对象关系映射(英语:(Object Relational Mapping,简称ORM),是一种用于实现面向对象编程语言里不同类型系统的数据之间的转换。
利用java的反射与ORM思想实现数据的导出功能。
数据模型 ( 设置:数据起始行, 目标格式,模板路径)
import org.poi.excel.annotation.Column;
import org.poi.excel.annotation.Excel;
import org.poi.excel.annotation.Format;
/**
* @author Cheng.Wei
* @ClassName User
* @Description 用户信息
* @date 2018年4月10日
*
*/
@Excel(startRow = 1, format = Format.XLS, location = "D://123.xls")
public class User {
@Column(value = "B")
private String name;
@Column(value = "C")
private String sex;
@Column(value = "D")
private Integer age;
/**省略get set*/
}
执行程序
public static void main(String[] args) {
User user1 = new User();
user1.setName("张三");
user1.setAge(20);
user1.setSex("男士");
User user2 = new User();
user2.setName("李四");
user2.setAge(25);
user2.setSex("男士");
List<User> users = new ArrayList<>();
users.add(user1);
users.add(user2);
//导出表格
boolean result = new WriteImpl<>(User.class).write(users, "D://444.xls");
}
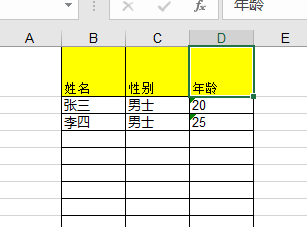
源码:
package org.poi.excel.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* @author Cheng.Wei
* @ClassName Excel
* @Description 数据模型注解
* @date 2018年4月10日
*/
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface Excel {
/**
* 数据起始行(默认第一行)
* @return
*/
int startRow() default 1;
/**
* 指定导出数据的模板存放路径
* @return
*/
String location();
/**
* Excel格式
* @return
*/
Format format() default Format.XLS;
}
package org.poi.excel.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* @author Cheng.Wei
* @ClassName Column
* @Description 属性映射到列
* @date 2018年4月10日
*
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Column {
/**
* Excel列映射 A,B,C ...
* @return
*/
String value();
}
package org.poi.excel.annotation;
/**
* @author Cheng.Wei
* @ClassName Excel
* @Description Excel文档类型
* @date 2018年4月10日
*
*/
public enum Format {
XLS, XLSX;
}
package org.poi.excel;
import java.util.List;
/**
* @author Cheng.Wei
* @ClassName Write
* @Description 写数据
* @date 2018年4月10日
*
*/
public interface Write<T> {
/**
* 写入
* @param data 数据
* @param location 文件输出路径
* @return
*/
boolean write(List<T> data, String location);
}
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.lang.reflect.Field;
import java.util.List;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.poi.excel.annotation.Column;
import org.poi.excel.annotation.Excel;
import org.poi.excel.annotation.Format;
/**
* @author Cheng.Wei
* @ClassName WriteImpl
* @Description 写数据
* @date 2018年4月10日
*
*/
public class WriteImpl<T> implements Write<T> {
private Class<T> clazz;
public WriteImpl(Class<T> clazz) {
this.clazz = clazz;
}
/**
* @see org.poi.excel.Write#write(java.util.List, java.lang.String)
*/
@Override
public boolean write(List<T> data, String location) {
Excel excel = clazz.getAnnotation(Excel.class);
//判断目标转换格式
if (excel.format().equals(Format.XLS)) {
return hssf(data, excel, location);
} else {
return xssf(data, excel, location);
}
}
/**
* 对XLS格式文档写入值
* @param data
* @param excel
* @param location
* @return
*/
boolean hssf(List<T> data, Excel excel, String location) {
Workbook wb = null;
Sheet sheet = null;
try {
wb = new HSSFWorkbook(new FileInputStream(excel.location()));
sheet = wb.getSheetAt(0);
} catch (IOException e) {
e.printStackTrace();
}
int startRow = excel.startRow();
for (T t : data) {
Field[] fields = t.getClass().getDeclaredFields();
sheet.createRow(startRow);
for (Field field : fields) {
if (!field.isAccessible()) {
field.setAccessible(true);
}
Column column = field.getAnnotation(Column.class);
try {
sheet.getRow(startRow).createCell(getCol(column.value()))
.setCellValue(field.get(t) == null ? "" : String.valueOf(field.get(t)));
} catch (IllegalAccessException e) {
e.printStackTrace();
return false;
}
}
startRow++;
}
try (OutputStream output = new FileOutputStream(location)) {
output.flush();
wb.write(output);
output.close();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
/**
* 对XLSX格式文档写入值
* @param data
* @param excel
* @param location
* @return
*/
boolean xssf(List<T> data, Excel excel, String location) {
Workbook wb = null;
Sheet sheet = null;
try{
wb = new XSSFWorkbook(new FileInputStream(excel.location()));
sheet = wb.getSheetAt(0);
} catch (IOException e) {
e.printStackTrace();
}
int startRow = excel.startRow();
for (T t : data) {
Field[] fields = t.getClass().getDeclaredFields();
sheet.createRow(startRow);
for (Field field : fields) {
if (!field.isAccessible()) {
field.setAccessible(true);
}
Column column = field.getAnnotation(Column.class);
try {
sheet.getRow(startRow).createCell(getCol(column.value()))
.setCellValue(field.get(t) == null ? "" : String.valueOf(field.get(t)));
} catch (IllegalAccessException e) {
e.printStackTrace();
return false;
}
}
startRow++;
}
try (OutputStream output = new FileOutputStream(location)) {
output.flush();
wb.write(output);
output.close();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
/**
* 将EXCEL中A,B,C...列映射成0,1,2...
*
* @param col
*/
protected static int getCol(String col) {
int count = -1;
col = col.toUpperCase();
char[] cs = col.toCharArray();
for (int i = 0, length = cs.length; i < length; i++) {
count += (cs[i] - 64) * Math.pow(26, cs.length - 1 - i);
}
return count;
}
}