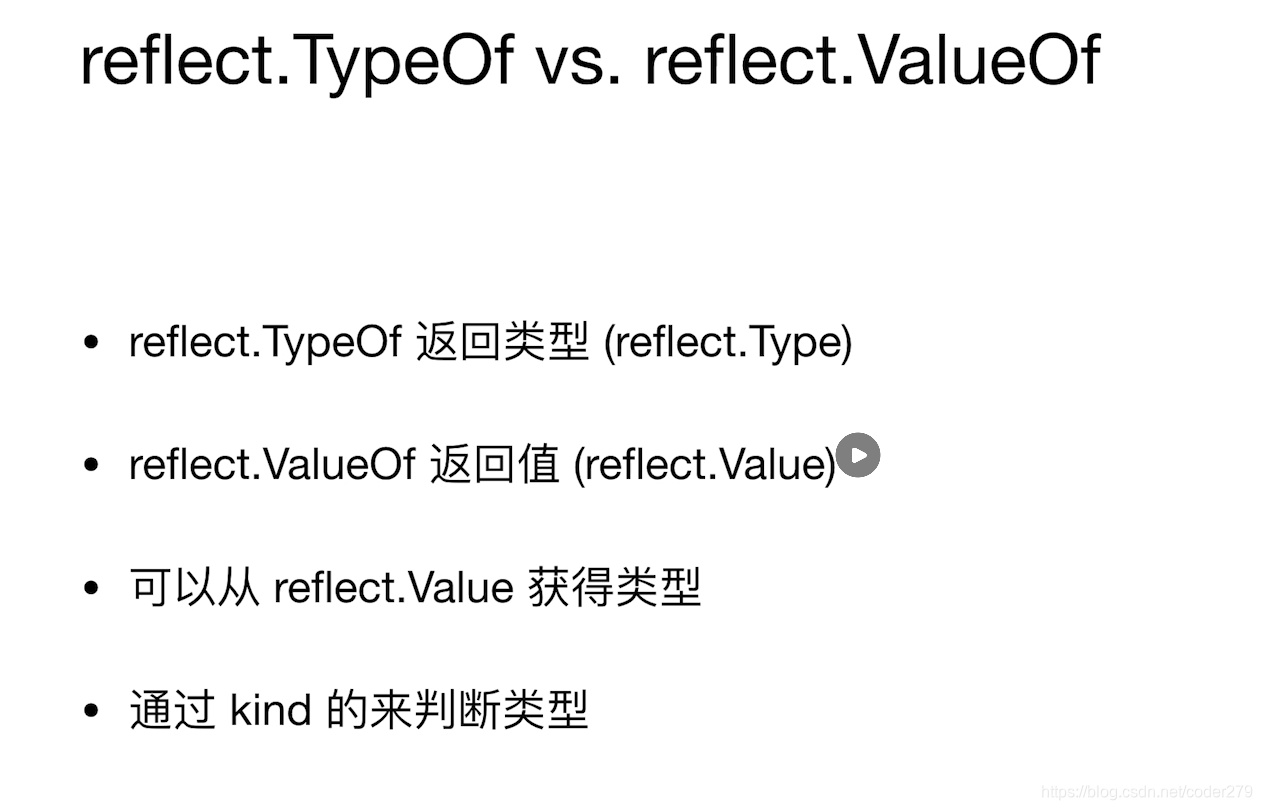
package reflect
import (
"fmt"
"reflect"
"testing"
)
func CheckType(v interface{}){
t := reflect.TypeOf(v)
switch t.Kind() {
case reflect.Float32,reflect.Float64:
fmt.Println("Float")
case reflect.Int,reflect.Int64:
fmt.Println("Int")
default:
fmt.Println("Unknow")
}
}
func TestBasicType(t *testing.T){
var v float64 = 12
CheckType(v)
}
func TestTypeAndValue(t *testing.T){
var f int64 = 10
t.Log(reflect.TypeOf(f),reflect.ValueOf(f))
t.Log(reflect.ValueOf(f).Type())
}
type Employ struct {
EmployeeID string
Name string `format:"nomal"`
Age int
}
func (e *Employ) UpdateAge(newVal int){
e.Age = newVal
}
type Customer struct {
CookieId string
Name string
Age int
}
func TestInvokeByName(t *testing.T){
e := &Employ{"1","Mike",30}
t.Logf("Name: value(%[1]v),Type(%[1]T)",reflect.ValueOf(*e).FieldByName("Name"))
if nameField,ok := reflect.TypeOf(*e).FieldByName("Name");!ok{
t.Error("Failed to get Name filed")
}else{
t.Log("Tag:format",nameField.Tag.Get("format"))
}
reflect.ValueOf(e).MethodByName("UpdateAge").Call([]reflect.Value{reflect.ValueOf(1)})
t.Log("Updated Age:",e)
}