一、Swagger介绍:
Swagger 是一个规范和完整的框架,用于生成、描述、调用和可视化
RESTful
风格的
Web
服务
(
https://swagger.io/
)
。
它的主要作用是:
1. 使得前后端分离开发更加方便,有利于团队协作
2. 接口的文档在线自动生成,降低后端开发人员编写接口文档的负担
3. 功能测试
Spring已经将
Swagger
纳入自身的标准,建立了
Spring-swagger
项目,现在叫
Springfox
。通过在 项目中引入Springfox
,即可非常简单快捷的使用
Swagger
。
注意:开发项目时候开启,上线后要关闭。
二、使用Swagger
1、引入依赖:
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
2、配置swagger的启动开关(谁使用谁配置):
# 开启swagger
swagger.enable=true
3、书写配置类:
@Configuration
@ConditionalOnProperty(prefix = "swagger",value = {"enable"},havingValue = "true")
@EnableSwagger2 //开启swagger注解支持
public class SwaggerConfiguration {
@Bean
public Docket buildDocket() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(buildApiInfo())
.select()
// 要扫描的API(Controller)基础包
.apis(RequestHandlerSelectors.basePackage("xx.xx.xx"))
.paths(PathSelectors.any())
.build();
}
private ApiInfo buildApiInfo() {
Contact contact = new Contact("文本内容","","");
return new ApiInfoBuilder()
.title("标题-用户服务API文档")
.description("包含用户服务api")
.contact(contact)
.version("1.0.0").build();
}
}
三、Swagger常用注解:
在Java
类中添加
Swagger
的注解即可生成
Swagger
接口文档,常用
Swagger
注解如下:
1、作用于类上。
@Api:修饰整个类,描述
Controller
的作用
@ApiOperation
:描述一个类的一个方法,或者说一个接 口
@ApiParam
:单个参数的描述信息
@ApiModel
:用对象来接收参数
@ApiModelProperty
:用对象接收参数时,描述对象的一个字段
@ApiResponse
:
HTTP
响应其中
1
个描述
@ApiResponses
:
HTTP
响应整体描述
@ApiIgnore
:使用该注解忽略这个
API
@ApiError
:发生错误返回的信息
@ApiImplicitParam
:一个请求参数
@ApiImplicitParams
:多个请求参数的描述信息
2、作用于方法上
@ApiImplicitParam
属性:
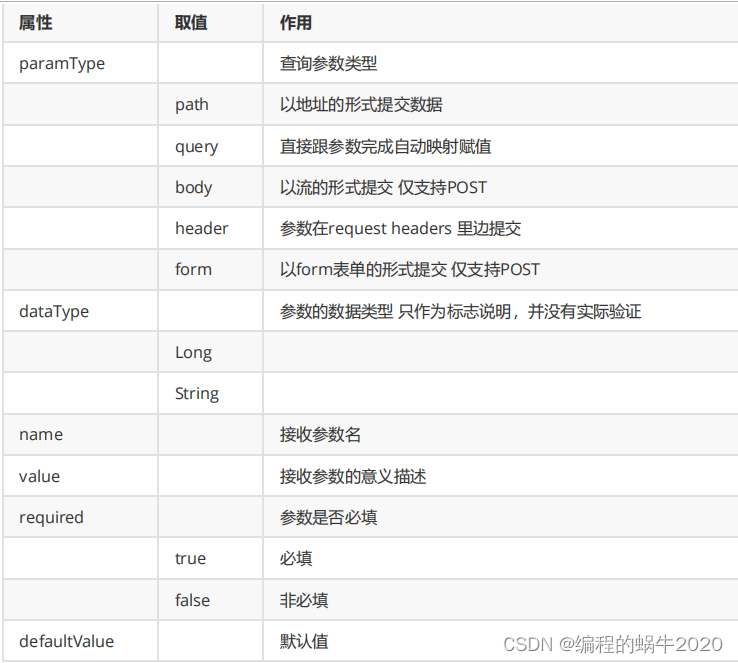
3、测试代码
@RestController
@Api(value = "用户服务的Controller", tags = "Consumer", description = "用户服务API")
public class ConsumerController {
@ApiOperation("测试hello")
@GetMapping(path = "/hello")
public String hello(){
return "hello";
}
@ApiOperation("测试hi")
@ApiImplicitParam(name="name",value="姓名",required = true,dataType = "String")
@PostMapping(value = "/hi")
public String hi(String name){
return "hi,"+name;
}
}
4、Swagger生成文档:
启动
xxx
服务工程,用浏览器访问:
http://localhost:53010/xxx
/swagger-ui.html
四、Swagger生成API文档的工作原理:
1
、
xxx工程
启动时会扫描到
SwaggerConfifiguration
类。
2
、在此类中指定了扫描包路径
xx.xx.xx
,会找到在此包下及子包下标记有@RestController注解的
controller
类。
3
、根据
controller
类中的
Swagger
注解生成
API
文档。