(1)创建数据表 People
CREATETABLE `people` (
`id` int(10) NOTNULL AUTO_INCREMENT,
`name` varchar(20) DEFAULT NULL,
`age` int(10) DEFAULT NULL,
`height` double(10,2) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=11 DEFAULT CHARSET=gb2312
(2)创建实体类People
@Data//有这个注解就不用写get、set、toString方法了,前提是要引入lombok依赖
public class People {
private String name;
private Integer id;
private Integer age;
private Double height;
}
(3)controller层代码
@RestController
@RequestMapping("/people")
public class PeopleController{
@Autowired
private PeopleService peopleService;
/**
* 批量添加数据
* @param people
* @return int返回形式可以自己定
*/
@PostMapping("/addList")
public int addList(@RequestBody List<People> people){
return peopleService.addList(people);
}
}
(4)service层代码
/**
* @author xxx
* @version $Id: PeopleService.java, v 0.1 2022-07-11 14:51
*/
public interface PeopleService {
int addList(List<People> people);
}
/**
* @author xxx
* @version $Id: PeopleServiceImpl.java, v 0.1 2022-07-11 14:52
*/
@Service
public class PeopleServiceImpl implements PeopleService {
@Resource
private PeopleMapper peopleMapper;
@Override
public int addList(List<People> people) {
return peopleMapper.addList(people);
}
}
(5)Mapper层代码
/**
* @author xxx
* @version $Id: PeopleMapper.xml.java, v 0.1 2022-07-11 14:54
*/
@Mapper
public interface PeopleMapper {
int addList(@Param("list") List<People> people);
}
(6)SQL语句
<insert id="addList">
insert into people(id,name,age,height)
values
<foreach collection="list" item="item" open="(" separator="),(" close=")">
#{item.id},#{item.name},#{item.age},#{item.height}
</foreach>
</insert>
(7)postman自测(注意入参json格式)
[{
"id": 10,
"name": "张三",
"age": "22",
"height": "185"
},{
"id": 9,
"name": "李四",
"age": "24",
"height": "188"
}]
(8)结果与演示
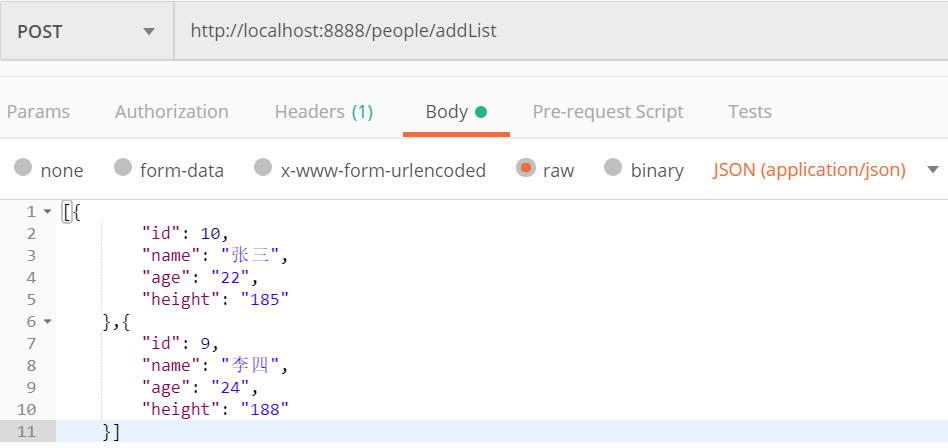
结果:
