问题

代码
主程序
package Chapter3;
public class Three_Four {
public static void main(String[] args) {
MyArrayList<Integer> list1 = new MyArrayList<>();
list1.add(1);
list1.add(3);
list1.add(5);
list1.add(7);
list1.add(9);
MyArrayList<Integer> list2 = new MyArrayList<>();
list2.add(1);
list2.add(2);
list2.add(5);
list2.add(7);
list2.add(8);
list2.add(9);
MyArrayList<Integer> mergeList;
mergeList = JiaoJi(list1, list2);
System.out.println("交集");
for(int i=0; i<mergeList.size(); i++) {
System.out.print(mergeList.get(i));
}
System.out.println();
mergeList = BingJi(list1, list2);
System.out.println("并集");
for(int i=0; i<mergeList.size(); i++) {
System.out.print(mergeList.get(i));
}
System.out.println();
}
private static MyArrayList<Integer> JiaoJi(MyArrayList<Integer> list1, MyArrayList<Integer> list2) {
MyArrayList<Integer> mergeList = new MyArrayList<>();
int index1 = 0;
int index2 = 0;
int v1, v2;
while(index1 < list1.size() && index2 < list2.size()) {
v1 = list1.get(index1);
v2 = list2.get(index2);
if(v1 < v2) {
index1++;
}else if(v1 > v2) {
index2++;
}else {
mergeList.add(v1);
index1++;
index2++;
}
}
return mergeList;
}
private static MyArrayList<Integer> BingJi(MyArrayList<Integer> list1, MyArrayList<Integer> list2) {
MyArrayList<Integer> mergeList = new MyArrayList<>();
int index1 = 0;
int index2 = 0;
int v1, v2;
while(index1 < list1.size() && index2 < list2.size()) {
v1 = list1.get(index1);
v2 = list2.get(index2);
if(v1 < v2) {
mergeList.add(v1);
index1++;
}else if(v1 > v2) {
mergeList.add(v2);
index2++;
}else {
mergeList.add(v1);
index1++;
index2++;
}
}
if(index1 == list1.size()) {
for(; index2<list2.size(); index2++) {
v2 = list2.get(index2);
mergeList.add(v2);
}
}
if(index2 == list2.size()) {
for(; index1<list1.size(); index1++) {
v1 = list1.get(index1);
mergeList.add(v1);
}
}
return mergeList;
}
}
MyArrayList
package Chapter3;
import java.util.Iterator;
public class MyArrayList<AnyType> implements Iterable<AnyType> {
private static final int DEFAULT_CAPACITY = 10;
private int theSize;
private AnyType[] theItems;
public MyArrayList() {
doClear();
}
public void clear() {
clear();
}
public int size() {
return theSize;
}
public boolean isEmpty() {
return size()==0;
}
public void trimToSize() {
ensureCapacity(size());
}
public AnyType get(int idx) {
if(idx < 0 || idx >= size()) {
throw new ArrayIndexOutOfBoundsException();
}
return theItems[idx];
}
public AnyType set(int idx, AnyType newVal) {
if(idx < 0 || idx >= size()) {
throw new ArrayIndexOutOfBoundsException();
}
AnyType old = theItems[idx];
theItems[idx] = newVal;
return old;
}
public boolean add(AnyType x) {
add(size(), x);
return true;
}
public void add(int idx, AnyType x) {
if(theItems.length == size()) {
ensureCapacity(size()*2+1);
}
for(int i = theSize; i>idx; i--) {
theItems[i] = theItems[i-1];
}
theItems[idx] = x;
theSize++;
}
public AnyType remove(int idx) {
AnyType removedItem = theItems[idx];
for(int i=idx; i<size()-1; i++) {
theItems[i] = theItems[i++];
}
theSize--;
return removedItem;
}
private void doClear() {
theSize = 0;
ensureCapacity(DEFAULT_CAPACITY);
}
private void ensureCapacity(int newCapacity) {
if(newCapacity < theSize) {
return;
}
AnyType[] old = theItems;
theItems = (AnyType [])new Object[newCapacity];
for(int i=0; i< size(); i++) {
theItems[i] = old[i];
}
}
public java.util.Iterator<AnyType> iterator(){
return new ArrayListIterator();
}
private class ArrayListIterator implements java.util.Iterator<AnyType>{
private int current = 0;
@Override
public boolean hasNext() {
return current<size();
}
@Override
public AnyType next() {
if(!hasNext())
throw new java.util.NoSuchElementException();
return theItems[current++];
}
public void remove() {
MyArrayList.this.remove(--current);
}
}
}
结果
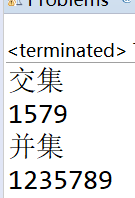