任务:在一个主线程中同时开启三个线程,每个线程中执行相同的数据插入动作,只是数据不同。
数据库在得到插入的响应后,作相应的处理,如果为数值1,延时10秒,为2延时5,为3不延时.
目的:体验C#多线程处理
后台数据库的淮备:
1.建表
create table dd (id Tinyint )
2.建触发器
create trigger tg_dd_ins on dd for insert
as
begin
declare @v Tinyint
select @v=id from inserted
if @v=1
waitfor delay '00:00:10'
else if @v=2
waitfor delay '00:00:05'
end
3.C#中处理过程
Form1.CS
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
using System.IO;
using System.Threading;
namespace threadprg
{
enum outputkind { otui, otfile };
public partial class Form1 : Form
{
private const string G_cnnstring = "Persist Security Info=False;User ID=user;Password=m258;Initial Catalog=test;Server=MSSQL";
private outputkind foutput;
outputkind Foutput
{
set { foutput = value; }
get { return foutput; }
}
public Form1()
{
InitializeComponent();
}
delegate void uidelegate();
private uidelegate d;
private void threadonedelegateexec()
{
if (this.InvokeRequired)
{
d = new uidelegate(threadonedelegateexec);
this.Invoke(d, null);
}
else
this.textBox1.Text = "thread one exec finished!";
}
private void threadtwodelegateexec()
{
if (this.InvokeRequired)
{
d = new uidelegate(threadtwodelegateexec);
this.Invoke(d,null);
}
else
this.textBox2.Text = "thread two exec finished!";
}
private void threadthreedelegateexec()
{
if (this.InvokeRequired)
{
d = new uidelegate(threadthreedelegateexec);
this.Invoke(d, null);
}
else
this.textBox3.Text = "thread three exec finished!";
}
private void thread1exec()
{
SqlConnection cnn = new SqlConnection(G_cnnstring);
cnn.Open();
SqlCommand cmd = new SqlCommand("insert into dd(id) values (1)");
cmd.Connection = cnn;
cmd.ExecuteNonQuery();
switch (foutput)
{
case outputkind.otui:
threadonedelegateexec();
break;
case outputkind.otfile:
WriteToAlertFile("threadonelog", "thread one exec finished!");
break;
default:
break;
}
cnn.Close();
}
private void thread2exec()
{
SqlConnection cnn = new SqlConnection(G_cnnstring);
cnn.Open();
SqlCommand cmd = new SqlCommand("insert into dd(id) values(2)");
cmd.Connection = cnn;
cmd.ExecuteNonQuery();
switch (foutput)
{
case outputkind.otui:
threadtwodelegateexec();
break;
case outputkind.otfile:
WriteToAlertFile("threadtwolog", "thread two exec finished!");
break;
default: break;
}
cnn.Close();
}
private void thread3exec()
{
SqlConnection cnn = new SqlConnection(G_cnnstring);
SqlCommand cmd = new SqlCommand("insert into dd(id) values(3)");
cnn.Open();
cmd.Connection = cnn;
cmd.ExecuteNonQuery();
switch (foutput)
{
case outputkind.otui:
threadthreedelegateexec();
break;
case outputkind.otfile:
WriteToAlertFile("threadthreeLog","Thread three exec finished!");
break;
default:
break;
}
cnn.Close();
}
private bool WriteToAlertFile(String xLogFileName, String xMessage)
{
return this.WriteToAlertFile(xLogFileName, xMessage, "D");
}
private bool WriteToAlertFile(String xLogFileName,String xMessage,String xFileType)
{
bool ret;
String mFileName,mRunPosition;
mRunPosition=Application.ExecutablePath+@"/log";
mRunPosition = @"c:/log";
try
{
if (!Directory.Exists(mRunPosition))
{
Directory.CreateDirectory(mRunPosition);
}
switch (xFileType)
{
case "D":
mFileName = mRunPosition + @"/Alert_" + xLogFileName + "_" + System.DateTime.Now.ToString("yyyyMMdd");
break;
case "M":
mFileName = mRunPosition + @"/Alert_" + xLogFileName + "_" + System.DateTime.Now.ToString("yyyyMM");
break;
case "Y":
mFileName = mRunPosition + @"/Alert_" + xLogFileName + "_" + System.DateTime.Now.ToString("yyyy");
break;
default:
mFileName = mRunPosition + @"/Alert_" + xLogFileName + "-" + System.DateTime.Now.ToString("YYYYMMDD");
break;
}
if (xLogFileName.IndexOf(".") == -1)
mFileName =mFileName+ ".log";
using(StreamWriter sw=new StreamWriter(mFileName))
{
sw.WriteLine(System.DateTime.Now.ToString(@"{yyyy/MM/dd HH:mm:ss}")+xMessage);
}
}
catch { ret=false; };
ret=true;
return ret;
}
private void Form1_Load(object sender, EventArgs e)
{
foutput = outputkind.otui;
//foutput = outputkind.otfile;
Thread T1 = new Thread(new ThreadStart(thread1exec));
T1.Start();
Thread T2 = new Thread(new ThreadStart(thread2exec));
T2.Start();
Thread T3 = new Thread(new ThreadStart(thread3exec));
T3.Start();
}
}
}
Form1.Designer.cs
namespace threadprg
{
partial class Form1
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.dataSet1 = new System.Data.DataSet();
((System.ComponentModel.ISupportInitialize)(this.dataSet1)).BeginInit();
this.SuspendLayout();
//
// button1
//
this.button1.Location = new System.Drawing.Point(180, 142);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(134, 43);
this.button1.TabIndex = 0;
this.button1.Text = "Go!!!";
this.button1.UseVisualStyleBackColor = true;
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(163, 31);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(160, 20);
this.textBox1.TabIndex = 1;
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(163, 67);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(160, 20);
this.textBox2.TabIndex = 2;
//
// textBox3
//
this.textBox3.Location = new System.Drawing.Point(163, 103);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(160, 20);
this.textBox3.TabIndex = 1;
//
// dataSet1
//
this.dataSet1.DataSetName = "NewDataSet";
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(534, 266);
this.Controls.Add(this.textBox3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.button1);
this.Name = "Form1";
this.Text = "Form1";
this.Load += new System.EventHandler(this.Form1_Load);
((System.ComponentModel.ISupportInitialize)(this.dataSet1)).EndInit();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Button button1;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Data.DataSet dataSet1;
}
}
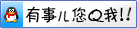