1、定义线程间交互数据
#ifndef MESSAGE_H
#define MESSAGE_H
#include <QMetaType>
#include <QVariant>
struct Message{
QVariant data;
};
Q_DECLARE_METATYPE(Message)
#endif
2、 业务类
#ifndef BUSINESS_H
#define BUSINESS_H
#include <QObject>
#include "Message.h"
class Business : public QObject
{
Q_OBJECT
public:
explicit Business(QObject *parent = nullptr);
public slots:
int onAdd();
Message onSub(Message in);
};
#endif
#include "business.h"
#include <QTimer>
#include <QDebug>
#include <QThread>
Business::Business(QObject *parent) : QObject(parent)
{
QTimer *t = new QTimer(this);
connect(t,&QTimer::timeout,this,[](){
qDebug()<<"timer:"<<QThread::currentThread();
});
t->start(10000);
}
int Business::onAdd()
{
static int i = 0;
qDebug()<<Q_FUNC_INFO<<QThread::currentThread();
return i++;
}
Message Business::onSub(Message in)
{
qDebug()<<Q_FUNC_INFO<<QThread::currentThread();
Message ret ;
ret.data = QVariant::fromValue(in.data.toInt()-1);
return ret ;
}
3、GUI线程
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_pushButton_clicked();
void on_pushButton_2_clicked();
signals:
int getVal();
private:
QObject * b ;
private:
Ui::MainWindow *ui;
};
#endif
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "business.h"
#include <QThread>
#include <QDebug>
#include "Message.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
b = new Business;
connect(this,SIGNAL(getVal()),b,SLOT(onAdd()),Qt::DirectConnection);
QThread *work = new QThread;
b->moveToThread(work);
work->start();
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_pushButton_clicked()
{
qDebug()<< thread();
qDebug()<<"add:"<<getVal();
}
void MainWindow::on_pushButton_2_clicked()
{
static int t = 10000;
Message in;
in.data = QVariant::fromValue(t);
Message ret;
qDebug()<< b->thread();
qDebug()<< thread();
if(b->thread() != thread())
{
QMetaObject::invokeMethod(b, "onSub", Qt::BlockingQueuedConnection,
Q_RETURN_ARG(Message,ret ),
Q_ARG(Message, in));
qDebug() <<"sub:"<<ret.data.toInt();
t = ret.data.toInt();
}
}
4、效果
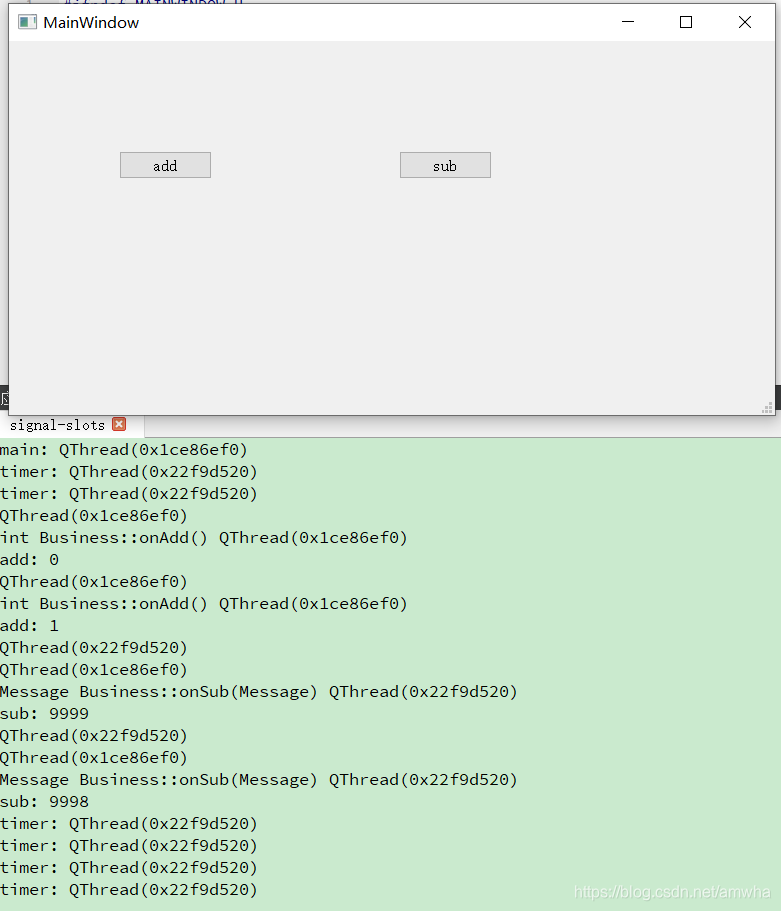