ToolBar
package com.example.framework.view;
import android.content.Context;
import android.content.res.TypedArray;
import android.util.AttributeSet;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.RelativeLayout;
import android.widget.TextView;
import com.example.framework.R;
public class ToolBar extends RelativeLayout {
private TextView titleTv;
private ImageView leftImg;
private ImageView rightImg;
private boolean rightAreaIsShow, leftIsShow;
private String titleText;
private int rightImgId, leftImgId;
private IToolbarListener iToolbarListener;
private String rightText;
private TextView rightTv;
public ToolBar(Context context) {
super(context);
init(context, null, 0);
}
public ToolBar(Context context, AttributeSet attrs) {
super(context, attrs);
init(context, attrs, 0);
}
public ToolBar(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init(context, attrs, defStyleAttr);
}
private void init(Context context, AttributeSet attrs, int defStyleAttr) {
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.ToolBar);
titleText = typedArray.getString(R.styleable.ToolBar_titleText);
leftImgId = typedArray.getResourceId(R.styleable.ToolBar_leftImg, 0);
rightImgId = typedArray.getResourceId(R.styleable.ToolBar_rightImage, 0);
leftIsShow = typedArray.getBoolean(R.styleable.ToolBar_leftIsShow, false);
rightAreaIsShow = typedArray.getBoolean(R.styleable.ToolBar_rightIsShow, false);
rightText = typedArray.getString(R.styleable.ToolBar_rightText);
typedArray.recycle();
LayoutInflater inflater = LayoutInflater.from(context);
inflater.inflate(R.layout.view_toolbar, this);
titleTv = findViewById(R.id.titleTv);
leftImg = findViewById(R.id.leftImg);
rightImg = findViewById(R.id.rightImg);
rightTv = findViewById(R.id.rightTv);
titleTv.setText(titleText);
rightTv.setText(rightText);
if (rightAreaIsShow && rightImgId != 0) {
rightImg.setImageResource(rightImgId);
}
if (leftIsShow && leftImgId != 0) {
leftImg.setImageResource(leftImgId);
}
leftImg.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
if (iToolbarListener != null) {
iToolbarListener.onLeftClick();
}
}
});
rightImg.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
if (iToolbarListener != null) {
iToolbarListener.onRightImgClick();
}
}
});
rightTv.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
if (iToolbarListener != null) {
iToolbarListener.onRightTvClick();
}
}
});
}
public void setToolbarListener(IToolbarListener iToolbarListener) {
this.iToolbarListener = iToolbarListener;
}
public static interface IToolbarListener {
void onLeftClick();
void onRightImgClick();
void onRightTvClick();
}
}
布局
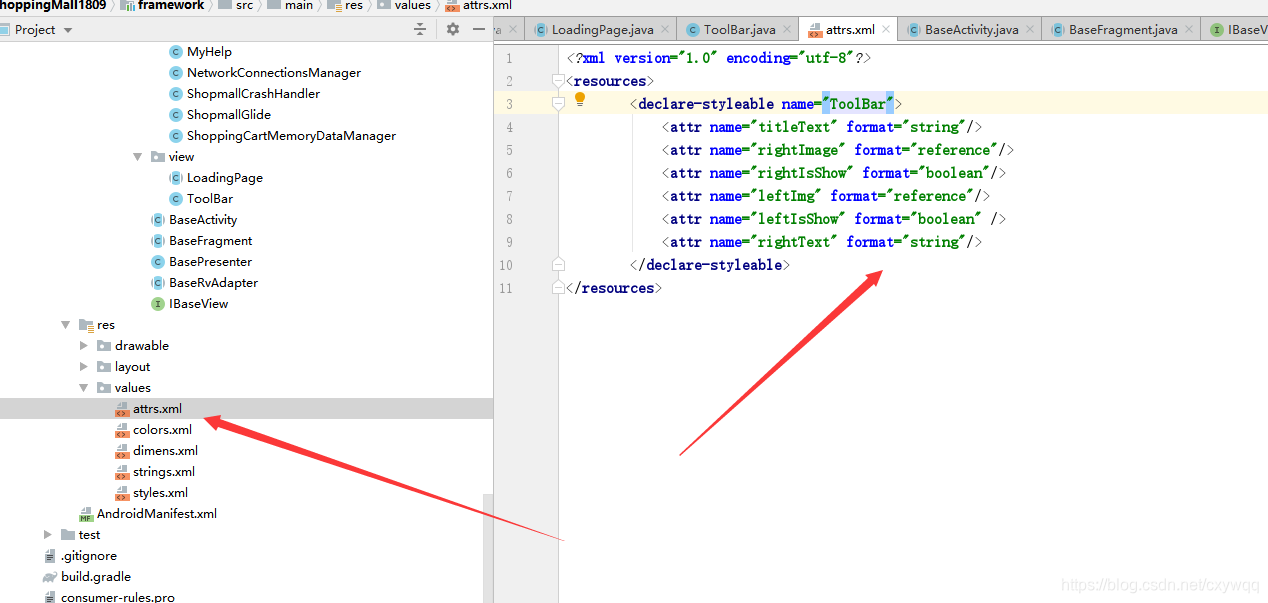
toolbar页面
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/leftImg"
android:layout_width="@dimen/toolbar_img_size"
android:layout_height="@dimen/toolbar_img_size"
android:layout_marginLeft="@dimen/toolbar_margin_size"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
></ImageView>
<TextView
android:id="@+id/titleTv"
style="@style/bigTextStyle"
android:layout_centerInParent="true"
></TextView>
<LinearLayout
android:id="@+id/rightArea"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="@dimen/toolbar_margin_size"
android:layout_alignParentRight="true"
android:orientation="horizontal"
>
<RelativeLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
>
<ImageView
android:layout_width="@dimen/toolbar_img_size"
android:layout_height="@dimen/toolbar_img_size"
android:id="@+id/rightImg"
android:layout_centerVertical="true"
></ImageView>
</RelativeLayout>
<RelativeLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
>
<TextView
android:id="@+id/rightTv"
style="@style/midTextStyle"
android:layout_centerVertical="true"
android:layout_marginTop="@dimen/dp_30"
></TextView>
</RelativeLayout>
</LinearLayout>
</RelativeLayout>
布局
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<com.example.framework.view.ToolBar
android:layout_width="match_parent"
android:layout_height="@dimen/dp_60"
android:id="@+id/toolbar">
</com.example.framework.view.ToolBar>
</LinearLayout>