c语言单链表练习
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Node
{
int data;
struct Node *pNext;
}pNode,*ListNode;
struct Node *Create_Node(int dat)
{
struct Node *pHead = (struct Node *)malloc(sizeof(pHead));
if(NULL == pHead)
{
return NULL;
}
memset(pHead,'\0',sizeof(pHead));
pHead->data = dat;
pHead->pNext = NULL;
return pHead;
}
struct Node *InsertNodeByHead(ListNode pHead,ListNode New)
{
New->pNext = pHead->pNext;
pHead->pNext = New;
}
struct Node *InsertNodeByTail(ListNode pHead,ListNode New)
{
struct Node *Temp = pHead;
while(NULL != Temp->pNext)
{
Temp = Temp->pNext;
}
Temp->pNext = New;
return Temp;
}
struct Node *InsertNodeByAppointBefore(ListNode pHead,int index,ListNode New)
{
struct Node *Temp = pHead;
int j = 0;
while((NULL != Temp->pNext) && (j < index -1))
{
Temp = Temp->pNext;
j++;
}
if(NULL == Temp)
{
return NULL;
}
return InsertNodeByHead(Temp,New);
}
struct Node *InsertNodeByAppointafter(ListNode pHead,int index,ListNode New)
{
struct Node *Temp = pHead;
int j = 0;
while((NULL != Temp->pNext) && (index -1) > 0)
{
Temp = Temp->pNext;
index--;
}
if(NULL == Temp)
{
return NULL;
}
New->pNext = Temp->pNext;
Temp->pNext = New;
return pHead;
}
struct Node *FindNodeByData(ListNode pHead,int dat)
{
struct Node *Temp = pHead;
while(NULL != Temp->pNext)
{
Temp = Temp->pNext;
if(Temp->data == dat)
{
return Temp;
}
}
return NULL;
}
int DeleteNodeByData(ListNode pHead,int dat)
{
struct Node *Temp = pHead;
struct Node *PreTemp = NULL;
while(NULL != Temp->pNext)
{
PreTemp = Temp;
Temp = Temp->pNext;
if(Temp->data == dat)
{
if(NULL == Temp->pNext)
{
PreTemp->pNext = NULL;
}
else{
PreTemp->pNext = Temp->pNext;
}
free(Temp);
return 1;
}
}
return 0;
}
void PrintNodeData(struct Node *pHead)
{
int num = 1;
struct Node *Temp = pHead->pNext;
while(NULL != Temp)
{
printf("Node %d:%d\n",num,Temp->data);
num++;
Temp = Temp->pNext;
}
}
void NodeBubble(ListNode pHead)
{
struct Node *Temp = pHead->pNext;
struct Node *PreTemp = pHead;
int t;
while(NULL != Temp)
{
PreTemp = Temp->pNext;
while(NULL != PreTemp)
{
if(Temp->data > PreTemp->data)
{
t = Temp->data;
Temp->data = PreTemp->data;
PreTemp->data = t;
}
PreTemp = PreTemp->pNext;
}
Temp = Temp->pNext;
}
}
int main(void)
{
ListNode pH;
pH = Create_Node(0);
InsertNodeByHead(pH,Create_Node(10));
InsertNodeByHead(pH,Create_Node(40));
InsertNodeByHead(pH,Create_Node(15));
InsertNodeByTail(pH,Create_Node(6));
InsertNodeByTail(pH,Create_Node(5));
InsertNodeByTail(pH,Create_Node(90));
PrintNodeData(pH);
printf("--------------------------------------\n");
InsertNodeByAppointBefore(pH,3,Create_Node(100));
printf("--------------结点之前插入------------\n");
PrintNodeData(pH);
printf("--------------结点之后插入------------\n");
InsertNodeByAppointafter(pH,4,Create_Node(200));
PrintNodeData(pH);
printf("-----------------查找结点-------------\n");
ListNode p = FindNodeByData(pH,15);
printf("ret = %d 查找成功\n",p->data);
printf("-----------------删除结点-------------\n");
DeleteNodeByData(pH,5);
PrintNodeData(pH);
printf("%d 删除成功\n",5);
printf("-----------------排序结点-------------\n");
NodeBubble(pH);
PrintNodeData(pH);
return 0;
}
运行结果
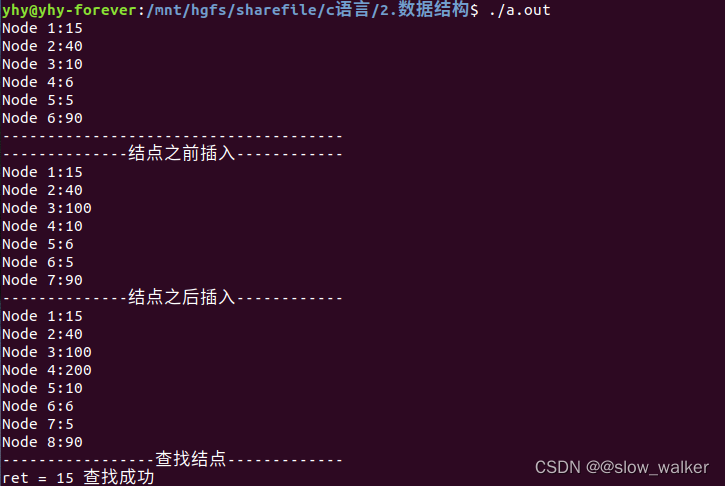
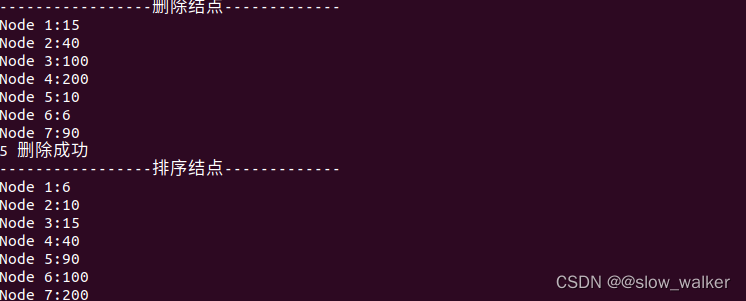