c语言双向链表练习
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Node
{
int data;
struct Node *pNext;
struct Node *Pre;
}pNode,*ListNode;
struct Node *Create_Node(int dat)
{
struct Node *pHead = (struct Node *)malloc(sizeof(pHead));
if(NULL == pHead)
{
return NULL;
}
memset(pHead,'\0',sizeof(pHead));
pHead->data = dat;
pHead->pNext = NULL;
pHead->Pre = NULL;
return pHead;
}
struct Node *InsertNodeByHead(ListNode pHead,ListNode New)
{
if(NULL == pHead || NULL == New)
{
return NULL;
}
New->pNext = pHead->pNext;
if(NULL != pHead->pNext)
{
pHead->pNext->Pre = New;
}
New->Pre = pHead;
pHead->pNext = New;
return pHead;
}
struct Node *InsertNodeByTail(ListNode pHead,ListNode New)
{
struct Node *Temp = pHead;
while(NULL != Temp->pNext)
{
Temp = Temp->pNext;
}
New->pNext = Temp->pNext;
New->Pre = Temp;
Temp->pNext = New;
return Temp;
}
struct Node *FindNodeByData(ListNode pHead,int dat)
{
struct Node *Temp = pHead;
while(NULL != Temp->pNext)
{
Temp = Temp->pNext;
if(Temp->data == dat)
{
return Temp;
}
}
return NULL;
}
int DeleteNodeByData(ListNode pHead,int dat)
{
struct Node *Temp = FindNodeByData(pHead,dat);
if(NULL == Temp)
{
return 0;
}
Temp->Pre->pNext = Temp->pNext;
if(NULL != Temp->pNext)
{
Temp->pNext->Pre = Temp->Pre;
}
free(Temp);
return 1;
}
void PrintNode(ListNode pHead)
{
struct Node *Temp = pHead;
int num = 1;
while(NULL != Temp->pNext)
{
Temp = Temp->pNext;
printf("node %d:%d\n",num,Temp->data);
num++;
}
}
int main(void)
{
ListNode pH = NULL;
pH = Create_Node(0);
InsertNodeByHead(pH,Create_Node(12));
InsertNodeByHead(pH,Create_Node(3));
InsertNodeByHead(pH,Create_Node(45));
InsertNodeByTail(pH,Create_Node(23));
InsertNodeByTail(pH,Create_Node(5));
InsertNodeByTail(pH,Create_Node(67));
PrintNode(pH);
printf("--------------删除结点后------------\n");
DeleteNodeByData(pH,67);
PrintNode(pH);
return 0;
}
运行结果
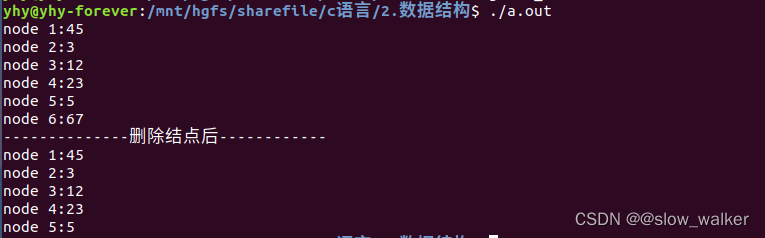