效果图
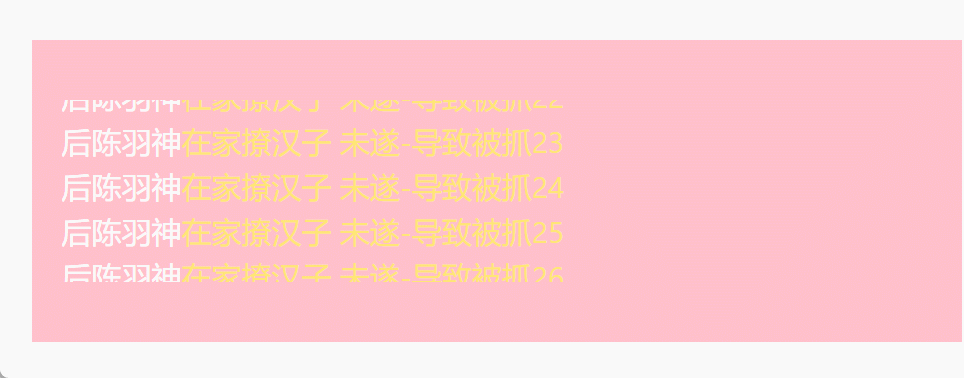
JS
import { useState, useEffect, useRef, Fragment } from 'react';
import styles from './style.less'
const index = () =>
{
const [data, setData] = useState(Array(30).fill(1))
const [hovered, setHovered] = useState(false)
const [listPanelStyle, setListPanelStyle] = useState(0)
const [noRoll, setNoRoll] = useState(false)
const [timer, setTimer] = useState(null)
const panelRef = useRef()
useEffect(() =>
{
hovered ? stopSlide() : startSlide()
return (() =>
{
clearInterval(timer)
})
}, [hovered])
const startSlide = () =>
{
let s = .6
, c = 1e3 / 60
let e = panelRef.current
, t = e.clientHeight
, i = e.parentElement.clientHeight;
let panelTop = listPanelStyle
let timeBomb = null
i < t ? timeBomb = setInterval(function ()
{
t / 2 + panelTop > 0 ? panelTop -= s : panelTop = 0,
setListPanelStyle(panelTop)
}, c) : setNoRoll({
noRoll: !0
})
setTimer(timeBomb)
}
const stopSlide = () =>
{
clearInterval(timer)
}
const mouseEnterHandler = () =>
{
setHovered(true)
}
const mouseLeaveHandler = () =>
{
setHovered(false)
}
const ScrollWarp = () =>
{
let dom = null
dom = ['小', '川'].map((d, i) => (
<Fragment key={i}>
{WarpDom()}
</Fragment>
))
return dom
}
const WarpDom = () =>
{
let dom = null
dom = data.map((data, index) => (
<p key={index} className={styles.list} >
<span style={{ color: 'rgb(255,255,255)', fontSize: '20px', lineHeight: '1.5' }}>后陈</span>
<span style={{ color: 'rgb(255,255,255)', fontSize: '20px', lineHeight: '1.5' }}>羽神</span>
<span style={{ color: 'rgb(255,230,116)', fontSize: '20px', lineHeight: '1.5' }}>在家</span>
<span style={{ color: 'rgb(255,230,116)', fontSize: '20px', lineHeight: '1.5' }}>撩汉子 未遂-导致被抓{index}</span>
</p>
))
return dom
}
return (
<div className={styles.container} >
<div className={styles.wrap} >
<div onMouseEnter={mouseEnterHandler} onMouseLeave={mouseLeaveHandler} ref={panelRef} style={{ top: listPanelStyle }} className={styles.panel} >
{!noRoll ? ScrollWarp() : WarpDom()}
</div>
</div>
</div>
);
};
export default index
CSS
.container {
background-color: pink;
background-attachment: scroll;
background-size: contain;
background-position: center top;
background-repeat: no-repeat;
margin-right: auto;
vertical-align: top;
padding-bottom: 40px;
top: 200px;
// left: -92px;
left: 91px;
width: 620px;
position: absolute;
padding-top: 40px;
padding-left: 20px;
height: 201px;
margin-left: auto;
margin: 0 auto;
}
.wrap {
position: relative;
height: 100%;
overflow: hidden;
}
.panel {
position: relative;
}
.list {
margin: 0;
}
.list span {
display: inline-block;
font-size: 20px;
line-height: 1.5;
}