target.value;
} else {
newData[index][keyName] = e;
}
this.setState({
dataSource: newData
});
};
handleNameChange = (value, formTypeName, formValueName) => {
this.formRef.current.setFieldsValue({ [(() => formTypeName)()]: undefined })
this.formRef.current.setFieldsValue({ [(() => formValueName)()]: undefined })
const { propertyList } = this.props;
const typeName = propertyList.filter(item => {
return item.id == value
})[0].propertyTypeName
const list = propertyList.filter(item => {
return item.id !== value
})
const valueList = list.filter(item => {
return item.propertyTypeName == typeName
})
this.setState({
ValueList: valueList
})
}
handleTypeChange = (value, formValueName) => {
this.formRef.current.setFieldsValue({ [(() => formValueName)()]: undefined })
this.setState({
valuetype: value
})
}
render() {
const { dataSource, ValueList, businessList, lookType } = this.state
const { propertyList } = this.props
const layout = {
labelCol: { span: 4 },
wrapperCol: { span: 12 },
};
const columns = [
{
title: '数据项',
dataIndex: 'name',
width: 200,
render: (text, record, index) => {
return (
<Form.Item
initialValue={text}
key={index}
name={`name_${record.key}`}
rules={[{ required: true, message: '请输入' }]}
>
{
propertyList ?
<Select
onChange={
(value) => {
this.handleNameChange(value, `type_${record.key}`, `value_${record.key}`)
}
}
placeholder="请选择"
>
{propertyList.map(item => (
<Select.Option key={item.id} >
{item.name}
</Select.Option>
))}
</Select> : ''
}
</Form.Item>
);
}
},
{
title: '操作方式',
dataIndex: 'mode',
width: 90,
render: (text, record, index) => {
return (
<span>等于</span>
);
}
},
{
title: '值类型',
dataIndex: 'type',
width: 120,
render: (text, record, index) => {
return (
<Form.Item
initialValue={text}
key={index}
name={`type_${record.key}`}
rules={[{ required: true, message: '请输入' }]}
>
<Select
onChange={(value) => {
this.handleTypeChange(value, `value_${record.key}`)
}}
placeholder="请选择"
>
<Select.Option value="fixed" >
固定值
</Select.Option>
<Select.Option value="dynamic" >
动态值
</Select.Option>
</Select>
</Form.Item>
);
}
},
{
title: '值',
dataIndex: 'value',
render: (text, record, index) => {
return (
<Form.Item
initialValue={text}
key={index}
name={`value_${record.key}`}
rules={[{ required: true, message: '请输入' }]}
>
{
lookType ?
(this.formRef.current.getFieldValue(`type_${record.key}`) == 'fixed' ?
<Input
autoFocus
onChange={e =>
this.handleFieldChange(e, 'value', record.key)
}
placeholder="请输入参数值"
/>
:
<Select
placeholder="请选择"
>
{this.getTypeList(record.key).map(item => (
<Select.Option key={item.id} >
{item.name}
</Select.Option>
))}
</Select>)
:
(record.type == 'fixed' ?
<Input
autoFocus
onChange={e =>
this.handleFieldChange(e, 'value', record.key)
}
placeholder="请输入参数值"
/>
:
<Select
placeholder="请选择"
>
{this.getTypeList(record.key).map(item => (
<Select.Option key={item.id} >
{item.name}
</Select.Option>
))}
</Select>)
}
</Form.Item>
);
}
},
{
title: '操作',
width: 110,
dataIndex: 'operation',
render: (text, record) => {
if (record.editable) {
return (
<span>
<Popconfirm
cancelText="取消"
okText="确定"
onConfirm={() => this.handleDelete(record.key)} title="是否要删除此行?"
>
<a>删除</a>
</Popconfirm>
</span>
);
} else {
return (
<span>
<Popconfirm
cancelText="取消"
okText="确定"
onConfirm={() => this.handleDelete(record.key)} title="是否要删除此行?"
>
<a>删除</a>
</Popconfirm>
</span>
);
}
}
}
];
return (
<Drawer
bodyStyle={{ paddingBottom: 80 }}
footer={
<div style={{ textAlign: 'center' }}>
<Button onClick={this.onClose} style={{ marginRight: 8 }}>
取消
</Button>
<Button onClick={this.saveParams} style={{ marginRight: '15px' }} type="primary">
保存
</Button>
</div>
}
onClose={() => this.onClose()}
placement="right"
title="自定义通知设置"
visible
width={720}
>
<Form ref={this.formRef}>
<span className={styles.contentTitle}> 设置数据</span>
<Table
className={styles.target}
columns={columns}
dataSource={dataSource}
pagination={false}
/>
<Button
icon={<PlusOutlined />}
onClick={() => {
this.handleAdd();
}}
style={{ width: '100%', marginTop: 16, marginBottom: 8 }}
type="dashed"
>
新增数据
</Button>
<div className={styles.contentTop} ></div>
<span className={styles.contentTitle}> 触发动作</span>
<Form.Item label="执行业务规则"
labelCol={{ span: 4 }}
name="businessRule"
wrapperCol={{ span: 14 }}
>
<Select
allowClear
mode="multiple"
placeholder="请选择"
>
{businessList.map(item => (
<Select.Option key={item.ruleId} >
{item.ruleName}
</Select.Option>
))}
</Select>
</Form.Item>
</Form>
</Drawer>
);
}
}
export default connect(({ dataModel: { propertyList, attrList } }) => ({ propertyList, attrList }))(ActivationAfter);