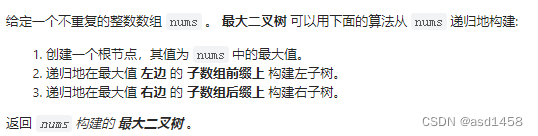
class Solution {
public TreeNode constructMaximumBinaryTree(int[] nums) {
return constructMax(nums,0,nums.length);
}
public TreeNode constructMax(int[] nums,int start,int end){
if(end-start==0){
return null;
}
if(end-start==1){
return new TreeNode(nums[start]);
}
int max = nums[start];
int index = start;
for(int i=start+1;i<end;i++){
if(max<nums[i]){
max = nums[i];
index = i;
}
}
TreeNode root = new TreeNode(max);
root.left = constructMax(nums,start,index);
root.right = constructMax(nums,index+1,end);
return root;
}
}
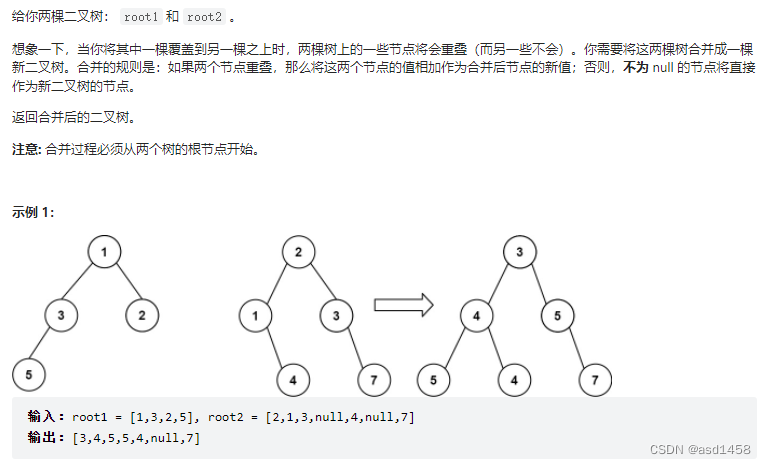
//递归
class Solution {
public TreeNode mergeTrees(TreeNode root1, TreeNode root2) {
if(root1==null&&root2==null){
return null;
}else if(root1==null&&root2!=null){
return root2;
}else if(root1!=null&&root2==null){
return root1;
}
TreeNode root = new TreeNode(root1.val+root2.val);
root.left = mergeTrees(root1.left,root2.left);
root.right = mergeTrees(root1.right,root2.right);
return root;
}
}
########################################################
//栈迭代
class Solution {
public TreeNode mergeTrees(TreeNode root1, TreeNode root2) {
Stack<TreeNode> stack = new Stack<>();
if(root1==null&&root2==null){
return null;
}
if(root1!=null&&root2==null){
return root1;
}
if(root1==null&&root2!=null){
return root2;
}
stack.push(root2);
stack.push(root1);
while(!stack.isEmpty()){
TreeNode cur1 = stack.pop();
TreeNode cur2 = stack.pop();
cur1.val = cur1.val+cur2.val;
if(cur1.left!=null&&cur2.left!=null){
stack.push(cur2.left);
stack.push(cur1.left);
}
if(cur1.right!=null&&cur2.right!=null){
stack.push(cur2.right);
stack.push(cur1.right);
}
if(cur1.left==null&&cur2.left!=null){
cur1.left = cur2.left;
}
if(cur1.right==null&&cur2.right!=null){
cur1.right = cur2.right;
}
}
return root1;
}
}
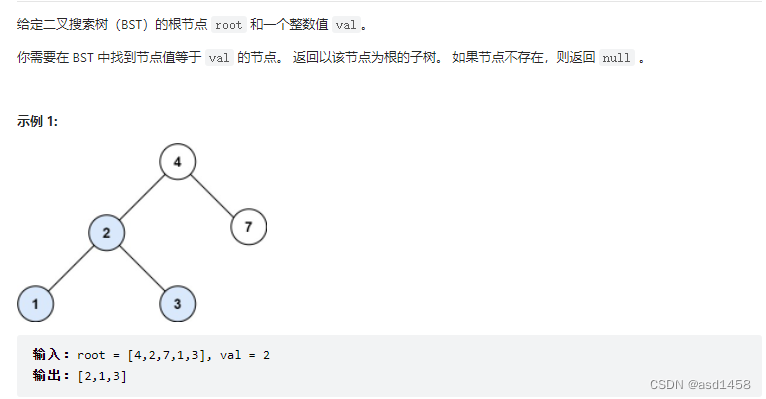
//递归
class Solution {
public TreeNode searchBST(TreeNode root, int val) {
if(root==null){
return null;
}
if(root.val==val){
return root;
}else if(root.val>val){
return searchBST(root.left,val);
}else{
return searchBST(root.right,val);
}
}
}
###########################
//迭代
class Solution {
public TreeNode searchBST(TreeNode root, int val) {
if(root==null){
return null;
}
Stack<TreeNode> stack = new Stack<>();
stack.push(root);
while(!stack.isEmpty()){
TreeNode cur = stack.pop();
if(cur.val==val){
return cur;
}else if(cur.val>val){
if(cur.left!=null){
stack.push(cur.left);
}
}else{
if(cur.right!=null){
stack.push(cur.right);
}
}
}
return null;
}
}
#递归
class Solution {
TreeNode max;
public boolean isValidBST(TreeNode root) {
if(root==null){
return true;
}
boolean left = isValidBST(root.left);
if(!left){
return false;
}
if(max!=null&&root.val<=max.val){
return false;
}
max = root;
boolean right = isValidBST(root.right);
return right;
}
}
#######################
class Solution {
public boolean isValidBST(TreeNode root) {
if(root==null){
return true;
}
double max = -Double.MAX_VALUE;
Stack<TreeNode> stack = new Stack<>();
while(root!=null||!stack.isEmpty()){
while(root!=null){
stack.push(root);
root = root.left;
}
root = stack.pop();
if(root.val<=max){
return false;
}
max = root.val;
root = root.right;
}
return true;
}
}
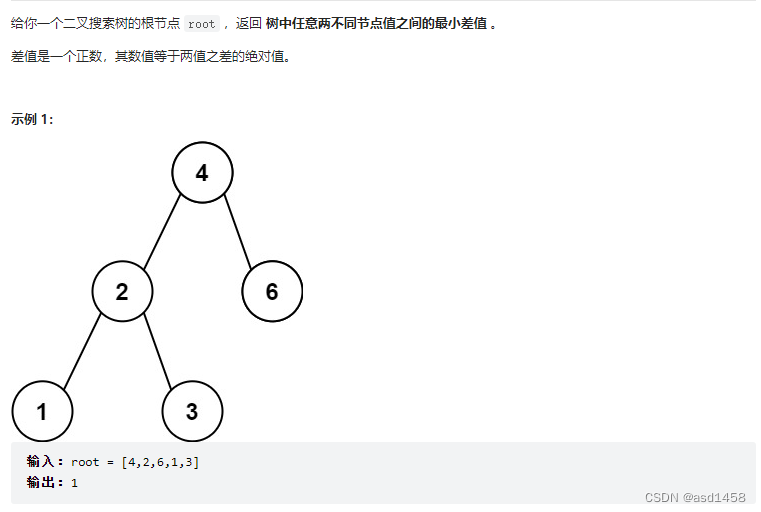
class Solution {
TreeNode pre;
int res = Integer.MAX_VALUE;
public int getMinimumDifference(TreeNode root) {
if(root==null){
return 0;
}
traversal(root);
return res;
}
public void traversal(TreeNode root){
if(root==null){
return;
}
traversal(root.left);
if(pre!=null){
res = Math.min(res,root.val-pre.val);
}
pre = root;
traversal(root.right);
}
}
#########################################
class Solution {
TreeNode pre=null;
int res = Integer.MAX_VALUE;
public int getMinimumDifference(TreeNode root) {
if(root==null){
return 0;
}
Stack<TreeNode> stack = new Stack<>();
while(root!=null||!stack.isEmpty()){
while(root!=null){
stack.push(root);
root = root.left;
}
root = stack.pop();
if(pre!=null){
res = Math.min(root.val-pre.val,res);
}
pre = root;
root = root.right;
}
return res;
}
}