文章目录
- [2409. 统计共同度过的日子数](https://leetcode.cn/problems/count-days-spent-together/)
- [141. 环形链表](https://leetcode.cn/problems/linked-list-cycle/description/)
- [876. 链表的中间结点](https://leetcode.cn/problems/middle-of-the-linked-list/description/)
- [142. 环形链表 II](https://leetcode.cn/problems/linked-list-cycle-ii/description/)
- [剑指 Offer 22. 链表中倒数第k个节点](https://leetcode.cn/problems/lian-biao-zhong-dao-shu-di-kge-jie-dian-lcof/description/)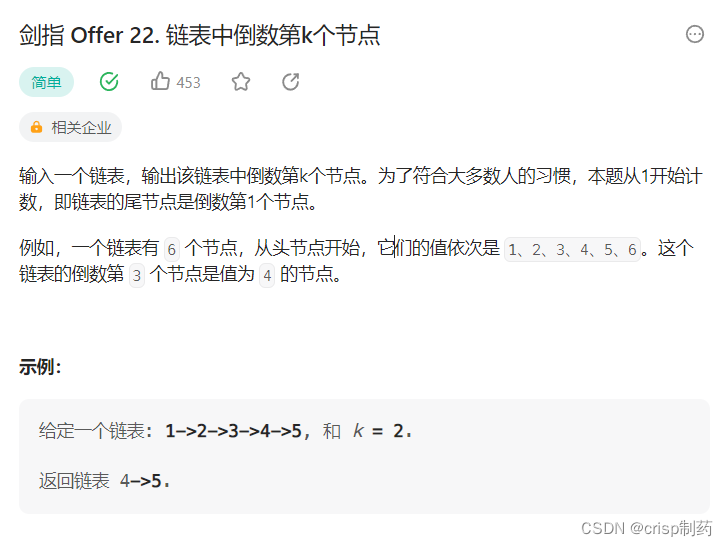
2409. 统计共同度过的日子数
class Solution {
int days[] = new int [] {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
public int countDaysTogether(String arriveAlice, String leaveAlice, String arriveBob, String leaveBob) {
String a = arriveAlice.compareTo(arriveBob)<0?arriveBob:arriveAlice;
String b = leaveAlice.compareTo(leaveBob)<0?leaveAlice:leaveBob;
int x = f(a),y=f(b);
return Math.max(y-x+1,0);
}
private int f(String s){
int i = Integer.parseInt(s.substring(0,2))-1;
int res = 0;
for(int j = 0;j<i;j++){
res+=days[j];
}
res += Integer.parseInt(s.substring(3));
return res;
}
}
141. 环形链表
这里我们使用快慢指针来解决这道题,当链表中有环时,那么快慢指针必定会相遇,如果没有环则不会相遇,
public class Solution {
public boolean hasCycle(ListNode head) {
ListNode s = head, f = head;
while(f != null && f.next != null){
s = s.next;
f = f.next.next;
if(s == f) return true;
}
return false;
}
}
876. 链表的中间结点
这题依旧是使用快慢指针,一个走一步一个走两步,快指针停止循环的条件为当前节点和下一个节点不为空,当快指针停下时,此时slow指针的位置就是目标位置。
142. 环形链表 II
public class Solution {
public ListNode detectCycle(ListNode head) {
ListNode fast = head, slow = head;
while (true) {
if (fast == null || fast.next == null) return null;
fast = fast.next.next;
slow = slow.next;
if (fast == slow) break;
}
fast = head;
while (slow != fast) {
slow = slow.next;
fast = fast.next;
}
return fast;
}
}
剑指 Offer 22. 链表中倒数第k个节点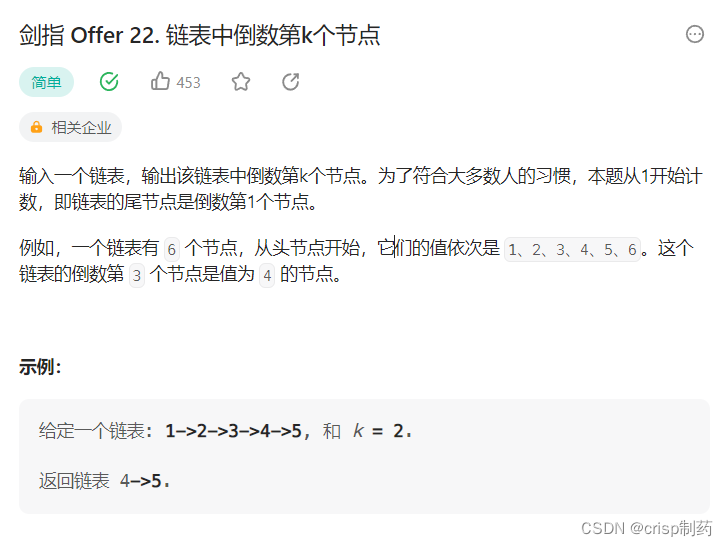
先让快指针移动k步,然后再让快慢指针都一步一步前进,当快指针移动到空值时,此时慢指针离尾结点距离为k-1,也就是倒数第k个节点的位置了
class Solution {
public ListNode getKthFromEnd(ListNode head, int k) {
ListNode former = head, latter = head;
for(int i = 0; i < k; i++)
former = former.next;
while(former != null) {
former = former.next;
latter = latter.next;
}
return latter;
}
}
链表大都是使用快慢指针来进行解题,然后根据不同题目来确定while循环结束的条件