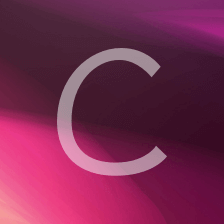
Linked List
文章平均质量分 68
dyllanzhou
这个作者很懒,什么都没留下…
展开
-
[Leetcode]Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head.For example,Given 1->2->3->4, you should return the list as 2->1->4->3.Your algorithm should use only constant space. You m原创 2015-10-01 12:39:56 · 254 阅读 · 0 评论 -
[Leetcode]Odd Even Linked List
Given a singly linked list, group all odd nodes together followed by the even nodes. Please note here we are talking about the node number and not the value in the nodes.You should try to do it in p原创 2016-01-16 20:55:51 · 362 阅读 · 0 评论 -
[Leetcode]Convert Sorted List to Binary Search Tree
Given a singly linked list where elements are sorted in ascending order, convert it to a height balanced BST./** * Definition for singly-linked list. * struct ListNode { * int val; * Lis原创 2015-10-12 22:03:00 · 285 阅读 · 0 评论 -
[Leetcode]Add Two Numbers
You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a link原创 2015-11-13 17:12:46 · 191 阅读 · 0 评论 -
[Leetcode]Reverse Linked List II
Reverse a linked list from position m to n. Do it in-place and in one-pass.For example:Given 1->2->3->4->5->NULL, m = 2 and n = 4,return 1->4->3->2->5->NULL.Note:Given m, n satisfy the follo原创 2015-10-27 21:09:10 · 647 阅读 · 0 评论 -
[Leetcode] Reorder List
Given a singly linked list L: L0→L1→…→Ln-1→Ln,reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→…You must do this in-place without altering the nodes' values.For example,Given {1,2,3,4}, reorder it to {1,4原创 2015-11-12 16:36:54 · 206 阅读 · 0 评论 -
[Leetcode] Linked List Cycle
Given a linked list, determine if it has a cycle in it.Follow up:Can you solve it without using extra space?class Solution {public: /*algorithm: hash solution time O(n) space O(n)原创 2015-09-16 11:09:06 · 290 阅读 · 0 评论 -
[Leetcode]Rotate List
Given a list, rotate the list to the right by k places, where k is non-negative.For example:Given 1->2->3->4->5->NULL and k = 2,return 4->5->1->2->3->NULL./** * Definition for singly-linked l原创 2015-11-11 21:43:49 · 217 阅读 · 0 评论 -
[Leetcode]Sort List
Sort a linked list in O(n log n) time using constant space complexity./** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : v原创 2015-11-09 23:26:44 · 185 阅读 · 0 评论 -
[Leetcode]Linked List Cycle II
Given a linked list, return the node where the cycle begins. If there is no cycle, returnnull.Note: Do not modify the linked list.Follow up:Can you solve it without using extra space?/**原创 2015-10-08 15:00:09 · 215 阅读 · 0 评论 -
[Leetcode]Insertion Sort List
Sort a linked list using insertion sort./** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; */原创 2015-10-23 13:47:20 · 255 阅读 · 0 评论 -
[Leetcode]Partition List
Given a linked list and a value x, partition it such that all nodes less thanx come before nodes greater than or equal to x.You should preserve the original relative order of the nodes in each of原创 2015-10-23 12:51:10 · 275 阅读 · 0 评论 -
[Leetcode]Remove Duplicates from Sorted List II
Given a sorted linked list, delete all nodes that have duplicate numbers, leaving onlydistinct numbers from the original list.For example,Given 1->2->3->3->4->4->5, return 1->2->5.Given 1->1->原创 2015-11-04 18:15:24 · 295 阅读 · 0 评论 -
[cc150]Chapter 3 | Stacks and Queues
Describe how you could use a single array to implement three stacks.1)solution 1 divide the array to 3 part, so each part can be one stack, but for each stack is limited to n/3 size [stk1][stk原创 2015-12-04 14:42:51 · 317 阅读 · 0 评论